2.13 Unit Test More Function Types
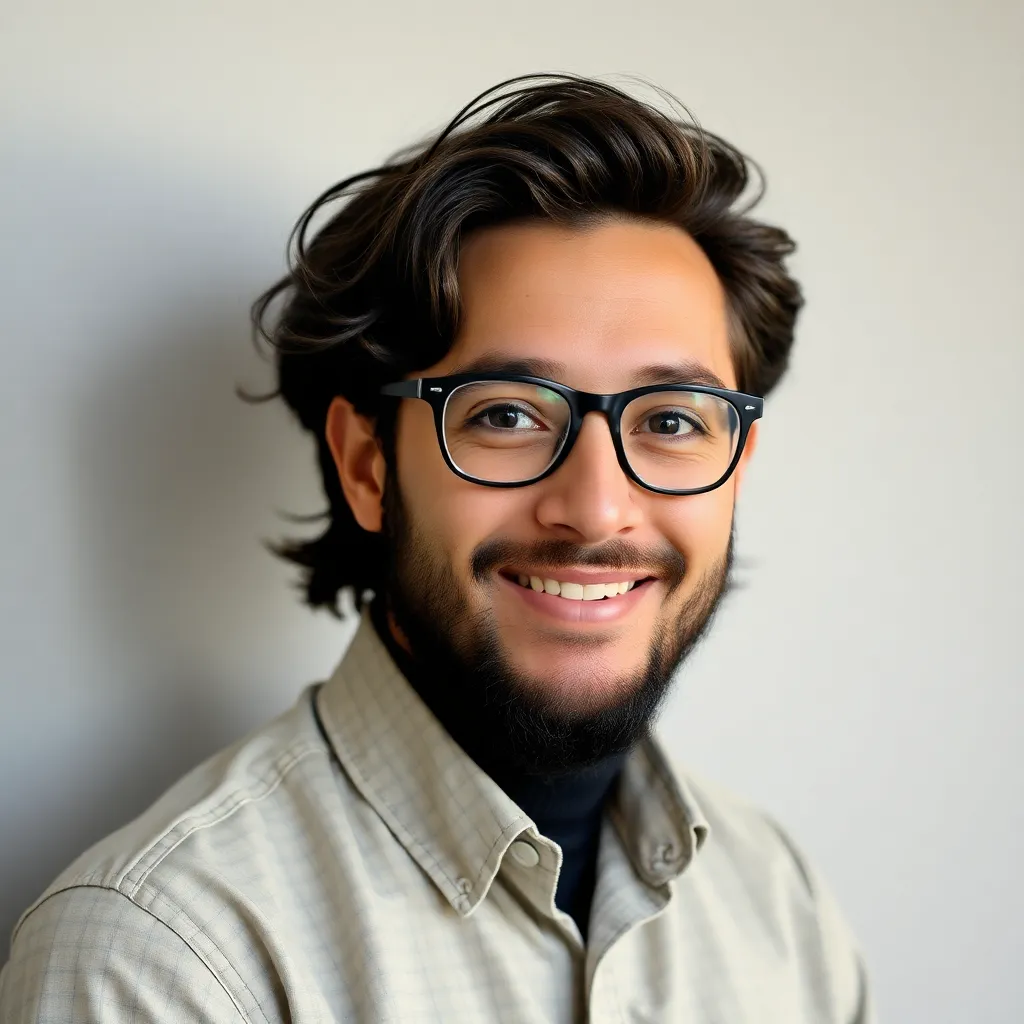
Onlines
Mar 31, 2025 · 6 min read
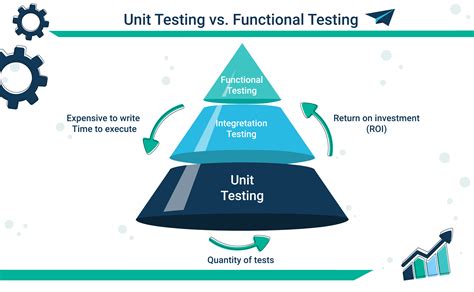
Table of Contents
2.13 Unit Test: Exploring More Function Types
Unit testing is a cornerstone of robust software development. It allows developers to verify the correctness of individual components (units) of code in isolation, ensuring that each part functions as expected before integration. While simple functions are relatively straightforward to test, the landscape broadens considerably when dealing with more complex function types. This article delves deeper into unit testing techniques for various function types, moving beyond the basics to cover scenarios involving functions with side effects, functions returning multiple values, functions utilizing mocking and patching, and asynchronous functions.
Understanding the Fundamentals of Unit Testing
Before diving into advanced function types, let's briefly revisit the core principles of unit testing. A good unit test should be:
- Independent: Each test should run independently without affecting others.
- Repeatable: The test should produce the same results every time it's run.
- Self-Validating: The test should automatically determine whether it passed or failed.
- Fast: Tests should execute quickly to facilitate rapid feedback during development.
We will primarily use a pseudo-code approach for demonstration purposes to ensure broad applicability across programming languages. However, concepts and principles will be universally applicable.
1. Unit Testing Functions with Side Effects
Functions with side effects modify something outside their own scope, such as modifying global variables, writing to files, or making network requests. These can make testing more challenging because the test needs to account for and manage these external interactions.
Example:
Let's consider a function that logs a message to a file:
function logMessageToFile(message, filename) {
// Opens the file, writes the message, and closes the file
openFile(filename)
writeFile(message)
closeFile(filename)
}
Testing this function directly would require managing file operations, which is not ideal for unit tests. The solution is to isolate the side effect using techniques like dependency injection and mocking.
Testing with Mocking:
Mocking involves replacing the dependency (the file system in this case) with a mock object that simulates its behavior.
testLogMessageToFile() {
mockFile = createMockFile() // Mock object simulating file operations
logMessageToFile("Test Message", mockFile)
assert(mockFile.contents == "Test Message") // Verify the message was written
}
This isolates the function's core logic from the external file system, making the test cleaner, faster, and more reliable.
2. Unit Testing Functions Returning Multiple Values
Many functions, particularly those performing complex computations, might return multiple values. Testing these functions requires handling each return value appropriately.
Example:
A function that calculates the area and perimeter of a rectangle:
function calculateRectangle(length, width) {
area = length * width
perimeter = 2 * (length + width)
return area, perimeter
}
Testing Multiple Return Values:
You can either unpack the returned values directly or access them by index if your language allows.
testCalculateRectangle() {
area, perimeter = calculateRectangle(5, 10)
assert(area == 50)
assert(perimeter == 30)
}
3. Unit Testing Functions with Complex Data Structures
Functions that operate on complex data structures, such as arrays, lists, dictionaries, or custom objects, necessitate more thorough testing. This involves verifying not only the output's correctness but also the integrity of the data structure itself.
Example:
A function that sorts an array of numbers:
function sortNumbers(numbers) {
// Sorting algorithm (e.g., bubble sort, merge sort)
return sortedNumbers
}
Testing Complex Data Structures:
Ensure the function handles various edge cases, including empty arrays, arrays with duplicates, and arrays with negative numbers. Use assertions to verify both the sorted order and the overall structure of the resulting array.
testSortNumbers() {
numbers = [5, 2, 8, 1, 9, 3]
sortedNumbers = sortNumbers(numbers)
assert(sortedNumbers == [1, 2, 3, 5, 8, 9])
assert(length(sortedNumbers) == length(numbers)) // Check array length
}
testSortNumbersEmptyArray() {
numbers = []
sortedNumbers = sortNumbers(numbers)
assert(length(sortedNumbers) == 0) // Handle empty array
}
4. Utilizing Mocking and Patching in Unit Tests
Mocking and patching are crucial for isolating units under test from their dependencies. Mocking replaces a dependency with a controlled substitute, while patching modifies the behavior of a specific function or method within a module.
Example:
Imagine a function that fetches data from a remote API:
function fetchDataFromAPI(url) {
response = makeAPIRequest(url)
// Process the response
return processedData
}
Testing with Mocking/Patching:
To prevent actual API calls during testing, you can mock the makeAPIRequest
function.
testFetchDataFromAPI() {
mockAPIRequest = function(url) { return {"data": "mock data"} }
patch(makeAPIRequest, mockAPIRequest) // Replace the original function
data = fetchDataFromAPI("someURL")
assert(data == "mock data")
unpatch(makeAPIRequest) // Restore the original function
}
5. Unit Testing Asynchronous Functions (Callbacks, Promises, Async/Await)
Asynchronous functions present unique challenges because their execution is not immediate. Testing requires handling asynchronous operations gracefully.
Example (using Promises):
function asyncFunction() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Async operation completed")
}, 100)
})
}
Testing Asynchronous Functions:
Use mechanisms provided by your testing framework to handle asynchronous operations. Common approaches include callbacks, promises, or async/await
.
testAsyncFunction() {
asyncFunction().then((result) => {
assert(result == "Async operation completed")
})
}
//Or using async/await (if supported by your testing framework and language):
testAsyncFunctionAsyncAwait() {
let result = await asyncFunction();
assert(result == "Async operation completed");
}
6. Testing Functions with Error Handling
Robust functions should gracefully handle potential errors. Testing should explicitly verify this error handling. This involves triggering error conditions and checking if the function responds appropriately.
Example:
function divide(numerator, denominator) {
if (denominator == 0) {
throw new Error("Cannot divide by zero")
}
return numerator / denominator
}
Testing Error Handling:
testDivideErrorHandling() {
try {
divide(10, 0)
assert(false, "Expected an error but none was thrown")
} catch (error) {
assert(error.message == "Cannot divide by zero")
}
}
7. Choosing the Right Assertion Library
The selection of an assertion library significantly impacts the effectiveness and readability of your unit tests. Many languages and frameworks offer dedicated assertion libraries providing various methods for comparing values, checking types, and verifying conditions. Consider the following factors:
- Readability: The assertion library should produce clear and concise error messages when tests fail.
- Features: The library should offer a range of assertions to suit diverse testing needs.
- Integration: Seamless integration with the testing framework is essential.
8. Best Practices for Unit Testing More Function Types
- Keep Tests Small and Focused: Each test should target a specific aspect of the function's behavior.
- Use Descriptive Test Names: The test name should clearly convey the purpose of the test.
- Prioritize Test Coverage: Strive for high test coverage, aiming to test all code paths and edge cases.
- Refactor Tests Regularly: Maintain clean, well-organized tests to improve readability and maintainability.
- Continuous Integration (CI): Integrate unit tests into a CI pipeline to automate testing and catch issues early in the development process.
Conclusion
Unit testing is an indispensable practice for creating high-quality, reliable software. While basic functions are relatively easy to test, handling more complex function types requires a deeper understanding of mocking, patching, asynchronous operations, error handling, and the appropriate usage of assertion libraries. By mastering these advanced techniques, developers can build robust, well-tested applications that are more resilient to defects and easier to maintain over time. Remember that effective unit testing isn't just about writing tests; it's about structuring your code in a testable way and establishing a culture of quality assurance from the outset of development.
Latest Posts
Latest Posts
-
Juror 8 Quotes With Page Numbers
Apr 01, 2025
-
What Does This Passage Imply About Cousin
Apr 01, 2025
-
Gramatica C Subject Pronouns And Ser Answer Key
Apr 01, 2025
-
I Look Into My Glass Reveals Elements Of Romanticism Through
Apr 01, 2025
-
Discrete Mathematics With Applications 5th Edition Solutions Pdf
Apr 01, 2025
Related Post
Thank you for visiting our website which covers about 2.13 Unit Test More Function Types . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.