1.10 Unit Test Triangle Similarity - Part 1
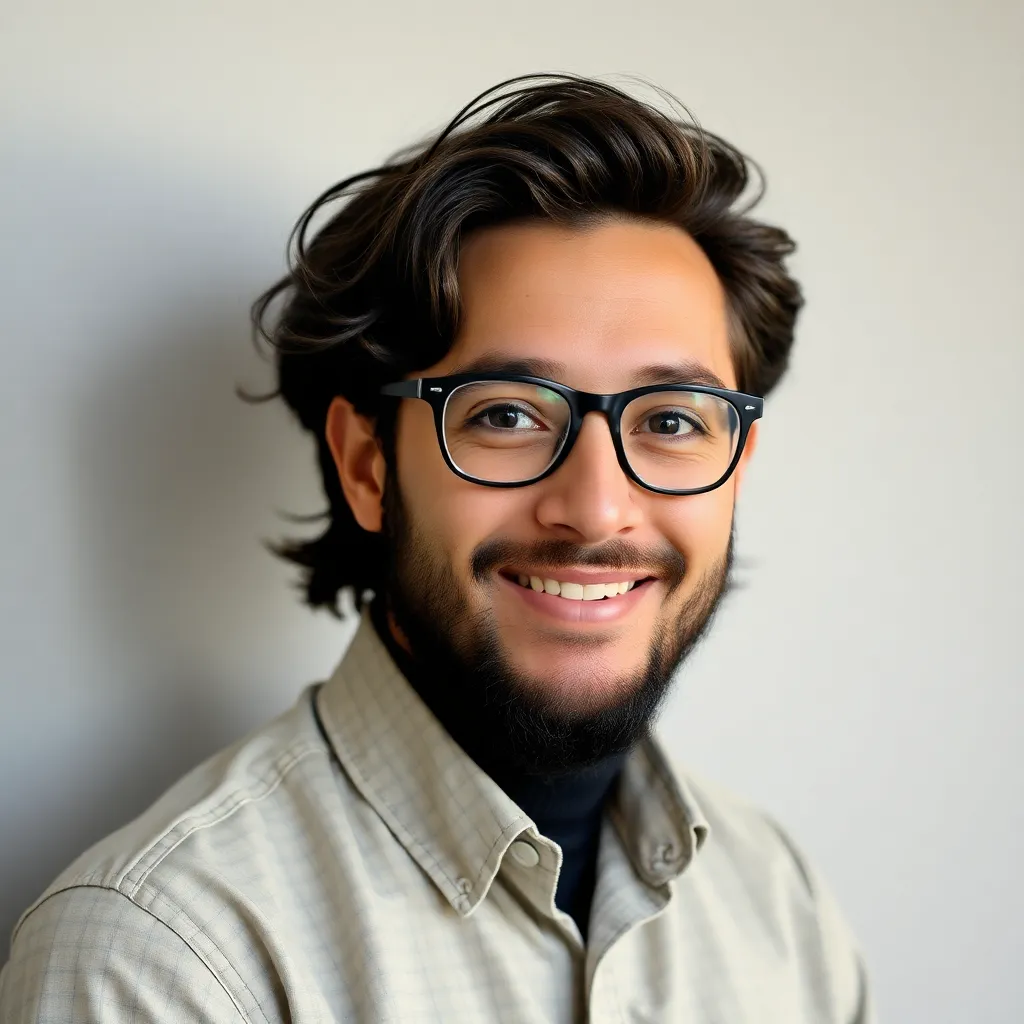
Onlines
Apr 12, 2025 · 5 min read

Table of Contents
1.10 Unit Test Triangle Similarity - Part 1: Laying the Foundation for Robust Geometric Calculations
Testing is a cornerstone of robust software development, and this principle extends significantly to geometric calculations, particularly those involving triangle similarity. This article focuses on unit testing strategies for algorithms determining triangle similarity, specifically covering the foundational aspects in Part 1. We'll explore various approaches, examining their strengths and weaknesses, and emphasizing best practices for writing effective and comprehensive tests. Part 2 will delve deeper into advanced testing techniques and edge cases.
Understanding Triangle Similarity
Before we dive into the testing process, it's crucial to understand the core concept: triangle similarity. Two triangles are considered similar if their corresponding angles are congruent (equal) and their corresponding sides are proportional. This means one triangle is essentially a scaled version of the other. There are several criteria to establish similarity:
-
AA (Angle-Angle): If two angles of one triangle are congruent to two angles of another triangle, the triangles are similar.
-
SSS (Side-Side-Side): If the three sides of one triangle are proportional to the three sides of another triangle, the triangles are similar.
-
SAS (Side-Angle-Side): If two sides of one triangle are proportional to two sides of another triangle and the included angles are congruent, the triangles are similar.
Setting up the Testing Environment
Choosing the right testing framework is critical. Popular choices include:
- JUnit (Java): A widely-used unit testing framework for Java.
- pytest (Python): A flexible and powerful testing framework for Python.
- NUnit (.NET): A unit testing framework for .NET languages like C#.
- Jest (JavaScript): A JavaScript testing framework particularly popular with React and other JavaScript projects.
Regardless of the framework you choose, the fundamental principles remain the same: isolate units of code (in this case, functions or methods related to triangle similarity), provide various inputs, and assert the expected outputs.
Test Cases for AA Similarity
Let's start with the AA similarity criterion. We'll need to design test cases covering various scenarios:
Positive Test Cases (Expecting Similarity):
-
Case 1: Identical Angles: Two triangles with identical angles (e.g., 60°, 60°, 60° and 60°, 60°, 60°). This is a basic, straightforward test.
-
Case 2: Different Angle Values: Two triangles with different but proportional angles (e.g., 30°, 60°, 90° and 30°, 60°, 90°). This tests whether the algorithm handles different angle combinations correctly.
-
Case 3: Angles in a Different Order: Two triangles with the same angles but listed in a different order (e.g., 30°, 60°, 90° and 90°, 30°, 60°). This checks robustness against variations in input order.
Negative Test Cases (Expecting No Similarity):
-
Case 4: One Angle Different: Two triangles where only one pair of angles is congruent (e.g., 30°, 60°, 90° and 30°, 70°, 80°).
-
Case 5: No Congruent Angles: Two triangles with completely different angles (e.g., 30°, 60°, 90° and 45°, 45°, 90°).
Example using Python and pytest:
import pytest
def is_similar_aa(triangle1, triangle2):
"""Checks similarity using the AA criterion."""
# Implementation of the AA similarity algorithm (omitted for brevity)
pass
def test_aa_similarity_identical():
assert is_similar_aa([60, 60, 60], [60, 60, 60]) == True
def test_aa_similarity_different_angles():
assert is_similar_aa([30, 60, 90], [30, 60, 90]) == True
def test_aa_similarity_different_order():
assert is_similar_aa([30, 60, 90], [90, 30, 60]) == True
def test_aa_similarity_one_angle_different():
assert is_similar_aa([30, 60, 90], [30, 70, 80]) == False
def test_aa_similarity_no_congruent_angles():
assert is_similar_aa([30, 60, 90], [45, 45, 90]) == False
Test Cases for SSS Similarity
The SSS similarity criterion requires checking the proportionality of sides.
Positive Test Cases (Expecting Similarity):
- Case 1: Identical Sides: Two triangles with identical sides.
- Case 2: Proportional Sides: Two triangles where the sides are proportional (e.g., sides 3, 4, 5 and 6, 8, 10).
- Case 3: Sides in Different Order: Two triangles with proportional sides but listed in a different order.
Negative Test Cases (Expecting No Similarity):
- Case 4: One Side Disproportional: Two triangles where only two sides are proportional.
- Case 5: No Proportional Sides: Two triangles with completely different side lengths.
Example incorporating error handling:
import pytest
def is_similar_sss(triangle1, triangle2):
"""Checks similarity using the SSS criterion."""
#Implementation with error handling for cases like empty lists or non-numeric values.
if not all(isinstance(x, (int, float)) for x in triangle1 + triangle2):
raise ValueError("Triangle sides must be numeric")
if not triangle1 or not triangle2:
raise ValueError("Triangles cannot be empty")
#Check for proportionality (omitted for brevity)
pass
def test_sss_similarity_proportional():
assert is_similar_sss([3, 4, 5], [6, 8, 10]) == True
def test_sss_similarity_error_handling_empty():
with pytest.raises(ValueError):
is_similar_sss([], [1,2,3])
def test_sss_similarity_error_handling_non_numeric():
with pytest.raises(ValueError):
is_similar_sss([1,"a",3],[1,2,3])
Test Cases for SAS Similarity
This criterion involves checking both side proportionality and the congruence of the included angle.
Positive Test Cases (Expecting Similarity):
- Case 1: Identical Sides and Angle: Two triangles with identical sides and included angle.
- Case 2: Proportional Sides and Congruent Angle: Two triangles with proportional sides and the same included angle.
Negative Test Cases (Expecting No Similarity):
- Case 3: Proportional Sides, Different Angle: Two triangles with proportional sides but different included angles.
- Case 4: One Side Disproportional, Congruent Angle: Two triangles where only one pair of sides is proportional, but the included angle is the same.
- Case 5: No Proportional Sides: Two triangles with non-proportional sides, even if the included angle is congruent.
Advanced Testing Techniques (Preview for Part 2)
Part 2 will cover more advanced aspects, including:
- Boundary Value Analysis: Testing values at the boundaries of valid input ranges (e.g., very small or very large side lengths).
- Equivalence Partitioning: Dividing input values into groups that are expected to behave similarly.
- Test-Driven Development (TDD): Writing tests before writing the code.
- Code Coverage Analysis: Measuring how much of the code is exercised by the tests.
- Integration Testing: Testing the interaction between multiple modules.
- Handling Invalid Inputs: Thoroughly testing responses to unexpected or invalid input such as negative side lengths or angles greater than 180 degrees.
Conclusion: Building a Strong Foundation
This first part lays the groundwork for comprehensive unit testing of triangle similarity algorithms. By systematically creating positive and negative test cases for each similarity criterion (AA, SSS, SAS), we've established a strong foundation for building reliable and robust geometric calculation software. Part 2 will expand on these concepts, introducing more sophisticated techniques and edge cases to further enhance the quality and reliability of your code. Remember, thorough testing is crucial for preventing unexpected errors and ensuring the accuracy of your geometric applications.
Latest Posts
Latest Posts
-
Which Of The Following Is True Of A Floating Graphic
Apr 13, 2025
-
Which Of The Following Is Not A Principle Of Puritanism
Apr 13, 2025
-
West Africa Showed A High Degree Of Regional Complementarity Answer
Apr 13, 2025
-
Hartman Nursing Assistant Care Workbook Answers
Apr 13, 2025
-
Biology Eoc Review Packet Answer Key Pdf
Apr 13, 2025
Related Post
Thank you for visiting our website which covers about 1.10 Unit Test Triangle Similarity - Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.