Activity Guide Input And Output Answer Key
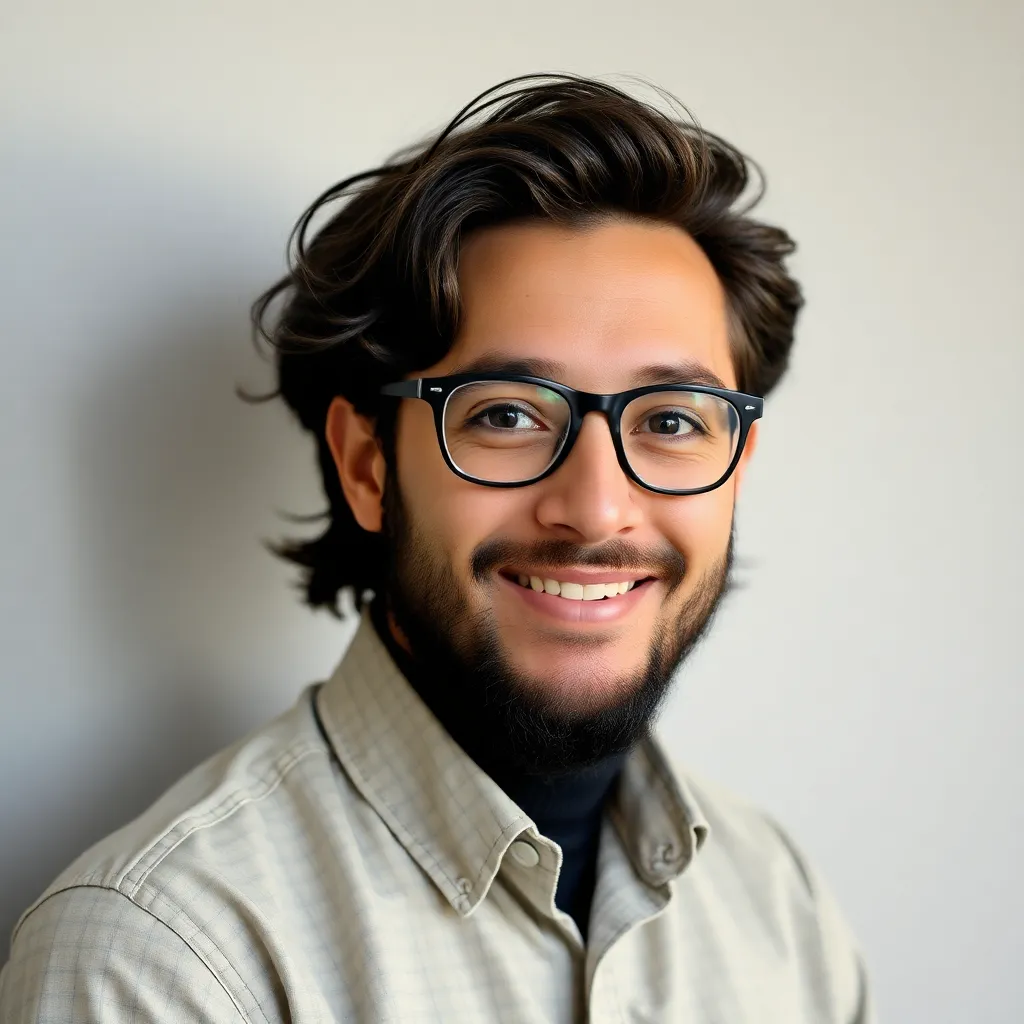
Onlines
Apr 05, 2025 · 6 min read
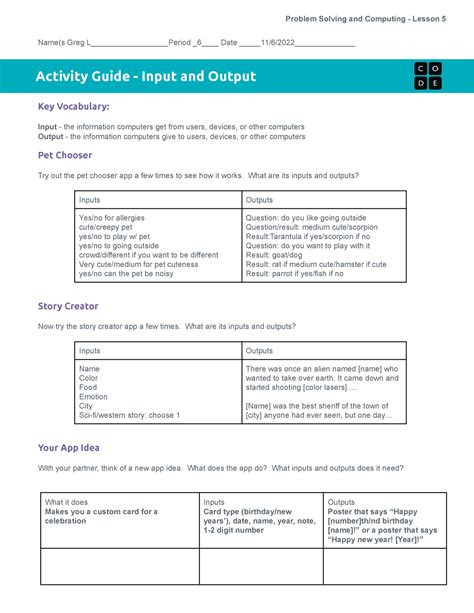
Table of Contents
Activity Guide: Input and Output – A Comprehensive Answer Key & Guide
This comprehensive guide delves into the fascinating world of input and output, a crucial concept in computer science and programming. We'll explore various activity guides, providing detailed answer keys and explanations to help you solidify your understanding. From basic input/output operations to more advanced techniques, this resource aims to be your ultimate companion for mastering this fundamental aspect of programming.
Understanding Input and Output
Before we dive into specific activities and their solutions, let's establish a firm grasp of the core concepts. Input refers to any data fed into a computer system, whether from a keyboard, mouse, sensor, file, or network connection. Output, conversely, is the data produced by the system as a result of processing the input. This output can be displayed on a screen, printed on paper, stored in a file, or transmitted over a network.
The interplay between input and output is the lifeblood of any interactive program. Without effective input mechanisms, a program cannot receive instructions or data. Without efficient output mechanisms, it cannot communicate its results.
Types of Input
Various methods exist for supplying input to a program:
- Keyboard Input: This is the most common form, allowing users to enter text, numbers, and commands directly.
- Mouse Input: Mice provide input in the form of clicks, drags, and movements, crucial for graphical user interfaces (GUIs).
- File Input: Programs can read data from files stored on the computer's storage devices.
- Network Input: Data can be received from other computers or devices over a network.
- Sensor Input: Sensors can provide input from the real world, like temperature, pressure, or light levels.
Types of Output
Similarly, programs utilize several methods to produce output:
- Screen Output: This displays information on the computer monitor, often the primary means of interaction.
- Printer Output: Hard copies of data can be generated using printers.
- File Output: Results can be saved to files for later use or analysis.
- Network Output: Data can be transmitted to other computers or devices over a network.
- Audio Output: Sounds and music can be generated and played.
Activity Guide 1: Basic Input/Output in Python
This activity focuses on fundamental input/output operations using Python.
Activity 1.1: Getting User Input
Write a Python program that asks the user for their name and age, then prints a greeting message incorporating this information.
Answer Key 1.1:
name = input("Enter your name: ")
age = input("Enter your age: ")
print(f"Hello, {name}! You are {age} years old.")
Activity 1.2: Calculating Area of a Rectangle
Write a Python program that takes the length and width of a rectangle as input from the user and calculates its area. Print the calculated area to the console.
Answer Key 1.2:
length = float(input("Enter the length of the rectangle: "))
width = float(input("Enter the width of the rectangle: "))
area = length * width
print(f"The area of the rectangle is: {area}")
Activity 1.3: File Input/Output
Write a Python program that writes a list of names to a file named "names.txt", then reads the names back from the file and prints them to the console.
Answer Key 1.3:
names = ["Alice", "Bob", "Charlie", "David"]
# Write names to the file
with open("names.txt", "w") as f:
for name in names:
f.write(name + "\n")
# Read names from the file
with open("names.txt", "r") as f:
for line in f:
print(line.strip())
Activity Guide 2: Input Validation and Error Handling
This section focuses on improving the robustness of your input/output programs by incorporating validation and error handling.
Activity 2.1: Age Validation
Modify Activity 1.1 to validate the user's age input. Ensure that the age is a positive integer. If the input is invalid, display an error message and prompt the user again.
Answer Key 2.1:
while True:
try:
age = int(input("Enter your age: "))
if age > 0:
break
else:
print("Age must be a positive integer.")
except ValueError:
print("Invalid input. Please enter a number.")
name = input("Enter your name: ")
print(f"Hello, {name}! You are {age} years old.")
Activity 2.2: File Existence Check
Modify Activity 1.3 to check if the "names.txt" file exists before attempting to read from it. If the file doesn't exist, display a message indicating this.
Answer Key 2.2:
import os
names = ["Alice", "Bob", "Charlie", "David"]
# Write names to the file
with open("names.txt", "w") as f:
for name in names:
f.write(name + "\n")
if os.path.exists("names.txt"):
with open("names.txt", "r") as f:
for line in f:
print(line.strip())
else:
print("The file 'names.txt' does not exist.")
Activity Guide 3: Advanced Input/Output Techniques
This section explores more advanced concepts in input and output.
Activity 3.1: Formatted Output
Write a Python program that takes a student's name, ID, and three exam scores as input. Output this information in a neatly formatted table to the console.
Answer Key 3.1:
name = input("Enter student name: ")
student_id = input("Enter student ID: ")
score1 = float(input("Enter score 1: "))
score2 = float(input("Enter score 2: "))
score3 = float(input("Enter score 3: "))
print("-" * 30)
print("{:<15} {:<10} {:<5}".format("Name", "ID", "Scores"))
print("-" * 30)
print("{:<15} {:<10} {:<5.2f} {:<5.2f} {:<5.2f}".format(name, student_id, score1, score2, score3))
print("-" * 30)
Activity 3.2: Working with CSV Files
Write a Python program that reads data from a CSV file containing student information (name, ID, scores) and calculates the average score for each student. Write the results (name, ID, average score) to a new CSV file.
Answer Key 3.2:
import csv
# Read from input CSV
with open('students.csv', 'r', newline='') as infile:
reader = csv.DictReader(infile)
student_data = list(reader)
#Process data
results = []
for student in student_data:
name = student['Name']
student_id = student['ID']
scores = [float(score) for score in [student['Score1'], student['Score2'], student['Score3']]]
average = sum(scores)/len(scores)
results.append({'Name': name, 'ID': student_id, 'Average Score': average})
# Write to output CSV
with open('results.csv', 'w', newline='') as outfile:
fieldnames = ['Name', 'ID', 'Average Score']
writer = csv.DictWriter(outfile, fieldnames=fieldnames)
writer.writeheader()
writer.writerows(results)
Note: For Activity 3.2, you'll need a students.csv
file with appropriate data. Create a CSV file with columns: Name, ID, Score1, Score2, Score3.
Conclusion
This guide has provided a comprehensive overview of input and output operations, ranging from basic concepts to more advanced techniques. By working through these activities and understanding the provided answer keys, you'll gain a solid foundation in handling input and output in your programs. Remember, consistent practice is key to mastering these essential skills. Continue exploring and experimenting with different input and output methods to further enhance your programming abilities. As you progress, you'll find yourself tackling increasingly complex problems with greater efficiency and confidence. The ability to effectively manage input and output is not just a technical skill; it's a fundamental aspect of creating user-friendly and efficient software applications.
Latest Posts
Latest Posts
-
Blueprint Reading For Welders 9th Edition Pdf Free
Apr 06, 2025
-
How Much Land Does A Man Need Summary
Apr 06, 2025
-
Aide Care For A Conscious Patient Should Be Preceded By
Apr 06, 2025
-
Summary Of Chapter 2 To Kill A Mockingbird
Apr 06, 2025
-
In Music What Does Allegro Mean Answer Key
Apr 06, 2025
Related Post
Thank you for visiting our website which covers about Activity Guide Input And Output Answer Key . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.