All Programming Languages Support Four Broad Data Types
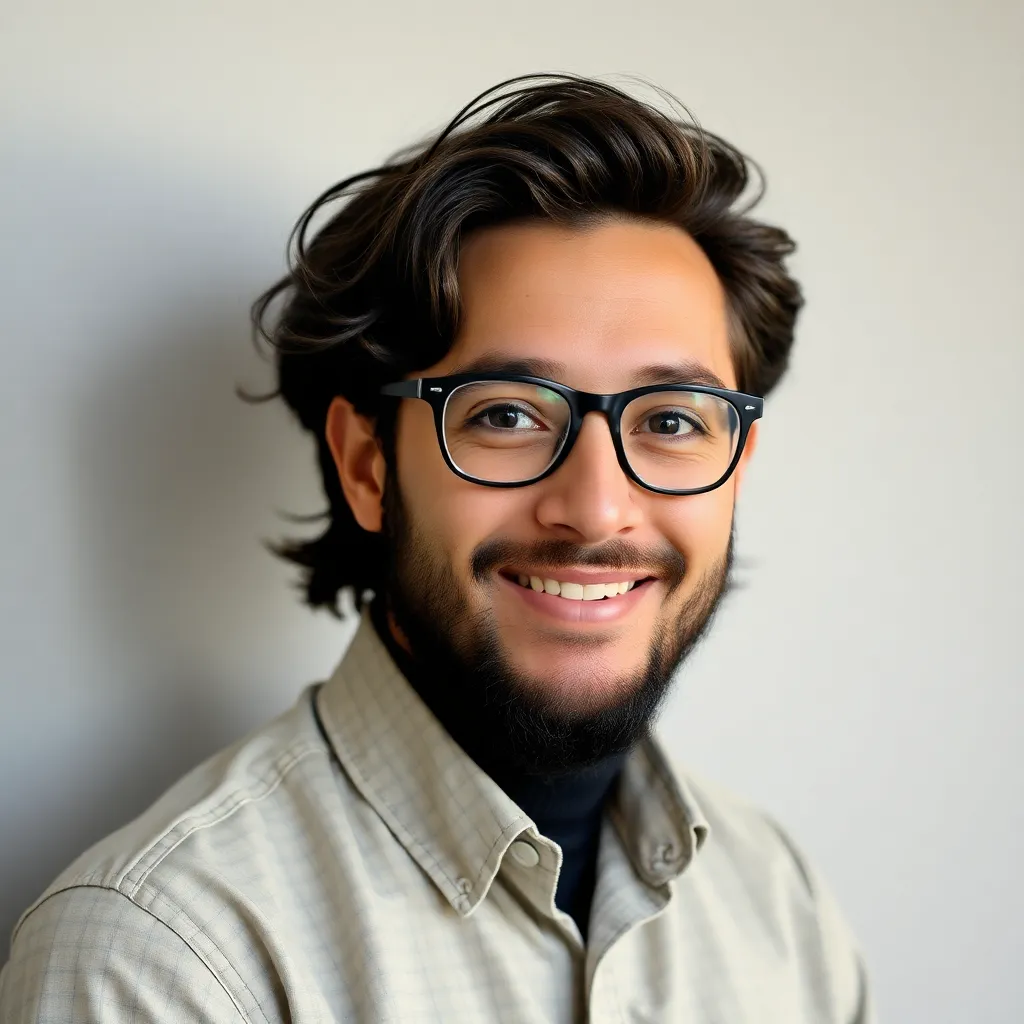
Onlines
May 11, 2025 · 6 min read
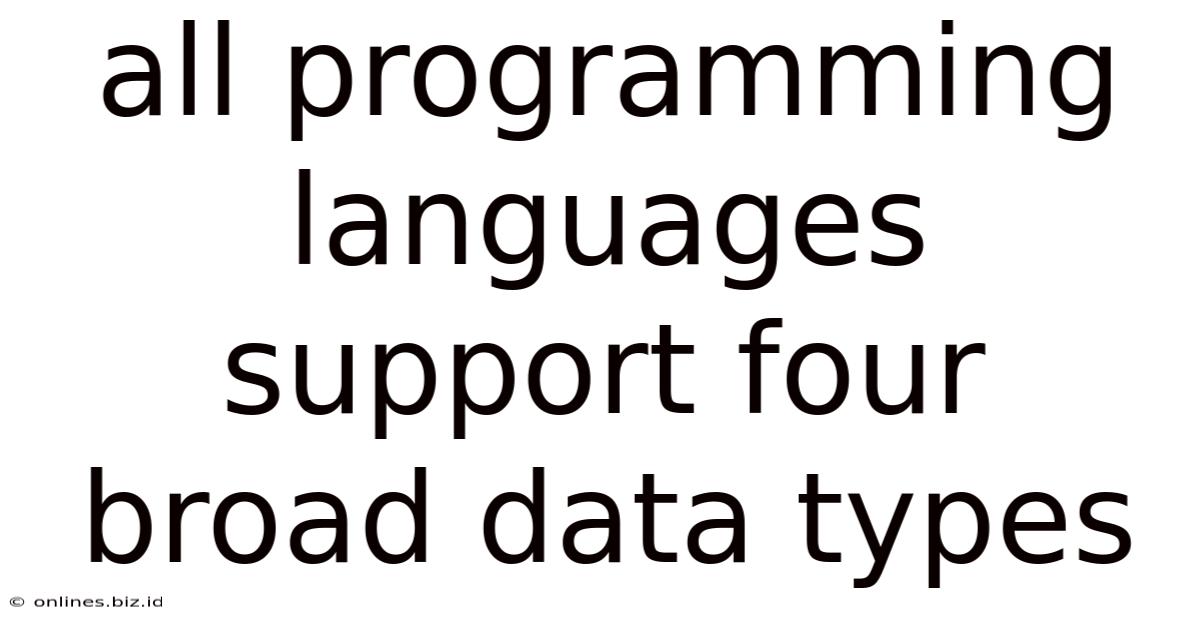
Table of Contents
All Programming Languages Support Four Broad Data Types: A Deep Dive
The world of programming is vast and diverse, with countless languages vying for attention. From the elegant simplicity of Python to the raw power of C++, each language boasts unique features and strengths. However, despite this apparent diversity, a fundamental unity underlies all programming languages: the support for four broad data types. These foundational types – integers, floating-point numbers, characters, and booleans – form the building blocks upon which all other data structures and complexities are built. While the specific implementation and nuances may differ between languages, the core concepts remain remarkably consistent. This article will delve deep into these four fundamental data types, exploring their properties, uses, and variations across different programming paradigms.
The Four Pillars: Understanding the Fundamental Data Types
Before we explore the specifics of each type, let's establish a common understanding. These four data types represent different ways a computer stores and manipulates information. They are the essential tools a programmer uses to represent the world within the confines of a digital environment. Understanding their characteristics is crucial for writing efficient, effective, and bug-free code.
1. Integers (int): The Whole Numbers
Integers represent whole numbers, both positive and negative, without any fractional component. These are fundamental for counting, indexing, and representing quantities where fractional values are not relevant. In many languages, the size of an integer is limited by the system's architecture (e.g., 32-bit or 64-bit), determining the range of values it can hold. Exceeding this range results in an integer overflow, leading to unexpected behavior.
Examples across languages:
- C/C++:
int x = 10;
- Java:
int x = 10;
- Python:
x = 10
(Python automatically handles integer size) - JavaScript:
let x = 10;
Variations: Many languages offer variations on the basic integer type to handle different ranges of values, such as short
, long
, long long
(C/C++), or byte
, short
, int
, long
(Java). These variations allow programmers to optimize memory usage and choose the most appropriate integer type for a given task. Unsigned integers, which only represent non-negative values, are also common.
Use Cases: Integers are ubiquitous in programming. They are used for:
- Loop counters: Controlling the number of iterations in loops.
- Array indexing: Accessing elements within arrays or lists.
- Representing quantities: Storing and manipulating numerical data like age, population, or scores.
2. Floating-Point Numbers (float/double): The Real Numbers
Floating-point numbers represent real numbers, including fractional values. They are essential for applications involving scientific computation, graphics, and any situation requiring decimal precision. These numbers are stored using a format that separates the mantissa (the significant digits) and the exponent (the power of 10). This representation allows for a wider range of values than integers, but introduces limitations in precision. Floating-point arithmetic can also suffer from rounding errors, which programmers need to be mindful of.
Examples across languages:
- C/C++:
float x = 3.14;
double y = 2.71828;
(double provides higher precision) - Java:
float x = 3.14f;
double y = 2.71828;
(the 'f' suffix is crucial in Java) - Python:
x = 3.14
(Python automatically handles floating-point numbers) - JavaScript:
let x = 3.14;
Variations: Similar to integers, many languages provide different floating-point types to control precision and memory usage. The float
type generally uses 32 bits, while double
uses 64 bits, offering greater precision.
Use Cases: Floating-point numbers are indispensable in:
- Scientific computing: Simulations, modeling, and mathematical calculations.
- Graphics programming: Representing coordinates, colors, and other visual elements.
- Financial applications: Handling monetary values and calculations involving decimals.
3. Characters (char): The Building Blocks of Text
Characters represent single letters, numbers, symbols, or other characters within a character set (like ASCII or Unicode). They form the basis for strings and text manipulation. The specific encoding (ASCII, UTF-8, etc.) determines how characters are represented in memory.
Examples across languages:
- C/C++:
char c = 'A';
- Java:
char c = 'A';
- Python:
c = 'A'
- JavaScript:
let c = 'A';
Variations: The size of a character type varies depending on the character encoding used. Unicode, for instance, requires more bits per character than ASCII.
Use Cases: Characters are critical for:
- String manipulation: Building and processing text.
- Input/output: Reading and writing textual data.
- Symbolic representations: Using characters to represent states or identifiers.
4. Booleans (bool): The Truth Values
Boolean variables represent truth values: true or false. They are fundamental for controlling program flow, making decisions based on conditions, and representing logical states.
Examples across languages:
- C/C++:
bool flag = true;
- Java:
boolean flag = true;
- Python:
flag = True
- JavaScript:
let flag = true;
Variations: While the basic concept is consistent across languages, the specific representation (e.g., 0 for false, 1 for true in some contexts) may vary slightly.
Use Cases: Booleans are essential for:
- Conditional statements: Controlling the execution of code blocks based on conditions (
if
,else if
,else
). - Logical operations: Performing logical AND, OR, and NOT operations.
- Flags and indicators: Representing the status of variables or processes.
Beyond the Four Fundamentals: Derived Data Types
While the four basic data types are the foundation, most programming languages offer a rich set of derived data types built upon these primitives. These include:
- Strings: Sequences of characters. Often implemented as arrays of characters.
- Arrays: Ordered collections of elements of the same type.
- Lists: Ordered collections of elements that can be of different types (common in Python).
- Sets: Unordered collections of unique elements.
- Dictionaries/Maps: Collections of key-value pairs, allowing for efficient data lookup.
- Structures/Classes: User-defined data types that group together related data and functions.
These derived types leverage the fundamental data types to create more complex and powerful ways to represent and manipulate information. Understanding the underlying primitives is key to grasping how these more sophisticated types function.
Language-Specific Nuances: A Glimpse into Diversity
While the four fundamental data types are universally present, their specific implementation and behavior can vary considerably across programming languages.
-
Type Systems: Languages differ in their type systems. Some are statically typed, requiring explicit type declarations, while others are dynamically typed, inferring types at runtime. Static typing generally leads to better error detection during compilation, while dynamic typing offers greater flexibility.
-
Integer Sizes: The range of values representable by integer types depends on the system's architecture and the language's implementation.
-
Floating-Point Precision: The precision of floating-point numbers varies, influencing the accuracy of calculations. Different languages may utilize different floating-point standards.
-
Character Encoding: The character set used (ASCII, UTF-8, etc.) affects how characters are represented and handled.
Conclusion: The Enduring Significance of Fundamental Data Types
Despite the vast landscape of programming languages and their diverse features, the four fundamental data types – integers, floating-point numbers, characters, and booleans – remain the bedrock of all computation. Understanding their properties, limitations, and variations across different languages is crucial for any aspiring programmer. They are not merely abstract concepts; they are the tangible building blocks with which we construct the digital world. Proficiency in working with these fundamental types is the key to unlocking the potential of any programming language and building robust, efficient, and effective software. Mastering them unlocks the power to create complex programs and systems, driving innovation and progress in the ever-evolving field of computer science.
Latest Posts
Latest Posts
-
Cesare Beccarias Essay On Crimes And Punishments Emphasized
May 12, 2025
-
Las Etapas De La Vida Leccion 9
May 12, 2025
-
What Can Insurance Protect You From Everfi
May 12, 2025
-
Identify The True Statement About The Head Of The Ulna
May 12, 2025
-
Intermediate States Can Be Created By
May 12, 2025
Related Post
Thank you for visiting our website which covers about All Programming Languages Support Four Broad Data Types . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.