4.17 Lab Mad Lib - Loops
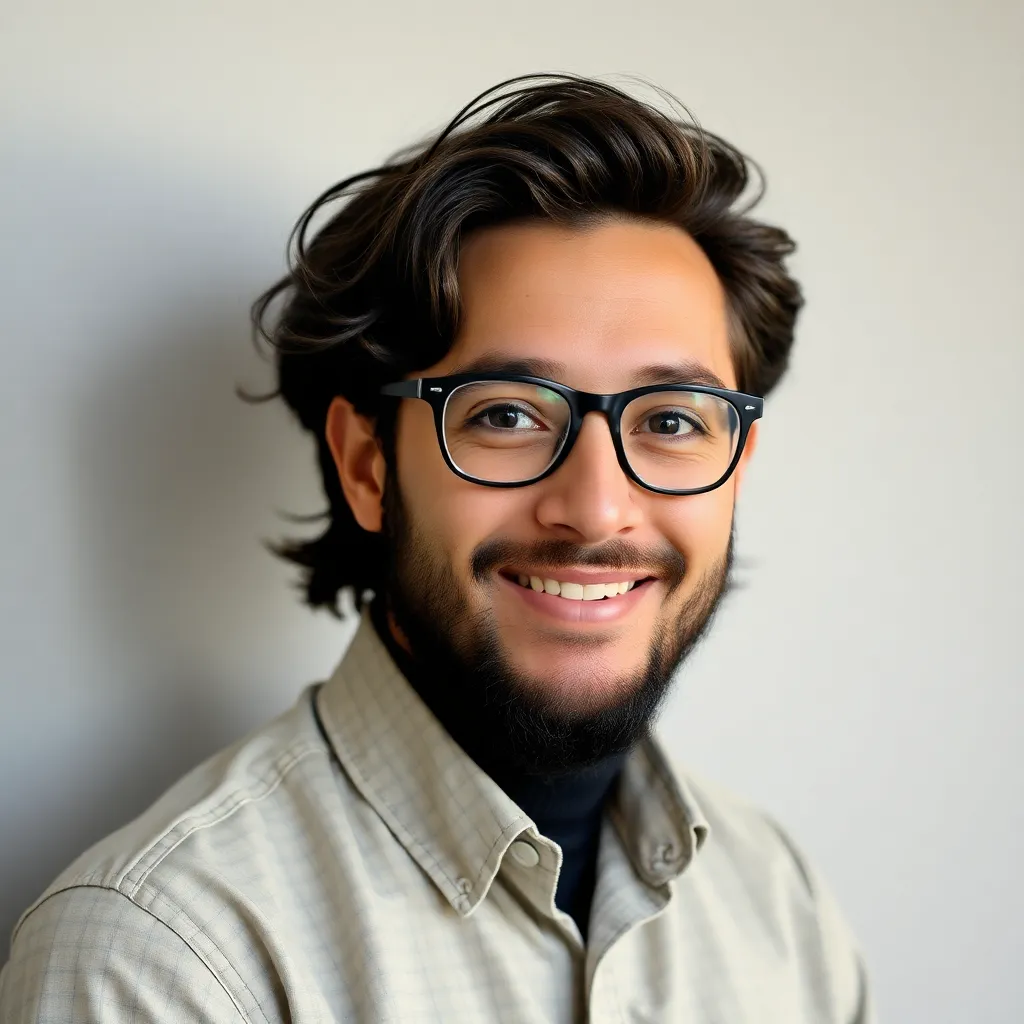
Onlines
Apr 01, 2025 · 6 min read
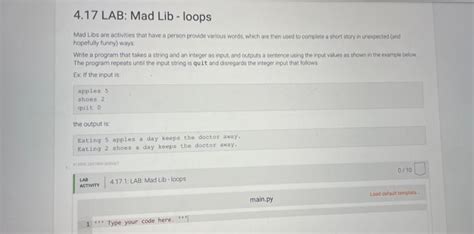
Table of Contents
4.17 Lab Mad Libs - Loops: A Deep Dive into Programming Fun
This article delves into the fascinating world of programming loops, using the whimsical context of Mad Libs as a playful yet effective teaching tool. We'll explore different types of loops – for
loops and while
loops – and how they can be implemented in various programming languages to create dynamic and interactive Mad Libs games. Beyond the fun, we'll cover crucial programming concepts such as user input, string manipulation, and conditional statements, all while building a robust and entertaining Mad Libs application.
Understanding Loops: The Heart of Iteration
Loops are fundamental programming constructs that allow you to execute a block of code repeatedly. This repetitive execution is crucial for tasks requiring iteration, such as processing lists, generating sequences, or, in our case, building a Mad Libs story. There are primarily two types of loops:
for
Loops: Iterating Through Known Iterations
for
loops are ideal when you know the number of times you need to repeat a block of code. They typically involve a counter variable that increments or decrements with each iteration until a specified condition is met. For example, if you need to ask the user for five different words, a for
loop is perfect because you know the exact number of iterations required.
Example (Python):
for i in range(5):
word = input(f"Enter word {i+1}: ")
# ... process the word ...
This Python code uses a for
loop to iterate five times. The range(5)
function generates a sequence of numbers from 0 to 4, and the loop body (the indented lines) executes once for each number.
while
Loops: Iterating Until a Condition is Met
while
loops are used when the number of iterations is not known beforehand. The loop continues to execute as long as a specified condition remains true. This is particularly useful in scenarios where the loop needs to terminate based on user input or some other dynamic condition.
Example (JavaScript):
let keepGoing = true;
while (keepGoing) {
let word = prompt("Enter a word (or type 'quit' to exit):");
if (word.toLowerCase() === "quit") {
keepGoing = false;
} else {
// ... process the word ...
}
}
This JavaScript example uses a while
loop that continues until the user types "quit". The keepGoing
variable acts as a flag, controlling the loop's execution.
Building Our Mad Libs Application: A Step-by-Step Guide
Now let's build our Mad Libs application. We'll use Python for its simplicity and readability, but the concepts can be easily adapted to other languages.
1. Defining the Story Template:
First, we need a story template. This will contain placeholders for the words the user will provide. We'll represent these placeholders using bracketed words, like {adjective}
, {noun}
, {verb}
, etc.
story_template = """
Once upon a time, in a land filled with {adjective} {nouns}, lived a {adjective} {noun}.
This {noun} loved to {verb} {adverbs} in the {adjective} {noun}. One day, the {noun} met a {adjective} {noun} who was {verb}ing {adverbs}.
The end.
"""
2. Gathering User Input Using Loops:
We'll use a for
loop to iterate through the list of word types needed for our story, prompting the user for each word. We'll store these words in a dictionary for easy access later.
word_types = ["adjective", "nouns", "adjective", "noun", "noun", "verb", "adverbs", "adjective", "noun", "noun", "adjective", "noun", "verb", "adverbs"]
word_dict = {}
for word_type in word_types:
while True:
word = input(f"Please enter a {word_type}: ")
if word: #check if user enters something
word_dict[word_type] = word
break
else:
print("Please enter a valid word.")
This code ensures that the user provides a valid input for each word type. It uses a nested while
loop to ensure that the user doesn't skip any input fields.
3. Populating the Story Template:
Once we have all the words, we can use string formatting to replace the placeholders in the story template with the user's input. Python's str.format()
method is well-suited for this task.
filled_story = story_template.format(**word_dict)
The **word_dict
unpacks the dictionary into keyword arguments for the format()
method.
4. Displaying the Completed Mad Libs Story:
Finally, we print the completed Mad Libs story to the console.
print("\nYour Mad Libs story:\n")
print(filled_story)
Complete Python Code:
story_template = """
Once upon a time, in a land filled with {adjective} {nouns}, lived a {adjective} {noun}.
This {noun} loved to {verb} {adverbs} in the {adjective} {noun}. One day, the {noun} met a {adjective} {noun} who was {verb}ing {adverbs}.
The end.
"""
word_types = ["adjective", "nouns", "adjective", "noun", "noun", "verb", "adverbs", "adjective", "noun", "noun", "adjective", "noun", "verb", "adverbs"]
word_dict = {}
for word_type in word_types:
while True:
word = input(f"Please enter a {word_type}: ")
if word:
word_dict[word_type] = word
break
else:
print("Please enter a valid word.")
filled_story = story_template.format(**word_dict)
print("\nYour Mad Libs story:\n")
print(filled_story)
Expanding the Mad Libs Application: Advanced Techniques
This basic Mad Libs application provides a strong foundation. Here are some ways to expand its functionality:
1. Error Handling and Input Validation:
The current code does basic input validation, but you could enhance it by checking for specific word types (e.g., ensuring the user inputs a plural noun when requested). You could also add more robust error handling to gracefully manage unexpected input, such as non-string entries.
2. Multiple Story Templates:
You can expand the program to include multiple story templates, allowing users to choose which story they want to play. This could be implemented by storing the templates in a list or dictionary and allowing the user to select one using a menu.
3. File Input/Output:
Storing story templates in separate files allows for easier management and expansion of the game's content. Similarly, saving generated stories to a file allows users to review or share their creations.
4. GUI Implementation:
For a more user-friendly experience, consider implementing a graphical user interface (GUI) using libraries like Tkinter (Python), Swing (Java), or similar GUI frameworks for other languages. This would replace the command-line interface with a visually appealing and intuitive experience.
5. Advanced String Manipulation:
Explore advanced string manipulation techniques to handle more complex story templates, potentially incorporating sentence structure analysis or even basic natural language processing (NLP) to personalize the story generation.
Conclusion: Loops, Mad Libs, and the Joy of Programming
This exploration of loops within the context of a Mad Libs game showcases the power and versatility of these programming constructs. By understanding how to effectively use for
and while
loops, along with techniques for user input, string manipulation, and error handling, you can create engaging and dynamic applications. The Mad Libs game serves as a fun and accessible way to learn and reinforce these important programming concepts, demonstrating that learning to code can be both educational and entertaining. Remember to continue experimenting and expanding upon these fundamentals to build your programming skills and create increasingly sophisticated applications.
Latest Posts
Latest Posts
-
Which Technology Is Shown In The Diagram
Apr 02, 2025
-
Selecciona La Palabra Que No Esta Relacionada
Apr 02, 2025
-
Refer To The Graphic What Type Of Cabling Is Shown
Apr 02, 2025
-
Activity A Continued From Previous Page
Apr 02, 2025
-
Separate But Equal Movie Questions Answer Key
Apr 02, 2025
Related Post
Thank you for visiting our website which covers about 4.17 Lab Mad Lib - Loops . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.