2.12 Unit Test Development Of Theme - Part 1
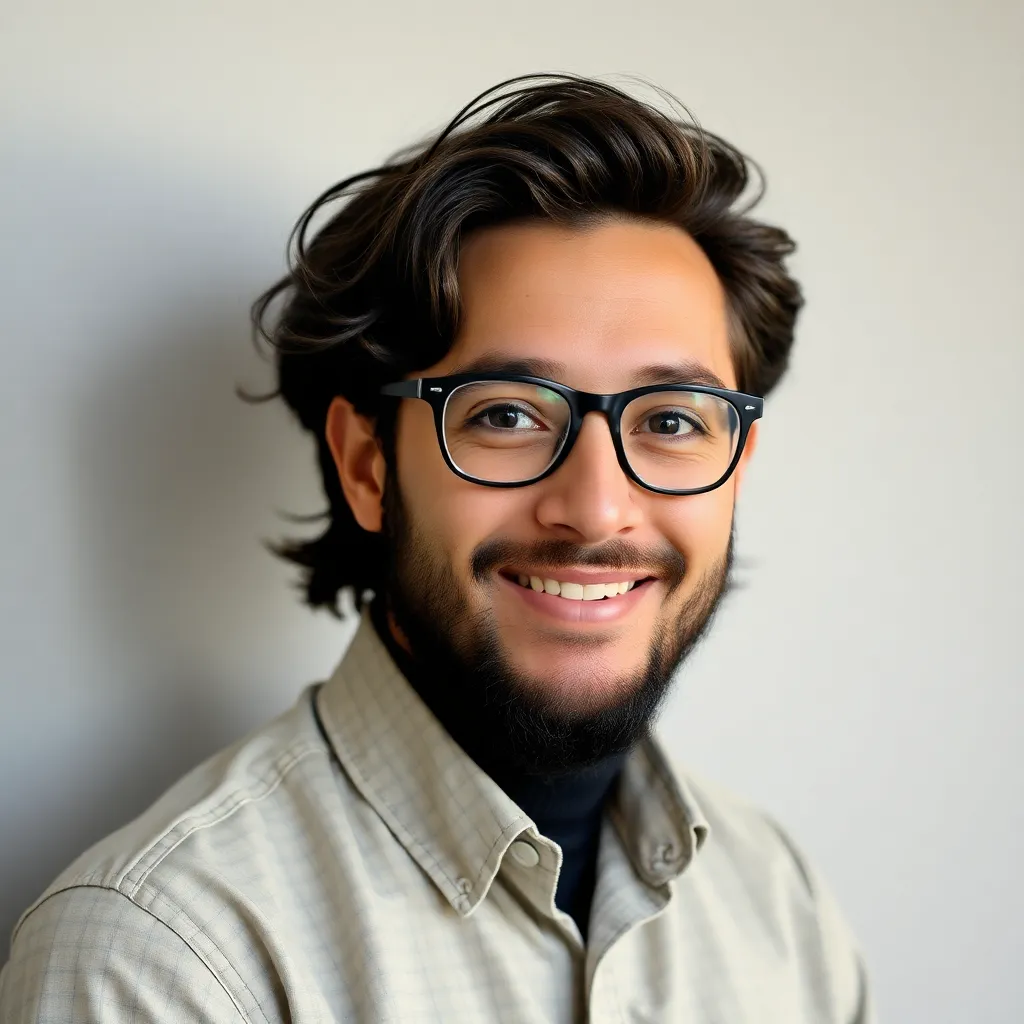
Onlines
May 08, 2025 · 7 min read
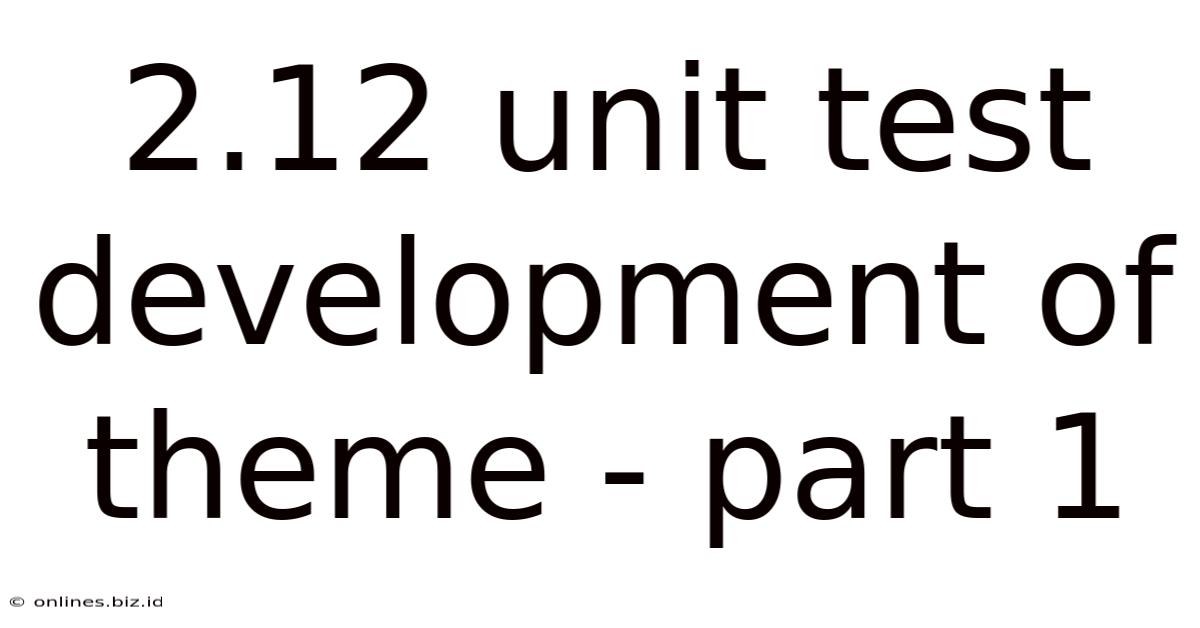
Table of Contents
2.12 Unit Test Development of Theme - Part 1: Laying the Foundation for Robust WordPress Themes
Developing robust and reliable WordPress themes requires a rigorous testing strategy. While visual inspection and manual testing are crucial, they are insufficient to guarantee a high-quality, bug-free theme. This is where unit testing comes into play. Unit testing, a cornerstone of software development best practices, allows developers to isolate individual components (units) of their code and verify their functionality in a controlled environment. This comprehensive guide will delve into the intricacies of unit testing for WordPress themes, specifically focusing on version 2.12, and will lay the groundwork for building a robust and maintainable theme.
Understanding the Importance of Unit Testing in Theme Development
Before diving into the specifics of 2.12 unit test development, it's crucial to understand why unit testing is essential for WordPress theme development. The benefits are numerous and impactful:
1. Early Bug Detection:
Catching bugs early in the development lifecycle is significantly cheaper and less time-consuming than fixing them later. Unit tests identify issues before they propagate through the entire theme, saving valuable development time and preventing potential headaches down the line.
2. Improved Code Quality:
The act of writing unit tests forces developers to write cleaner, more modular, and better-documented code. This is because well-structured code is inherently easier to test.
3. Enhanced Maintainability:
A well-tested theme is easier to maintain and extend. When changes are made, the unit tests act as a safety net, ensuring that existing functionality remains intact. This is particularly critical as themes evolve and grow over time.
4. Increased Confidence:
Having a comprehensive suite of unit tests provides developers with increased confidence in their code. They can make changes and refactor with less fear of introducing regressions.
5. Simplified Debugging:
When a bug is discovered, unit tests help to quickly isolate the problematic code section. Instead of sifting through large amounts of code, developers can focus on the specific unit failing the test.
Setting up the Testing Environment for 2.12 Theme Development
Before we can begin writing unit tests, we need to set up the necessary environment. This typically involves:
1. Choosing a Testing Framework:
Several PHP testing frameworks are available, including PHPUnit, which is a popular and robust choice for WordPress development. PHPUnit provides a structured approach to writing and running tests, along with various assertion methods to verify expected outcomes.
2. Setting up PHPUnit:
Installing PHPUnit usually involves using Composer, a dependency manager for PHP. You'll need to add PHPUnit as a development dependency to your project's composer.json
file.
{
"require-dev": {
"phpunit/phpunit": "^9.5"
}
}
After adding this, run composer install
in your terminal within your theme's directory to install PHPUnit.
3. Creating Test Files:
Organize your test files in a structured manner. A common convention is to create a tests
directory within your theme's directory. Inside this directory, you'll create individual PHP files for each unit you want to test. For example, you might have files like tests/functions.php
or tests/class-my-custom-class.php
.
4. Writing Your First Test:
A simple PHPUnit test typically looks like this:
assertEquals(2, 1 + 1);
}
}
This simple test verifies that 1 + 1 equals 2. The assertEquals()
method is one of many assertion methods provided by PHPUnit.
Unit Testing Specific Components of a 2.12 Theme
Now, let's dive into testing specific aspects of a WordPress theme within the context of version 2.12. This will cover a variety of scenarios, highlighting different testing techniques.
1. Testing Theme Functions:
Many themes use custom functions to handle various tasks. These functions should be thoroughly tested. For example, let's say you have a function to sanitize user input:
// functions.php
function sanitize_my_input($input) {
return sanitize_text_field($input);
}
The corresponding test might look like this:
alert('XSS')";
$sanitizedInput = sanitize_my_input($unsanitizedInput);
$this->assertNotContains("