5.12.1 Change Order Of Elements In Function List Argument
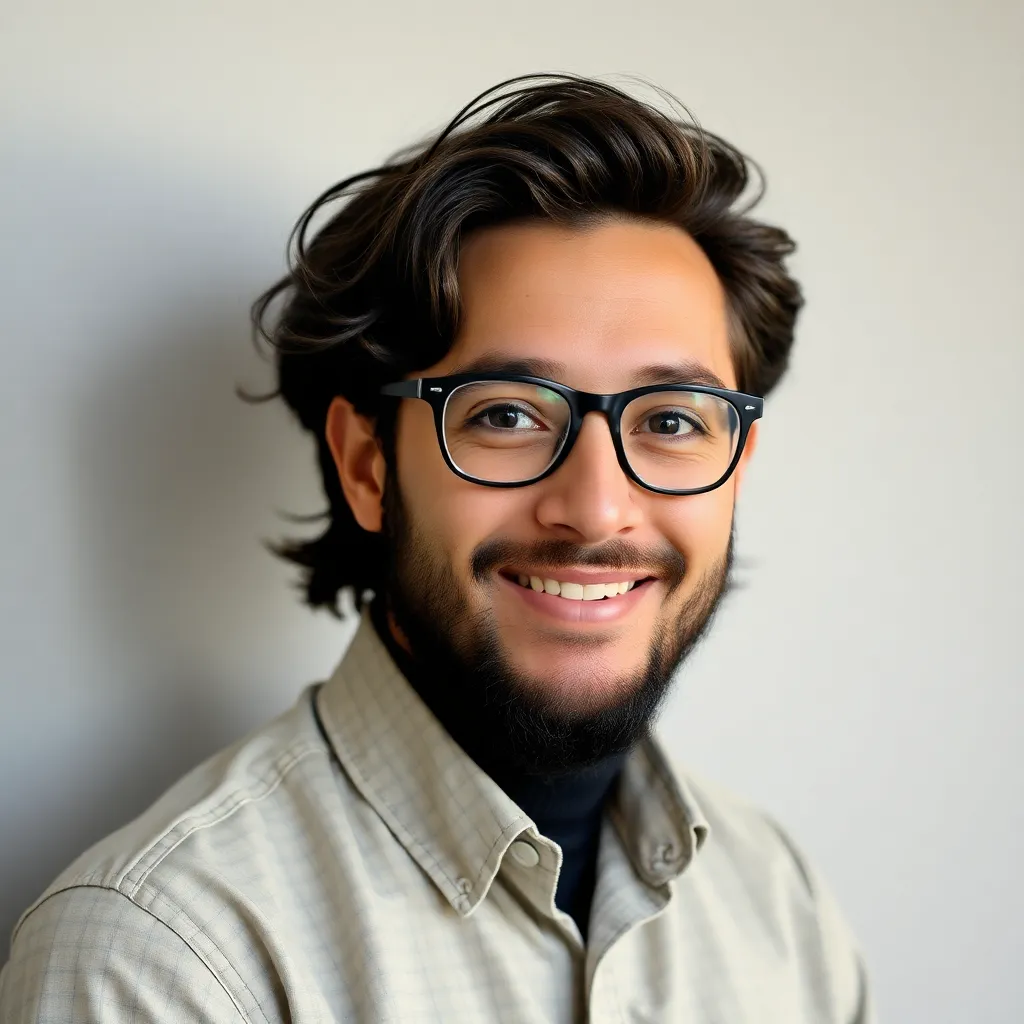
Onlines
May 12, 2025 · 6 min read
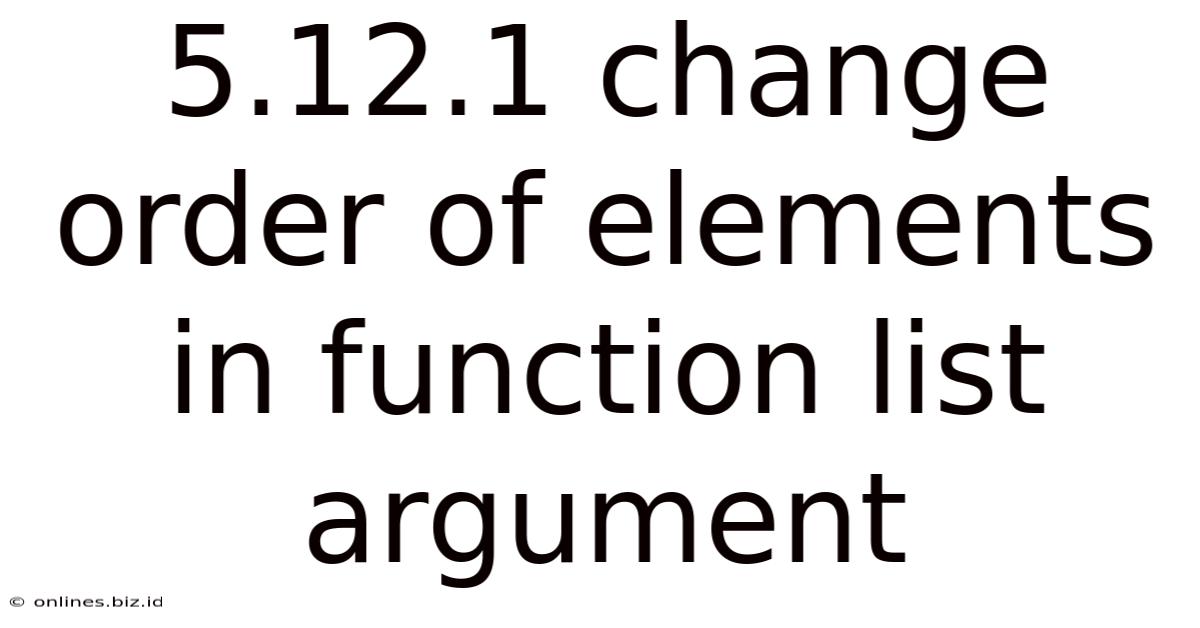
Table of Contents
5.12.1 Change Order of Elements in Function List Argument: A Comprehensive Guide
Understanding how to manipulate function arguments, specifically list arguments, is crucial for writing efficient and flexible Python code. This in-depth guide focuses on methodically altering the order of elements within a list passed as an argument to a function. We'll explore various techniques, best practices, and potential pitfalls, equipping you with the knowledge to confidently handle this common programming task.
Understanding the Problem: Why Reorder List Arguments?
Before diving into solutions, let's establish the context. Why would you need to change the order of elements in a list passed as a function argument? Several scenarios necessitate this:
- Data Processing & Analysis: Imagine analyzing sensor data where the order of readings is initially incorrect. Reordering the list within a function allows for accurate analysis.
- Algorithm Implementation: Many algorithms (sorting, searching) require specific input order. A function might need to rearrange the list to meet these algorithm requirements.
- User Interface/UX Improvements: Perhaps you're building a user interface where displayed items need to be reordered based on user preferences. A function could handle this rearrangement.
- Flexibility & Reusability: Functions that accept lists as arguments become far more versatile if they can handle different orderings, increasing code reusability.
Methods for Reordering List Arguments in Python
Python provides several ways to reorder elements within a list passed as a function argument. Let's examine the most common and effective approaches:
1. Using Slicing and Concatenation
This is a straightforward method ideal for simple reorderings. It involves extracting portions of the list using slicing and then concatenating them in the desired order.
def reorder_list(data):
"""Reorders a list using slicing and concatenation."""
# Example: Move the last element to the beginning
reordered_data = data[-1:] + data[:-1]
return reordered_data
my_list = [1, 2, 3, 4, 5]
reordered_list = reorder_list(my_list)
print(f"Original List: {my_list}")
print(f"Reordered List: {reordered_list}")
This example moves the last element to the beginning. You can adapt the slicing indices to achieve various reorderings. However, for complex reorderings, this approach can become cumbersome and less readable.
2. Using the sorted()
function with a custom key
The sorted()
function, combined with a custom key
function, offers a powerful approach for reordering based on specific criteria. This is particularly useful when the reordering logic is complex or involves multiple criteria.
def reorder_by_criteria(data, criteria):
"""Reorders a list based on a custom criteria function."""
return sorted(data, key=criteria)
my_list = [(1, 'b'), (3, 'a'), (2, 'c')]
# Reorder by the second element of each tuple (string)
def criteria_by_string(item):
return item[1]
reordered_list = reorder_by_criteria(my_list, criteria_by_string)
print(f"Original List: {my_list}")
print(f"Reordered List (by string): {reordered_list}")
#Reorder by the first element of each tuple (number)
def criteria_by_number(item):
return item[0]
reordered_list = reorder_by_criteria(my_list, criteria_by_number)
print(f"Reordered List (by number): {reordered_list}")
Here, the criteria
function determines the sorting order. You can define complex logic within this function to meet your specific needs. Remember, sorted()
returns a new sorted list; the original list remains unchanged.
3. In-place Reordering with List Methods (insert()
, pop()
, append()
)
For in-place modification (directly changing the original list), you can use list methods like insert()
, pop()
, and append()
. This approach is efficient for smaller lists and simpler reorderings but can become less manageable for more complex scenarios.
def reorder_in_place(data):
"""Reorders a list in-place using list methods."""
last_element = data.pop() #removes the last element and assigns it to last_element
data.insert(0, last_element) # inserts last_element at index 0
return data
my_list = [1, 2, 3, 4, 5]
reordered_list = reorder_in_place(my_list)
print(f"Original List (modified in place): {my_list}")
print(f"Reordered List (modified in place): {reordered_list}")
This example demonstrates moving the last element to the beginning in-place. Be cautious with in-place modifications, as they can lead to unexpected behavior if not handled carefully.
4. Leveraging the reversed()
function
If you need to reverse the order of elements, the built-in reversed()
function provides a concise solution. Note that reversed()
returns an iterator, which you need to convert back to a list if necessary.
def reverse_list(data):
"""Reverses a list using the reversed() function."""
return list(reversed(data))
my_list = [1, 2, 3, 4, 5]
reversed_list = reverse_list(my_list)
print(f"Original List: {my_list}")
print(f"Reversed List: {reversed_list}")
This method is particularly efficient for reversing lists.
5. Using list.sort()
with a custom key
(In-place Sorting)
Similar to sorted()
, list.sort()
allows in-place sorting based on a custom key
function. This modifies the original list directly.
def reorder_in_place_with_key(data, criteria):
"""Reorders a list in-place using list.sort() with a custom key."""
data.sort(key=criteria)
return data
my_list = [(1, 'b'), (3, 'a'), (2, 'c')]
#Reorder by the second element of each tuple (string)
def criteria_by_string(item):
return item[1]
reordered_list = reorder_in_place_with_key(my_list, criteria_by_string)
print(f"Original List (modified in place): {my_list}")
print(f"Reordered List (modified in place, by string): {reordered_list}")
Remember that list.sort()
modifies the list in-place, unlike sorted()
. Choose the appropriate method based on whether you need to preserve the original list.
Choosing the Right Method: Best Practices
The optimal method depends on the specific reordering requirement and your coding preferences:
- Simple Reorderings: Slicing and concatenation or
insert()
,pop()
,append()
are suitable for simple cases. - Complex Reorderings based on Criteria: Use
sorted()
orlist.sort()
with a customkey
function. This promotes readability and maintainability, especially for intricate logic. - Reversing: The
reversed()
function offers the most concise solution for reversing lists. - In-place vs. Creating a New List: Consider whether you need to modify the original list or create a new one. In-place modification is generally more efficient but can be less predictable if not handled carefully.
Advanced Scenarios and Considerations
-
Handling Large Datasets: For extremely large lists, consider the efficiency implications of each method.
sorted()
andlist.sort()
often have optimized implementations for better performance with large datasets. -
Error Handling: Implement robust error handling to manage potential exceptions (e.g.,
TypeError
if thekey
function is incompatible with the list elements). -
Immutability: Remember that tuples are immutable; you cannot directly reorder elements within a tuple. You'd need to convert the tuple to a list, perform the reordering, and then convert it back if needed.
Conclusion: Mastering List Reordering
Understanding how to effectively reorder elements within a list passed as a function argument is a crucial skill for any Python programmer. This guide has covered multiple approaches, from simple slicing and concatenation to sophisticated sorting with custom key
functions. By carefully considering your specific needs and adopting best practices, you can write efficient, readable, and maintainable Python code that elegantly handles list reordering. Remember to choose the method that best suits your complexity and performance requirements, paying close attention to whether you need in-place modification or the creation of a new list. Mastering these techniques empowers you to write more flexible and robust Python programs.
Latest Posts
Latest Posts
-
According To The Chart When Did A Pdsa Cycle Occur
May 12, 2025
-
Bioflix Activity Gas Exchange The Respiratory System
May 12, 2025
-
Economic Value Creation Is Calculated As
May 12, 2025
-
Which Items Typically Stand Out When You Re Scanning Text
May 12, 2025
-
Assume That Price Is An Integer Variable
May 12, 2025
Related Post
Thank you for visiting our website which covers about 5.12.1 Change Order Of Elements In Function List Argument . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.