3.11 Unit Test: Changing Expectations - Part 1
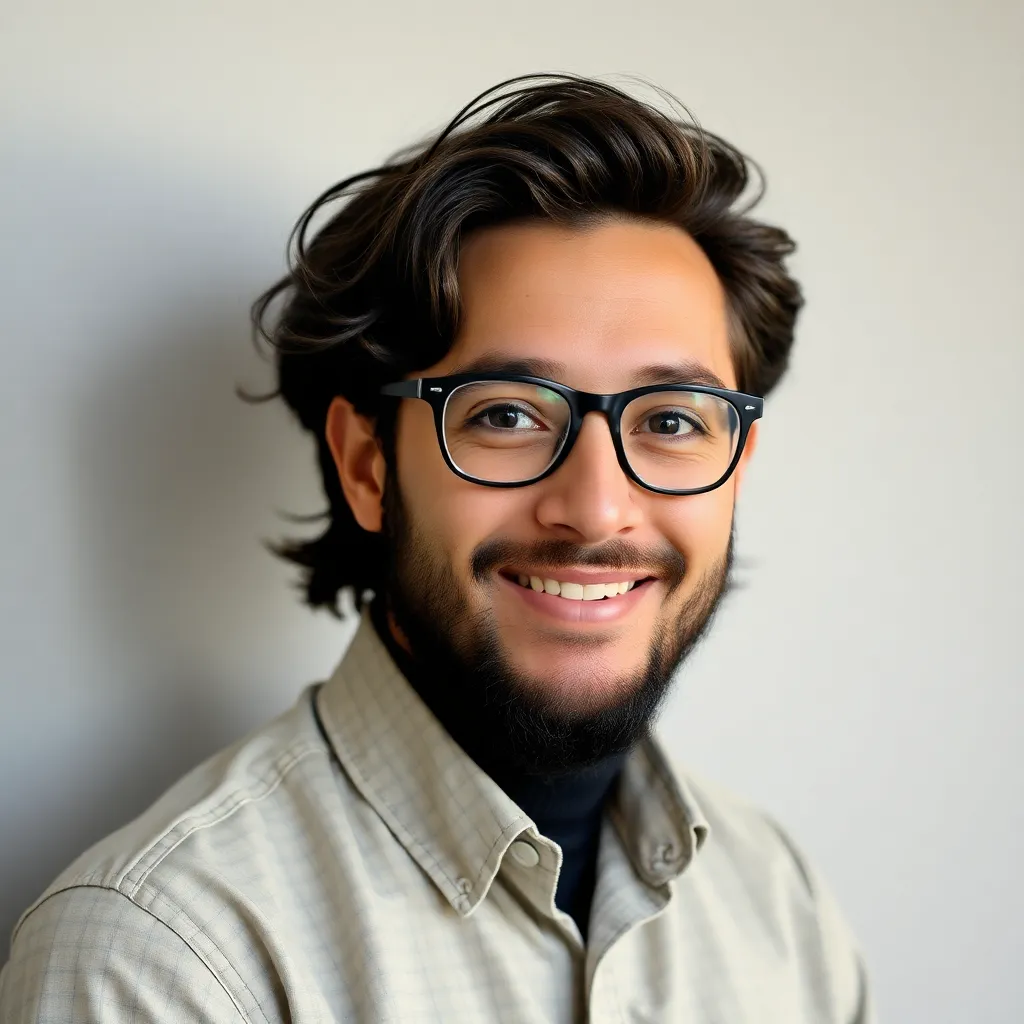
Onlines
Mar 29, 2025 · 6 min read
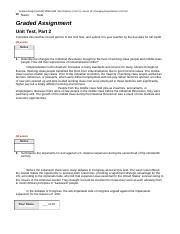
Table of Contents
3.11 Unit Test: Changing Expectations - Part 1
Unit testing is a cornerstone of robust software development. It allows developers to isolate individual components of their code and verify their behavior in a controlled environment. While writing effective unit tests is crucial, understanding how to adapt your tests as your code evolves is equally important. This is particularly true when dealing with changing expectations—when the functionality of a unit under test undergoes modification. This first part of a two-part series will delve into the nuances of managing evolving expectations in your unit tests, focusing on identifying when changes are needed and strategically modifying your tests to reflect the updated behavior.
Understanding the Need for Change
Before jumping into how to change your expectations, it's vital to understand when it's necessary. Changes in your codebase will inevitably necessitate adjustments to your unit tests. Ignoring these changes can lead to tests that no longer accurately reflect the reality of your application's behavior, rendering them ineffective and potentially misleading.
Here are some key scenarios that typically necessitate changes to your test expectations:
1. Bug Fixes and Improvements:
When you fix a bug, the expected output of the unit under test might change. Your test needs to be updated to reflect the corrected behavior. A failing test post-bug fix often indicates a problem with the test itself, not necessarily a regression.
2. Feature Additions and Modifications:
Adding new features or modifying existing ones directly impacts the functionality of your code. Unit tests must be adjusted to accommodate these changes. New tests might be added to cover the new functionality, and existing tests may require alterations to account for the updated behavior of the unit.
3. Refactoring:
Refactoring involves restructuring your code without changing its external behavior. While the implementation details change, the unit's functionality should remain the same. However, even refactoring might necessitate adjustments to your tests to match the refactored code's structure. This ensures the tests still effectively verify the unchanged behavior.
4. Dependency Updates:
Changes in external dependencies (libraries, APIs) can indirectly affect the behavior of your code. Your tests need to adapt to account for any resultant changes in your unit's output.
5. Performance Optimizations:
Performance optimization might subtly alter the unit's behavior. While the core functionality remains the same, timing or resource usage might change. This could impact your test assertions, specifically if you're testing time-sensitive aspects or resource consumption.
Strategies for Modifying Test Expectations
Modifying test expectations effectively requires a structured approach. Here's a breakdown of key strategies:
1. Updating Assertions:
The most straightforward change often involves adjusting the assertions within your tests. If the expected output of your unit has changed, your assertions must reflect this. This might involve:
- Changing expected values: If your function previously returned "apple", but now returns "orange" due to a feature update, update your assertion to expect "orange".
- Modifying assertion types: You might need to switch from an equality assertion (
assertEqual
) to a different assertion type, such as aassertIn
or aassertTrue
, depending on the change in expected behavior. - Adding new assertions: A feature addition might necessitate adding new assertions to verify the correct behavior of the new functionality.
2. Mocking and Stubbing:
When dealing with changes in dependencies, effectively utilizing mocking and stubbing becomes critical. Instead of testing the actual behavior of external dependencies, you can mock their behavior, allowing you to control their interaction with the unit under test. This ensures that changes in the dependencies don't unexpectedly break your tests.
For example:
# Before update (using a real database)
from my_database import Database
def test_my_function():
db = Database()
result = my_function(db)
assert result == expected_value
# After update (mocking the database)
from unittest.mock import Mock
def test_my_function():
mock_db = Mock()
mock_db.query.return_value = mock_data # Mock the database's behavior
result = my_function(mock_db)
assert result == expected_value
This example demonstrates how mocking a database interaction isolates the test from potential changes in the database itself.
3. Parameterization:
For tests with multiple scenarios, parameterization proves incredibly helpful. You can define test cases using different input parameters and expected outputs. This allows for flexibility when modifying expectations. If a particular input's output changes, you only need to update that specific test case within the parameterized test suite.
import pytest
@pytest.mark.parametrize("input, expected", [
(1, 2),
(2, 4),
(3, 6), # Updated expectation
])
def test_my_function(input, expected):
result = my_function(input)
assert result == expected
4. Refactoring Tests:
In some cases, significant changes to your code might warrant refactoring your tests. This might involve restructuring the tests, breaking down large tests into smaller, more focused ones, or improving the overall organization of your test suite. This helps maintain test clarity and manageability as your code evolves.
5. Version Control and Collaboration:
Always use version control (like Git) to track changes to your code and tests. This allows you to easily revert to previous versions if needed. Furthermore, collaborative development practices and clear communication are essential. Team members should be aware of changes to the codebase and the corresponding implications for the tests.
Identifying and Addressing Test Failures
When test failures occur after code changes, it's crucial to investigate systematically:
- Reproduce the Failure: Ensure you can consistently reproduce the failure.
- Analyze the Failure: Carefully examine the failure message and trace the execution path to pinpoint the root cause.
- Is it a Real Bug or a Test Issue?: Determine if the failure points to an actual bug in the code or an outdated test.
- Update the Test: If the failure is due to a legitimate change in the code's behavior, update the test to reflect the new expectations.
- Commit Changes: Commit the code and test changes with clear, descriptive commit messages.
Common Pitfalls to Avoid
- Ignoring failing tests: Failing tests should never be ignored. They signal a problem that needs to be addressed, either in the code or in the tests themselves.
- Overly complex tests: Keeping tests concise and focused simplifies the process of identifying and fixing issues.
- Insufficient test coverage: Inadequate test coverage can lead to undetected regressions.
- Lack of documentation: Clear documentation of tests and their purpose is vital for maintainability.
- Ignoring the principle of least astonishment: Tests should be predictable and easy to understand; unexpected changes in test behavior should be treated with caution.
Conclusion (Part 1)
Effectively managing changing expectations in your unit tests is vital for maintaining the integrity and reliability of your software. Understanding when changes are needed, employing appropriate strategies for modifying expectations, and systematically addressing test failures are key to ensuring your tests continue to accurately reflect the evolving behavior of your application. The next part of this series will delve into advanced techniques and best practices for managing test expectations, including handling asynchronous operations and dealing with complex dependencies. Remember, consistent testing is a continuous process, requiring adaptation and refinement as your software grows and matures. By mastering the art of changing expectations in your unit tests, you’ll build a more robust and maintainable codebase.
Latest Posts
Latest Posts
-
Nurse Susan Is Completing The Discharge Process With Troy
Mar 31, 2025
-
Match Each Type Of Sales Channel To Its Corresponding Description
Mar 31, 2025
-
Human Population Growth Worksheet Pdf Answer Key
Mar 31, 2025
-
Which Of The Following Statements About Blood Is False
Mar 31, 2025
-
Modules 3 4 Operating System Overview Group Exam
Mar 31, 2025
Related Post
Thank you for visiting our website which covers about 3.11 Unit Test: Changing Expectations - Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.