5.11 Unit Test The Power Of Language - Part 1
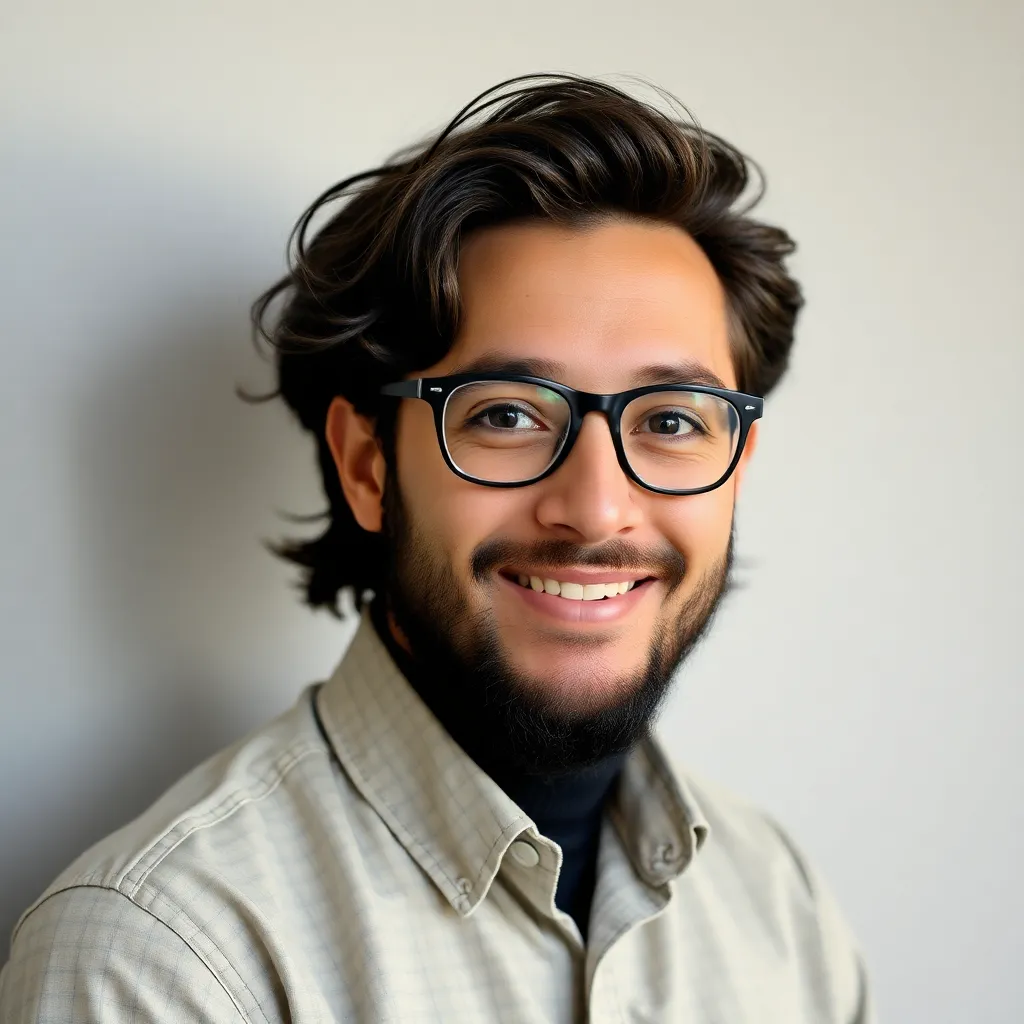
Onlines
Mar 21, 2025 · 6 min read
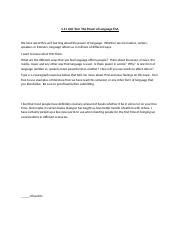
Table of Contents
5.11 Unit Test: The Power of Language - Part 1
The world of software development thrives on precision. Every line of code, every function, every interaction needs to function flawlessly. This is where unit testing comes in, providing a crucial safety net to catch errors before they reach production. But effective unit testing is more than just writing assertions; it's about crafting clear, concise, and powerful language in your tests. This is the first part of a two-part series exploring the profound impact of language on the effectiveness of your 5.11 (or any other) unit tests. We'll delve into the principles of creating tests that are not only functional but also highly readable, maintainable, and ultimately, powerful.
The Importance of Readable Unit Tests
Imagine a codebase littered with cryptic, poorly named unit tests. Debugging becomes a nightmare, collaboration turns into a frustrating exercise, and maintaining the code becomes an overwhelming task. The direct consequence? Increased development time, higher costs, and a greater risk of introducing bugs.
Conversely, well-written unit tests are a developer's best friend. They act as living documentation, explaining the intended behavior of the code. They're easy to understand, maintain, and extend. This fosters collaboration, speeds up development, and ultimately leads to higher-quality software. The key ingredient? Effective use of language.
Choosing Descriptive Names
The most crucial aspect of writing powerful unit tests is using descriptive names. Avoid cryptic abbreviations or vague terms. Instead, opt for clear, self-explanatory names that immediately convey the purpose of the test.
Consider this example (Python):
Bad:
def test_func1():
# ... test logic ...
Good:
def test_calculate_total_price_returns_correct_value_when_discount_applied():
# ... test logic ...
The "good" example clearly communicates what the test verifies. It's immediately apparent to anyone reading the code what the function is testing. This level of clarity significantly improves readability and maintainability.
The Power of Assertions
Assertions are the heart of any unit test. They verify that the code behaves as expected. Choosing the right assertion is vital for effective testing. Different assertion libraries offer a variety of options, each with its own purpose.
Utilizing Different Assertion Types
Avoid simply checking for equality (assertEqual
). Explore other assertion types to express your expectations more precisely:
assertTrue
/assertFalse
: Ideal for testing boolean conditions. They make the test's intent very clear.assertIn
/assertNotIn
: Excellent for checking membership in a collection (list, set, etc.). This enhances the readability when dealing with collections.assertRaises
: Used for checking exceptions. It explicitly verifies that a specific exception is raised under certain conditions. This is crucial for robust error handling.assertIs
/assertIsNot
: Checks object identity, differentiating between equality and the same object in memory. This is particularly useful for testing object immutability.
Example (Python using unittest
):
import unittest
class TestMyFunction(unittest.TestCase):
def test_positive_number_returns_positive(self):
self.assertTrue(my_function(5) > 0)
def test_zero_returns_zero(self):
self.assertEqual(my_function(0), 0)
def test_negative_number_raises_exception(self):
with self.assertRaises(ValueError):
my_function(-5)
This shows how different assertion types clearly communicate the specific expectations of each test case.
Structuring Your Tests for Clarity
The structure of your unit tests significantly impacts readability. Organize your tests logically, grouping related tests together. Use descriptive comments to explain complex logic or edge cases. A well-structured test suite is easier to navigate, understand, and debug.
Using Test Fixtures
Test fixtures provide a way to set up and tear down resources needed for your tests. They ensure that each test starts with a clean slate, preventing unintended side effects. Frameworks like pytest
and unittest
provide mechanisms for creating fixtures. This avoids code duplication and improves test organization.
Example (Python using pytest
):
import pytest
@pytest.fixture
def my_database():
# ... setup database connection ...
yield db_connection
# ... tear down database connection ...
def test_database_connection(my_database):
# ... test logic using my_database ...
This fixture ensures that a database connection is established before each test and closed afterwards, preventing resource leaks and ensuring consistent testing.
The Importance of Comments
While clear code often diminishes the need for extensive comments, strategic commenting significantly enhances readability. Use comments to explain:
- Why a particular test is necessary: Clarify the reasoning behind a specific test case, particularly if it deals with unusual scenarios or edge cases.
- Complex logic: If a particular test's implementation is intricate, add comments to break down the logic into manageable steps. This helps others (and your future self) understand the test.
- Expected behavior: Clearly state the expected outcome of the test. This serves as a clear reference point when reviewing the test.
Avoid redundant comments that simply restate the obvious code. Focus on adding value by clarifying the intent and reasoning behind the test.
Beyond Syntax: The Art of Expressive Testing
Effective unit testing goes beyond simply writing code that passes. It's about writing tests that are expressive, conveying the intent and behavior in a clear and concise manner.
Choosing Descriptive Variable Names
Just as function names are crucial, descriptive variable names within the tests themselves are equally important. Avoid single-letter variables or meaningless names. Use names that reflect the data's purpose within the specific test context. This adds another layer of clarity and readability.
Keeping Tests Concise
Avoid overly long or complex tests. Break down large tests into smaller, more focused ones. This improves readability, maintainability, and helps isolate failures more effectively. Aim for a single assertion per test whenever possible (though this is not always practical). The goal is to test one specific aspect of the system at a time.
Example: Testing a Simple Function
Let's illustrate these principles with a concrete example. Consider a simple function that calculates the area of a rectangle:
def calculate_rectangle_area(length, width):
if length <= 0 or width <= 0:
raise ValueError("Length and width must be positive values.")
return length * width
Here's how we might write unit tests for this function using pytest
:
import pytest
def test_calculate_rectangle_area_positive_values():
assert calculate_rectangle_area(5, 10) == 50
def test_calculate_rectangle_area_zero_length_raises_error():
with pytest.raises(ValueError):
calculate_rectangle_area(0, 10)
def test_calculate_rectangle_area_zero_width_raises_error():
with pytest.raises(ValueError):
calculate_rectangle_area(5, 0)
def test_calculate_rectangle_area_negative_length_raises_error():
with pytest.raises(ValueError):
calculate_rectangle_area(-5, 10)
def test_calculate_rectangle_area_negative_width_raises_error():
with pytest.raises(ValueError):
calculate_rectangle_area(5, -10)
Notice how each test is concise and focuses on a specific aspect of the function's behavior. The names clearly indicate what each test verifies. The use of pytest.raises
simplifies the exception handling logic.
Conclusion: Laying the Foundation for Powerful Tests
In this first part, we've explored the fundamental principles of crafting powerful unit tests through the effective use of language. We've covered choosing descriptive names, leveraging diverse assertion types, structuring tests for clarity, using comments strategically, and writing expressive, concise tests. By implementing these best practices, you dramatically improve the readability, maintainability, and overall effectiveness of your test suite. In Part 2, we will dive deeper into advanced testing techniques and explore how to effectively handle complex scenarios and edge cases, further strengthening your unit testing capabilities. Remember, well-written unit tests are an investment that pays off handsomely in the long run. They are the cornerstone of robust, reliable, and maintainable software.
Latest Posts
Latest Posts
-
Final Exam For Is 100 C Answers
Mar 28, 2025
-
James Stewart Calculus 8th Edition Solutions Pdf
Mar 28, 2025
-
Summary Chapter 2 To Kill A Mockingbird
Mar 28, 2025
-
Chapter 18 Summary Of The Giver
Mar 28, 2025
-
The Cpt Code For Posting Mr Bowdens Payment Is
Mar 28, 2025
Related Post
Thank you for visiting our website which covers about 5.11 Unit Test The Power Of Language - Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.