3.12 Unit Test Polynomials - Part 1
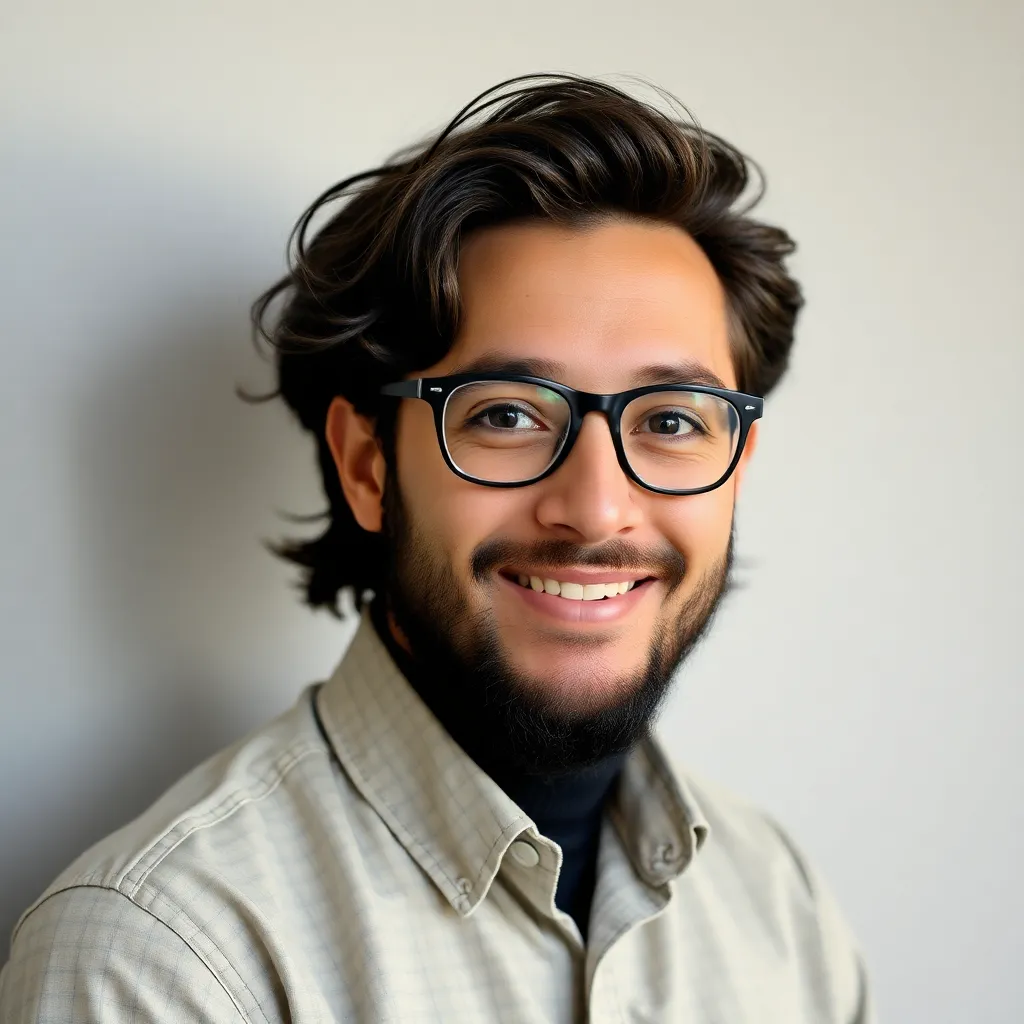
Onlines
Apr 24, 2025 · 5 min read

Table of Contents
3.12 Unit Test Polynomials - Part 1: Foundations and Essential Concepts
This comprehensive guide delves into the crucial world of unit testing polynomials, specifically focusing on the foundational concepts and techniques essential for building robust and reliable mathematical software. We'll explore various testing strategies, common pitfalls, and best practices, all within the context of a hypothetical 3.12 unit testing framework (though the principles apply broadly across testing frameworks). This is Part 1, laying the groundwork for more advanced techniques in subsequent parts.
Understanding Polynomial Representation
Before diving into testing, we must understand how polynomials are typically represented in code. Common representations include:
-
Coefficient Arrays: A polynomial like 3x² + 2x + 1 can be represented as an array
[3, 2, 1]
, where the index corresponds to the exponent (starting from 0). This is efficient for many operations. -
Linked Lists: Each term (coefficient and exponent) can be a node in a linked list, providing flexibility for polynomials with potentially very high or sparse exponents.
-
Object-Oriented Representation: A class
Polynomial
might encapsulate the coefficients, offering methods for addition, subtraction, multiplication, evaluation, etc. This is often preferred for larger projects due to better code organization and maintainability.
The choice of representation significantly impacts the design and implementation of your unit tests. We will primarily focus on the coefficient array representation due to its simplicity and widespread use in introductory contexts.
Essential Unit Test Cases for Polynomial Operations
Let's assume we have a Polynomial
class (or module) with methods for basic polynomial operations. We need to design unit tests covering a wide range of scenarios to ensure correctness and robustness. These tests should cover:
1. Polynomial Addition
-
Positive Test Cases:
- Adding two polynomials with equal degrees: Test cases should include polynomials of various degrees, ensuring the addition correctly combines like terms. Examples: (x + 1) + (2x + 3) = 3x + 4; (x² + x + 1) + (x² + 2x + 1) = 2x² + 3x + 2.
- Adding polynomials with unequal degrees: This covers scenarios where one polynomial has higher-order terms than the other. Example: (x² + 1) + (x + 2) = x² + x + 3.
- Adding polynomials with zero coefficients: Ensure correct handling of zero coefficients in the resulting polynomial. Example: (x² + 1) + (0) = x² + 1.
- Adding a polynomial to itself: A simple check for self-addition. Example: (x + 1) + (x + 1) = 2x + 2.
-
Negative Test Cases (Edge Cases):
- Null or empty polynomials: The function should gracefully handle cases where one or both inputs are null or empty arrays. It should likely return a copy of the non-null polynomial, or a zero polynomial.
- Invalid input types: The function should raise appropriate exceptions if inputs are not arrays or contain non-numeric values.
- Polynomials with very large coefficients or degrees: Test cases should consider the limitations of data types and potential overflow scenarios.
2. Polynomial Subtraction
Similar to addition, subtraction tests need to cover:
- Positive Test Cases: Include a variety of polynomials with equal and unequal degrees, including those with zero coefficients.
- Negative Test Cases: Address null/empty inputs, invalid input types, and scenarios that might lead to unexpected behavior (e.g., subtraction resulting in zero or negative coefficients).
3. Polynomial Multiplication
Polynomial multiplication is more complex, requiring more comprehensive tests:
-
Positive Test Cases:
- Multiplying two monomials: Simple cases like (2x) * (3x) = 6x².
- Multiplying a monomial by a polynomial: Example: (2x) * (x² + x + 1) = 2x³ + 2x² + 2x.
- Multiplying two polynomials of various degrees: Ensure correct expansion and combination of like terms. Example: (x + 1) * (x + 2) = x² + 3x + 2.
- Multiplying a polynomial by a constant: Example: 2 * (x² + x + 1) = 2x² + 2x + 2.
-
Negative Test Cases:
- Handle null/empty inputs.
- Handle invalid input types.
- Test for potential overflow with large coefficients.
4. Polynomial Evaluation
This involves substituting a value for 'x' and calculating the resulting value of the polynomial.
-
Positive Test Cases:
- Evaluate at various points: Choose various x-values (positive, negative, zero).
- Evaluate polynomials of different degrees.
- Evaluate with large or small input values.
-
Negative Test Cases:
- Handle invalid input types for x-values.
- Handle potential overflow situations.
Implementing Unit Tests Using a Hypothetical Framework (3.12)
While the specific syntax will vary depending on the actual framework, the core principles remain consistent. We'll use a pseudo-code representation for the 3.12 framework (which you can adapt to your chosen testing library like JUnit, pytest, or unittest):
# Hypothetical 3.12 Unit Testing Framework (pseudo-code)
@Test("Polynomial Addition: Equal Degree")
def test_add_equal_degree():
p1 = Polynomial([1, 2]) # x + 2
p2 = Polynomial([3, 4]) # 3x + 4
p_sum = p1.add(p2)
assert_equals(p_sum.coefficients, [4, 6]) # 4x + 6
@Test("Polynomial Addition: Unequal Degree")
def test_add_unequal_degree():
p1 = Polynomial([1, 2, 3]) # x² + 2x + 3
p2 = Polynomial([4, 5]) # 4x + 5
p_sum = p1.add(p2)
assert_equals(p_sum.coefficients, [1, 6, 8]) # x² + 6x + 8
@Test("Polynomial Multiplication")
def test_multiply():
p1 = Polynomial([1,1]) # x + 1
p2 = Polynomial([2,3]) # 2x + 3
p_prod = p1.multiply(p2)
assert_equals(p_prod.coefficients, [2,5,3]) # 2x² + 5x + 3
# ... more test cases ...
# Run tests:
run_tests()
This illustrates how you'd structure your unit tests. Each @Test
annotation designates a test case with a descriptive name. assert_equals
verifies the expected output against the actual result. The run_tests()
function executes all annotated test methods.
Best Practices for Unit Testing Polynomials
-
Use a robust testing framework: Leverage the features provided by established testing frameworks to manage test cases, assertions, and reporting.
-
Write clear and descriptive test names: Test names should clearly communicate the purpose and scope of each test case.
-
Prioritize edge cases: Pay special attention to boundary conditions and scenarios that are prone to errors (null inputs, empty polynomials, large coefficients, etc.).
-
Aim for high test coverage: Strive to cover all possible code paths and scenarios within your
Polynomial
class. -
Use mocking (for larger systems): If your polynomial operations are part of a larger system, you might use mocking techniques to isolate your polynomial tests from dependencies on other components.
Conclusion (Part 1)
This first part establishes a strong foundation for unit testing polynomials. We've explored various polynomial representations, outlined essential test cases for core operations (addition, subtraction, multiplication, evaluation), and demonstrated a basic approach to implementing these tests using a hypothetical framework. In the subsequent parts, we will delve into more advanced topics, such as testing for polynomial division, root finding algorithms, and handling complex numbers, along with integrating more sophisticated testing strategies and techniques. Remember that thorough unit testing is crucial for the reliability and correctness of your mathematical software. By following the principles and practices discussed here, you can build robust and maintainable polynomial-handling code.
Latest Posts
Latest Posts
-
Which Is Not A Lifespan Consideration For Sensory Perception
Apr 24, 2025
-
2020 Practice Exam 1 Mcq Ap Csp
Apr 24, 2025
-
Room With A View Book Summary
Apr 24, 2025
-
To Kill A Mocking Bird Chapter 1 Summary
Apr 24, 2025
-
Language Handbook 1 The Parts Of Speech
Apr 24, 2025
Related Post
Thank you for visiting our website which covers about 3.12 Unit Test Polynomials - Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.