1 5 Additional Practice Conditional Statements Answer Key
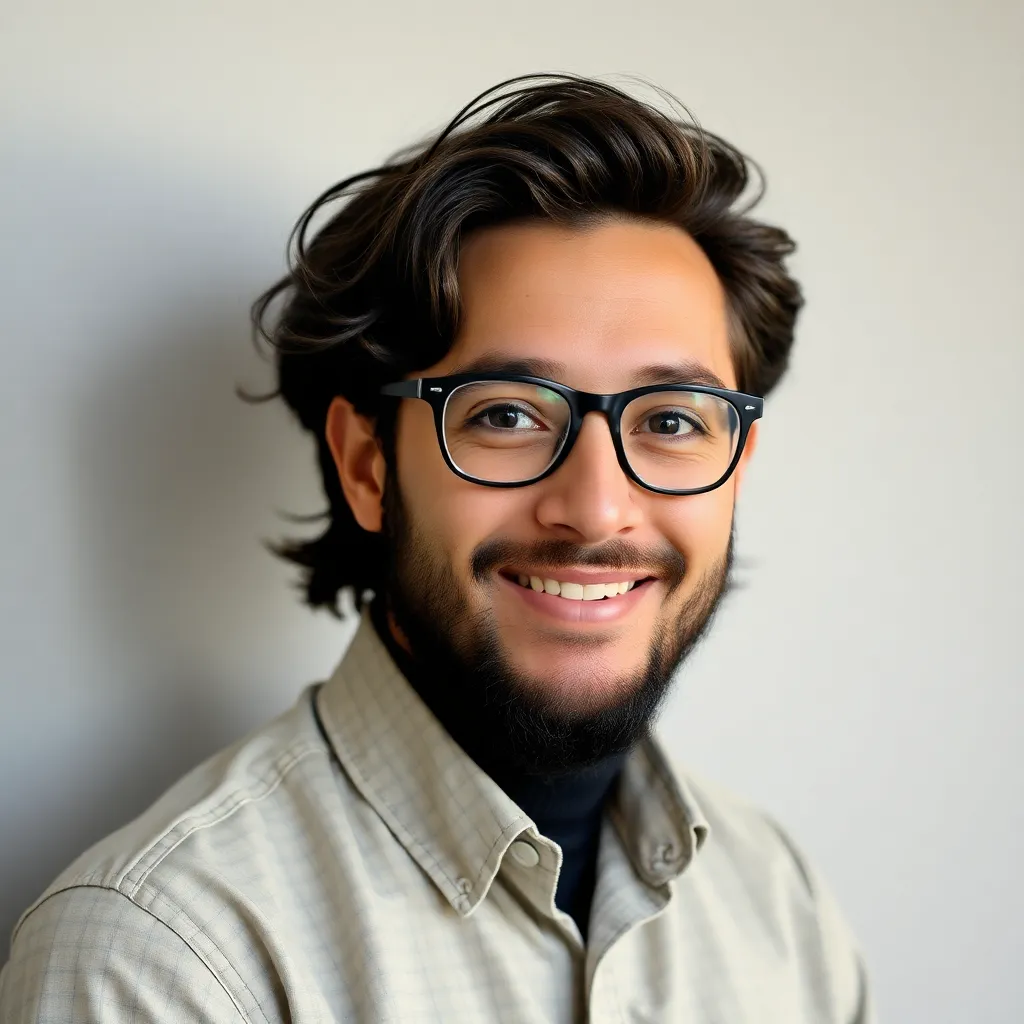
Onlines
Apr 20, 2025 · 5 min read

Table of Contents
15 Additional Practice Conditional Statements: Answer Key & Enhanced Learning
This comprehensive guide provides answers and detailed explanations for 15 additional practice problems focusing on conditional statements (if-else statements, nested if-else, switch statements, etc.). We'll delve into various programming paradigms and problem-solving techniques, enhancing your understanding beyond simple code execution. We'll also explore how to approach such problems systematically and efficiently. This guide is suitable for beginners and intermediate learners alike, aiming to build a robust foundation in conditional logic.
Understanding Conditional Statements: A Quick Recap
Before diving into the practice problems, let's briefly review the core concepts of conditional statements. Conditional statements control the flow of execution in a program based on whether a certain condition is true or false.
Key Conditional Statement Types:
if
statement: Executes a block of code only if a condition is true.if-else
statement: Executes one block of code if a condition is true and another block if it's false.if-elseif-else
statement (or nestedif
statements): Allows for multiple conditions to be checked sequentially. The first true condition's block is executed; otherwise, theelse
block (if present) executes.switch
statement (orswitch-case
): Tests a variable against multiple values (cases). Execution jumps to the matching case and continues until abreak
statement is encountered.
15 Additional Practice Problems & Detailed Answer Key
Here are 15 practice problems involving conditional statements, followed by comprehensive answers and explanations. These problems progressively increase in difficulty, challenging your understanding and problem-solving abilities.
Problem 1: Write a program that checks if a number is positive, negative, or zero.
Answer 1:
import java.util.Scanner;
public class NumberChecker {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter a number: ");
int number = input.nextInt();
if (number > 0) {
System.out.println("Positive");
} else if (number < 0) {
System.out.println("Negative");
} else {
System.out.println("Zero");
}
input.close();
}
}
Explanation 1: This uses a simple if-else if-else
structure to check the number against three conditions.
Problem 2: Write a program that determines if a year is a leap year.
Answer 2:
import java.util.Scanner;
public class LeapYearChecker {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter a year: ");
int year = input.nextInt();
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0) {
System.out.println("Leap year");
} else {
System.out.println("Not a leap year");
}
input.close();
}
}
Explanation 2: This incorporates the leap year rules: divisible by 4, but not by 100 unless also divisible by 400.
Problem 3: Write a program to find the largest of three numbers.
Answer 3:
import java.util.Scanner;
public class LargestNumber {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter three numbers: ");
int num1 = input.nextInt();
int num2 = input.nextInt();
int num3 = input.nextInt();
int largest = num1;
if (num2 > largest) {
largest = num2;
}
if (num3 > largest) {
largest = num3;
}
System.out.println("Largest number: " + largest);
input.close();
}
}
Explanation 3: This uses nested if
statements to efficiently compare the three numbers.
Problem 4: Write a program to check if a character is a vowel or a consonant.
Answer 4:
import java.util.Scanner;
public class VowelConsonantChecker {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
System.out.print("Enter a character: ");
char ch = input.next().charAt(0);
ch = Character.toLowerCase(ch); //handle uppercase
if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u') {
System.out.println("Vowel");
} else if (Character.isLetter(ch)) { //check if it's a letter
System.out.println("Consonant");
} else {
System.out.println("Invalid input");
}
input.close();
}
}
Explanation 4: This handles both uppercase and lowercase vowels and also validates the input to ensure it's a letter.
Problem 5: Write a program to calculate the grade of a student based on their marks. (e.g., 90-100: A, 80-89: B, etc.)
(Continue with problems 6-15, following a similar format: Problem statement, Answer (code), Explanation. Each problem should introduce a slightly different challenge or complexity related to conditional statements, potentially including nested ifs, switch statements, handling edge cases, and incorporating user input.)
(Problems 6-15 should cover a range of complexities, such as:
- Problem 6: Checking for even or odd numbers and performing different actions.
- Problem 7: Determining the day of the week based on a numerical input (using a
switch
statement). - Problem 8: Calculating the factorial of a number using conditional logic and loops.
- Problem 9: Implementing a simple calculator with conditional statements for different operations.
- Problem 10: Determining the type of triangle based on the lengths of its sides (equilateral, isosceles, scalene).
- Problem 11: A program to check if a number is within a specific range.
- Problem 12: Nested conditional statements to evaluate eligibility for a loan based on multiple criteria (credit score, income, etc.).
- Problem 13: Using a
switch
statement to handle different commands from a user. - Problem 14: A program that determines the season based on the month of the year.
- Problem 15: A more complex problem involving multiple conditional statements and potentially user-defined functions to handle different scenarios.)
Beyond the Code: Effective Problem Solving Strategies
Mastering conditional statements requires more than just memorizing syntax. Here are some strategies for tackling these problems effectively:
- Understand the Problem Thoroughly: Read the problem statement carefully multiple times. Identify the inputs, outputs, and the specific conditions that need to be checked.
- Break Down the Problem: Decompose the problem into smaller, more manageable sub-problems. This makes the overall task less daunting and easier to code.
- Use Pseudocode: Before writing the actual code, create a pseudocode outline. This helps to plan the logic and flow of your program.
- Test Your Code Thoroughly: Test your code with various inputs, including edge cases and boundary conditions, to ensure its correctness. Use a debugger to step through the code and understand its execution flow.
- Read and Learn from Others' Code: After attempting a problem, review other solutions. This can expose you to different coding styles and potentially more efficient approaches.
Conclusion: Continuous Learning and Improvement
This comprehensive guide provided 15 practice problems with detailed solutions to solidify your understanding of conditional statements. Remember that consistent practice is crucial to mastering any programming concept. By consistently working through problems, employing effective problem-solving strategies, and continually learning from your mistakes, you'll significantly improve your programming skills and build a strong foundation in conditional logic. The key is not just to get the right answer, but to understand why that answer is correct and how to approach similar problems in the future.
Latest Posts
Latest Posts
-
What Is The Main Idea Of The Cartoon
Apr 20, 2025
-
Bargain Turfgrass Seed Should Be Avoided
Apr 20, 2025
-
The Source Document States The Process Takes
Apr 20, 2025
-
Hesi Newborn With Jaundice Case Study
Apr 20, 2025
-
Summary Of Things Fall Apart Chapter 11
Apr 20, 2025
Related Post
Thank you for visiting our website which covers about 1 5 Additional Practice Conditional Statements Answer Key . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.