5.06 Unit Test Critical Skills Practice 3
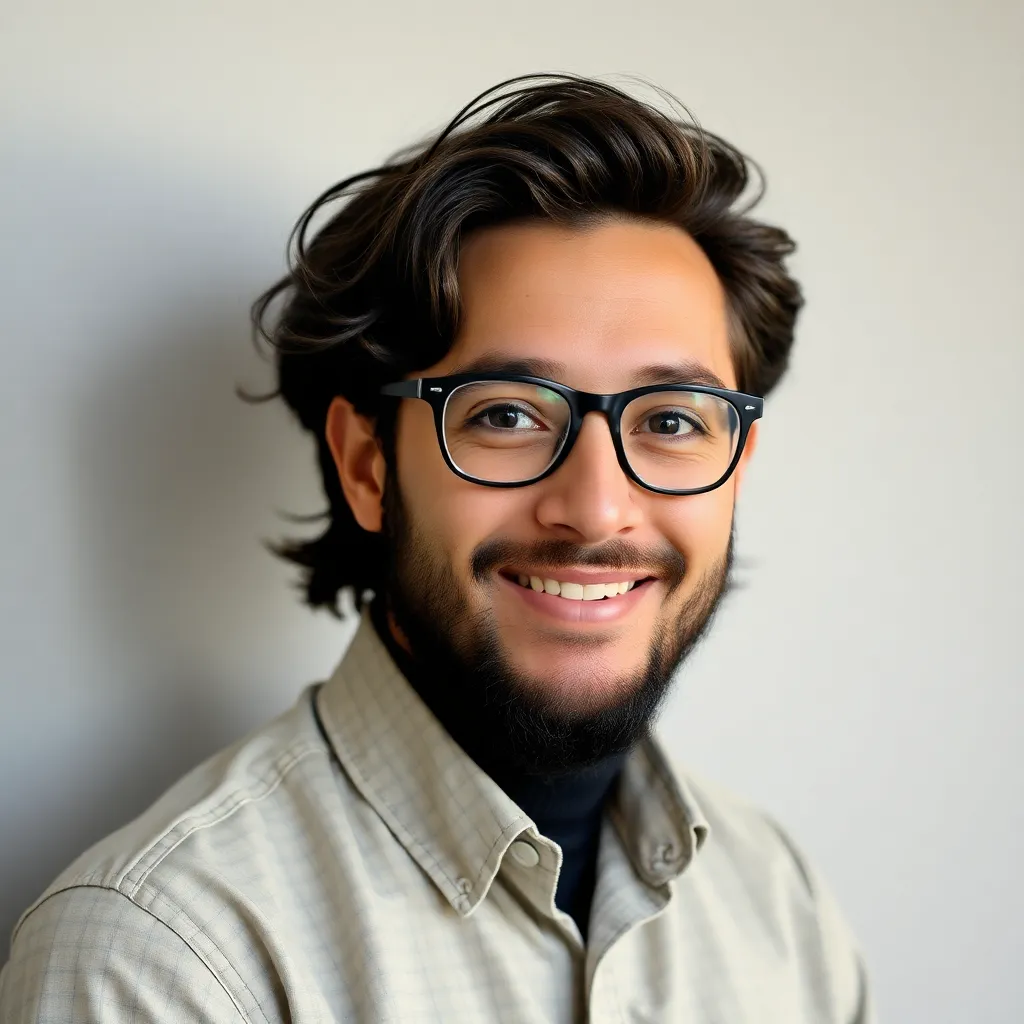
Onlines
Mar 18, 2025 · 5 min read

Table of Contents
Mastering Unit Testing: A Deep Dive into Critical Skills and Practice 3 of 5.06
Unit testing, a cornerstone of software development best practices, ensures individual components of your code function correctly in isolation. This in-depth guide focuses on Practice 3 of 5.06 Unit Testing Critical Skills, examining the core principles and advanced techniques to elevate your testing proficiency. We'll delve into practical examples, common pitfalls to avoid, and best practices for writing robust and maintainable unit tests.
While a specific "5.06 Unit Testing Critical Skills Practice 3" lacks standardized universal context, this article addresses the typical skills and challenges encountered within this presumed stage of a unit testing curriculum. We'll assume this practice involves more complex scenarios, requiring a deeper understanding of mocking, dependency injection, and test-driven development (TDD).
Understanding the Fundamentals: Before Diving into Practice 3
Before tackling advanced techniques, let's briefly revisit fundamental concepts:
What is Unit Testing?
Unit testing involves testing individual units or modules of code in isolation to ensure they function as expected. This contrasts with integration testing (testing the interaction between modules) and system testing (testing the entire system). The goal is to identify and fix bugs early in the development lifecycle, reducing overall development time and cost.
Key Benefits of Unit Testing
- Early Bug Detection: Identify and resolve issues before they propagate into larger problems.
- Improved Code Quality: Writing testable code often leads to cleaner, more modular designs.
- Reduced Debugging Time: Isolating bugs becomes significantly easier with well-structured unit tests.
- Increased Confidence in Refactoring: Unit tests provide a safety net when making code changes.
- Improved Code Maintainability: Tests serve as living documentation, demonstrating how code should function.
Essential Testing Frameworks
Various testing frameworks simplify the process of writing and running unit tests. Popular choices include:
- JUnit (Java): A widely used framework for Java unit testing.
- pytest (Python): A versatile and popular framework for Python.
- Jest (JavaScript): A powerful framework for JavaScript, often used with React, Angular, and Vue.js.
- NUnit (.NET): A popular framework for .NET applications.
- PHPUnit (PHP): A widely adopted framework for PHP.
The specific framework used depends on the programming language and project requirements. However, the underlying principles of unit testing remain consistent across all frameworks.
Delving into Practice 3: Advanced Unit Testing Techniques
Practice 3, assuming a progression of skills, likely introduces more complex scenarios requiring advanced techniques:
1. Mastering Mocking and Stubbing
Mocking and stubbing are crucial for isolating units under test. They allow you to replace dependencies with simulated objects that control their behavior.
-
Mocking: Creating a simulated object that mimics the behavior of a real dependency. Mocking often involves verifying interactions (e.g., ensuring a method was called with specific parameters). Frameworks like Mockito (Java) and
unittest.mock
(Python) provide powerful mocking capabilities. -
Stubbing: Creating a simulated object that returns predetermined values. Stubbing focuses on controlling the output of a dependency, without necessarily verifying interactions.
Example (Python with unittest.mock
):
from unittest import mock
class MyClass:
def __init__(self, dependency):
self.dependency = dependency
def my_method(self):
return self.dependency.some_method()
# Mocking
with mock.patch('__main__.MyDependency.some_method', return_value='mocked_value'):
my_object = MyClass(MyDependency())
result = my_object.my_method()
assert result == 'mocked_value'
This example demonstrates how to mock the some_method
of MyDependency
to control the outcome of my_method
.
2. Effective Use of Dependency Injection
Dependency injection is a design pattern that promotes loose coupling and testability. Instead of creating dependencies within a class, they are injected from the outside. This allows for easy substitution of dependencies with mocks or stubs during testing.
Example (Python):
class MyDependency:
def some_method(self):
return "real value"
class MyClass:
def __init__(self, dependency):
self.dependency = dependency
def my_method(self):
return self.dependency.some_method()
# Dependency Injection for testing:
mock_dependency = mock.Mock()
mock_dependency.some_method.return_value = "mocked value"
my_object = MyClass(mock_dependency)
result = my_object.my_method()
assert result == "mocked value"
This shows how injecting a mock dependency simplifies testing.
3. Handling Asynchronous Operations
Testing asynchronous operations (like network requests or database interactions) requires special techniques. Often, you'll need to use asynchronous testing frameworks or leverage features like asyncio
(Python) or Promises (JavaScript) to handle asynchronous code gracefully within your tests.
4. Test-Driven Development (TDD)
TDD is a development approach where you write tests before writing the actual code. This forces you to think carefully about the design and behavior of your code, leading to more robust and well-structured units. The "Red-Green-Refactor" cycle is central to TDD:
- Red: Write a failing test that defines the desired behavior.
- Green: Write the minimum amount of code necessary to pass the test.
- Refactor: Improve the code's design and readability while ensuring the tests still pass.
5. Advanced Assertion Techniques
Beyond simple assertEqual
assertions, explore more advanced assertion techniques:
assertIn
/assertNotIn
: Verify if an element is present (or absent) in a collection.assertTrue
/assertFalse
: Verify boolean conditions.assertRaises
: Verify that an exception is raised.- Custom Assertions: Create your own assertions to express specific testing needs.
Common Pitfalls to Avoid
- Over-Testing: Don't test trivial details; focus on essential functionality.
- Under-Testing: Insufficient testing leaves vulnerabilities. Aim for good test coverage.
- Ignoring Edge Cases: Pay attention to boundary conditions and unusual inputs.
- Test Coupling: Avoid tests that depend on each other; keep them independent.
- Slow Tests: Optimize your tests for speed to maintain developer productivity.
- Ignoring Test Failures: Address failing tests promptly; they indicate issues in your code.
Best Practices for Writing Maintainable Unit Tests
- Use Descriptive Test Names: Clearly indicate the purpose of each test.
- Keep Tests Small and Focused: Each test should verify a single aspect of functionality.
- Follow a Consistent Structure: Maintain a consistent pattern for writing and organizing tests.
- Use a Version Control System: Track changes to your tests and codebase.
- Automate Testing: Integrate tests into your build process for continuous integration.
- Review Your Tests Regularly: Keep tests up-to-date as your code evolves.
Conclusion: Elevating Your Unit Testing Skills
Mastering unit testing is an ongoing process. This in-depth look at "Practice 3 of 5.06 Unit Testing Critical Skills" has highlighted advanced techniques like mocking, dependency injection, and test-driven development. By mastering these skills and following best practices, you can build a robust testing strategy that enhances code quality, reduces bugs, and accelerates development. Remember, the investment in thorough unit testing pays off in the long run with more reliable, maintainable, and ultimately successful software projects. Continuously refine your skills, explore new tools and techniques, and always strive to improve your testing methodologies to ensure your software consistently meets its objectives.
Latest Posts
Latest Posts
-
Week 3 Checkpoint Quiz Critical Reasoning
Mar 18, 2025
-
Edulastic Formative And Summative Assessments Made Easy Answers Key
Mar 18, 2025
-
Choose The Statement That Is True Concerning Hemoglobin
Mar 18, 2025
-
Advanced Hardware Lab 4 4 Troubleshoot The Motherboard Processor And Ram
Mar 18, 2025
-
All Of The Following Are True Regarding Rebates Except
Mar 18, 2025
Related Post
Thank you for visiting our website which covers about 5.06 Unit Test Critical Skills Practice 3 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.