2.13 Lab - Create Movie Table
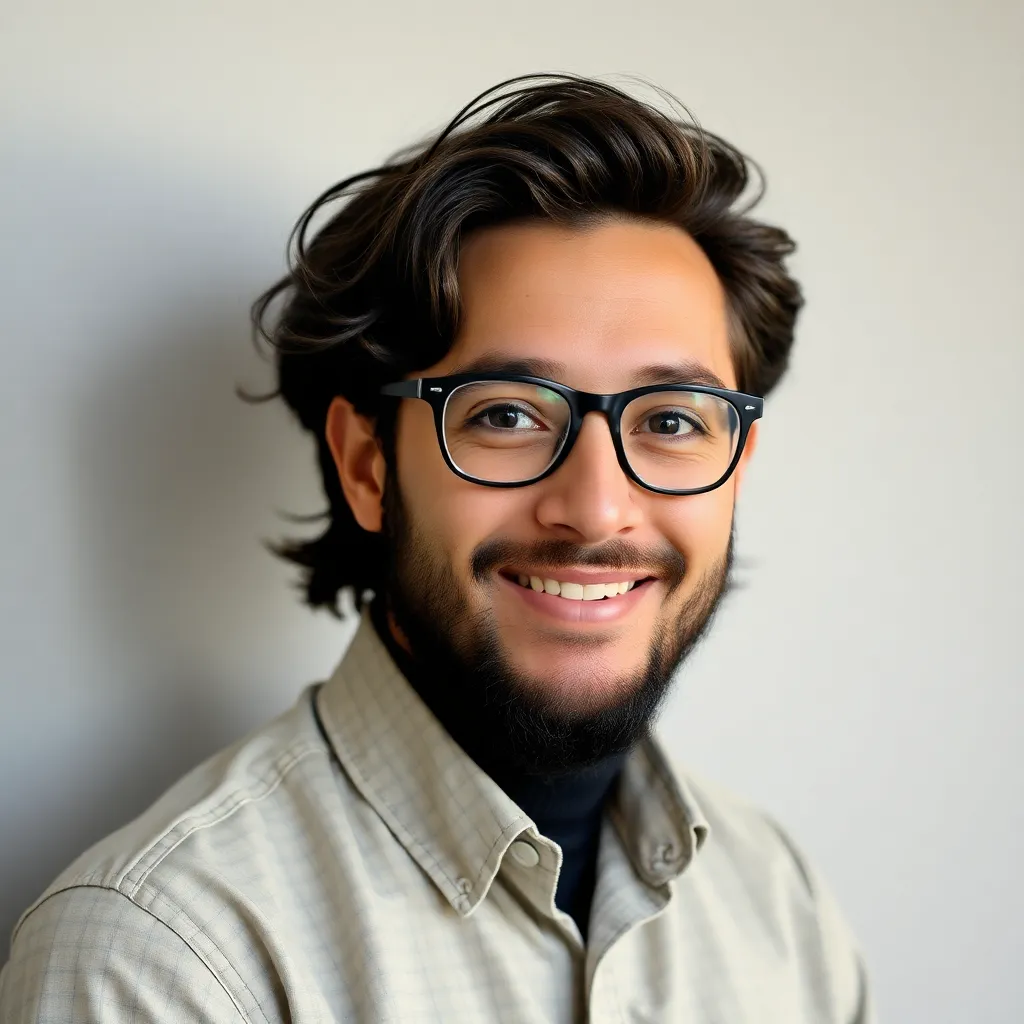
Onlines
Apr 24, 2025 · 5 min read

Table of Contents
2.13 Lab: Create Movie Table – A Deep Dive into Database Design and Implementation
This comprehensive guide delves into the intricacies of creating a "Movie" table within a database context, specifically focusing on the practical aspects of database design and implementation. We'll explore various aspects, from defining appropriate data types and constraints to optimizing for performance and scalability. This lab goes beyond simply creating the table; it teaches you how to build a robust and well-structured foundation for a larger movie database application.
Understanding Database Design Principles
Before diving into the SQL code, it's crucial to understand fundamental database design principles. These principles ensure data integrity, efficiency, and scalability. Key aspects include:
- Normalization: This process minimizes data redundancy and improves data integrity. We'll aim for at least the third normal form (3NF) in our design.
- Data Types: Choosing the correct data types is vital for both storage efficiency and data validation. We'll carefully select data types suitable for each attribute of our "Movie" table.
- Constraints: Constraints like
NOT NULL
,UNIQUE
,PRIMARY KEY
, andFOREIGN KEY
enforce data integrity and consistency. We'll implement relevant constraints to ensure data quality. - Indexing: Proper indexing significantly enhances query performance. We'll consider which columns benefit from indexing to speed up data retrieval.
Defining the Attributes of the Movie Table
Let's define the attributes (columns) for our Movie
table. A well-designed table anticipates future needs and allows for flexible data management. Here’s a proposed schema:
Attribute Name | Data Type | Constraints | Description |
---|---|---|---|
movie_id |
INT |
PRIMARY KEY , AUTO_INCREMENT |
Unique identifier for each movie. |
title |
VARCHAR(255) |
NOT NULL |
Title of the movie. |
release_year |
YEAR |
Year the movie was released. | |
director |
VARCHAR(255) |
Name of the director. | |
rating |
DECIMAL(2,1) |
Movie rating (e.g., 7.5). | |
genre |
VARCHAR(255) |
Genre of the movie (e.g., Action, Comedy, Drama). Could be normalized. | |
runtime_minutes |
INT |
Runtime of the movie in minutes. | |
plot_summary |
TEXT |
Brief summary of the movie's plot. | |
box_office_gross |
DECIMAL(15,2) |
Gross box office revenue (in USD). | |
production_company |
VARCHAR(255) |
Name of the production company. |
SQL Code for Creating the Movie Table
Now, let's translate the above schema into SQL code. The specific SQL dialect might vary slightly depending on your database system (MySQL, PostgreSQL, SQL Server, etc.), but the core concepts remain consistent.
CREATE TABLE Movie (
movie_id INT PRIMARY KEY AUTO_INCREMENT,
title VARCHAR(255) NOT NULL,
release_year YEAR,
director VARCHAR(255),
rating DECIMAL(2,1),
genre VARCHAR(255),
runtime_minutes INT,
plot_summary TEXT,
box_office_gross DECIMAL(15,2),
production_company VARCHAR(255)
);
This SQL statement creates the Movie
table with the specified attributes and constraints. The AUTO_INCREMENT
clause ensures that movie_id
values are automatically generated and unique.
Normalization Considerations: Genre Table
Notice that the genre
attribute is currently a single VARCHAR field. For a larger database, this can lead to redundancy and inconsistencies (e.g., "Action", "action", "ACTION"). A better approach would be to normalize the genre
data into a separate table.
Let's create a Genre
table:
CREATE TABLE Genre (
genre_id INT PRIMARY KEY AUTO_INCREMENT,
genre_name VARCHAR(255) UNIQUE NOT NULL
);
Now, we'll modify the Movie
table to include a foreign key referencing the Genre
table:
ALTER TABLE Movie
ADD COLUMN genre_id INT,
ADD CONSTRAINT fk_movie_genre FOREIGN KEY (genre_id) REFERENCES Genre(genre_id);
This refactoring eliminates redundancy and improves data integrity. Adding new genres only requires updating the Genre
table; no changes to the Movie
table are needed.
Indexing for Performance Optimization
To improve query performance, especially on large datasets, we should add indexes to frequently queried columns. For example, indexing the title
column will speed up searches based on movie titles.
CREATE INDEX idx_movie_title ON Movie (title);
Similarly, indexing release_year
and genre_id
could be beneficial depending on typical query patterns. The choice of which columns to index depends on your application's specific needs.
Populating the Movie Table with Data
After creating the table, you'll need to populate it with data. This can be done using SQL INSERT
statements:
INSERT INTO Movie (title, release_year, director, rating, genre_id, runtime_minutes, plot_summary, box_office_gross, production_company)
VALUES
('The Shawshank Redemption', 1994, 'Frank Darabont', 9.3, 1, 142, 'Two imprisoned men bond over a number of years, finding solace and eventual redemption through acts of common decency.', 28341469, 'Columbia Pictures'),
('The Godfather', 1972, 'Francis Ford Coppola', 9.2, 2, 175, 'The aging patriarch of an organized crime dynasty transfers control of his clandestine empire to his reluctant son.', 286000000, 'Paramount Pictures'),
('The Dark Knight', 2008, 'Christopher Nolan', 9.0, 3, 152, 'When the menace known as the Joker wreaks havoc and chaos on the city of Gotham, Batman must accept one of the greatest psychological and physical tests of his ability to fight injustice.', 1004934033, 'Warner Bros.');
Remember to insert data into the Genre
table first before adding movies referencing those genres.
Advanced Considerations
- Data Validation: Implement more robust data validation using triggers and constraints to prevent invalid data from being entered into the table.
- Data Security: Consider security measures to protect sensitive data, especially if your database contains user-provided information.
- Scalability: Design your database to handle increasing amounts of data efficiently as your movie collection grows. This might involve partitioning or sharding techniques for extremely large datasets.
- Transactions: Use transactions to ensure data consistency and prevent partial updates in case of errors.
Conclusion
Creating a well-designed and efficient database table, like the Movie
table in this lab, is a cornerstone of building robust and scalable applications. This guide has covered the fundamental aspects, from selecting appropriate data types and implementing constraints to optimizing for performance through indexing and normalization. Remember that effective database design is an iterative process. As your understanding grows and your application evolves, you may need to refine your schema and add new features. By understanding these principles and techniques, you’ll be well-equipped to design and manage your own database systems effectively. Keep practicing, experimenting, and learning to become proficient in database management.
Latest Posts
Related Post
Thank you for visiting our website which covers about 2.13 Lab - Create Movie Table . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.