Ap Cs A Unit 5 Progess Check
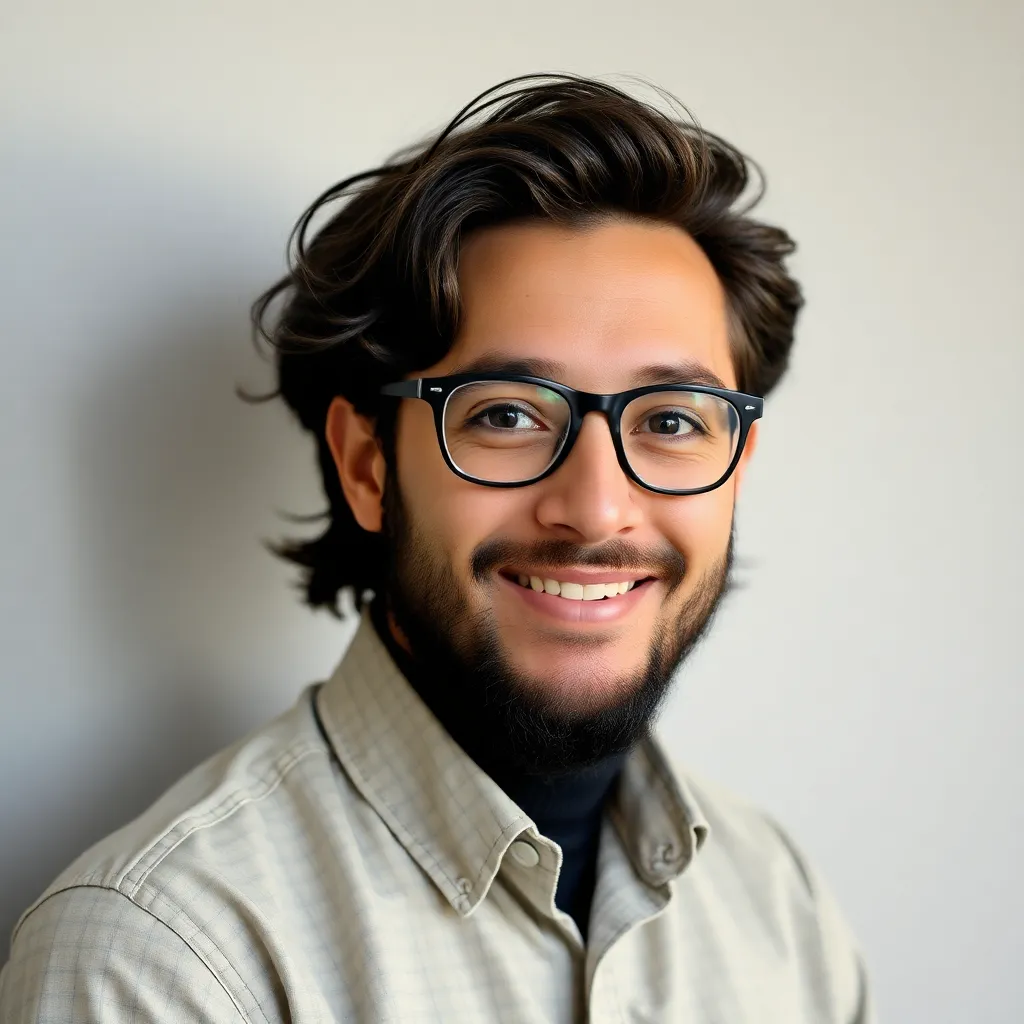
Onlines
Mar 03, 2025 · 7 min read
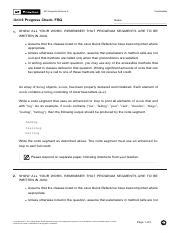
Table of Contents
AP CS A Unit 5 Progress Check: A Comprehensive Guide
The AP Computer Science A Unit 5 Progress Check is a significant milestone for students preparing for the AP exam. This unit focuses on object-oriented programming, a cornerstone of modern software development. Successfully navigating this progress check requires a thorough understanding of key concepts and the ability to apply them to diverse programming problems. This guide provides a comprehensive overview of Unit 5, covering essential topics, common challenges, and effective strategies for mastering the material.
Understanding the Core Concepts of AP CS A Unit 5
Unit 5 builds upon previous units, introducing more advanced object-oriented programming techniques. The core concepts you'll need to grasp include:
1. Classes and Objects
- Classes: Blueprints for creating objects. They define the data (instance variables) and behavior (methods) that objects of that class will possess. Think of a class as a template.
- Objects: Instances of a class. They are the actual entities created based on the class blueprint. Consider an object as a specific realization of the template.
Example: A Dog
class might have instance variables like name
, breed
, and age
, and methods like bark()
, fetch()
, and eat()
. Each individual dog you create would be an object of the Dog
class.
2. Instance Variables and Methods
- Instance Variables: Data associated with individual objects. They store the state of an object. Each object has its own copy of instance variables.
- Methods: Functions defined within a class that operate on the object's data. They define the object's behavior.
Example: In our Dog
class, name
, breed
, and age
are instance variables, while bark()
, fetch()
, and eat()
are methods.
3. Constructors
- Constructors: Special methods used to initialize the state of an object when it's created. They have the same name as the class and are automatically called when a new object is instantiated. Constructors ensure objects are created in a valid state.
Example: The Dog
class constructor might take name
and breed
as arguments and initialize the instance variables accordingly.
4. Access Modifiers (public, private)
- Access Modifiers: Control the visibility and accessibility of class members (instance variables and methods).
public
: Accessible from anywhere.private
: Accessible only within the class itself. Encapsulation, a crucial object-oriented principle, is achieved through private instance variables, controlled access through public methods (getters and setters).
Example: Instance variables representing a dog's internal health status might be private
while methods for getting and setting the dog's name would be public
.
5. Inheritance
- Inheritance: Creating new classes (subclasses) based on existing classes (superclasses). Subclasses inherit the properties and methods of their superclasses, allowing for code reuse and a hierarchical structure. This promotes extensibility and reduces code duplication.
Example: A GoldenRetriever
class could inherit from the Dog
class, inheriting all the properties and methods of the Dog
class, and adding its own specific characteristics.
6. Polymorphism
- Polymorphism: The ability of objects of different classes to respond to the same method call in their own specific way. This allows for flexibility and extensibility.
Example: Both GoldenRetriever
and Poodle
(both subclasses of Dog
) can respond to the bark()
method, but each might implement the method differently (different bark sounds).
7. ArrayLists and Wrapper Classes
- ArrayLists: Dynamic arrays that can grow or shrink as needed. Essential for storing collections of objects.
- Wrapper Classes: Classes that provide a way to treat primitive data types (like
int
,double
,boolean
) as objects. Necessary when working with collections that only accept objects.
Example: You might use an ArrayList<Dog>
to store a collection of Dog
objects. If you need to store integers in an ArrayList, you would use the Integer
wrapper class.
Common Challenges in Unit 5 and How to Overcome Them
Students often struggle with specific aspects of Unit 5. Here's a breakdown of common challenges and strategies for overcoming them:
1. Understanding the Relationship Between Classes and Objects
Many students initially confuse classes and objects. Remember, a class is a blueprint, while an object is a specific instance created from that blueprint. Visualizing this distinction using real-world analogies can be helpful.
2. Mastering Access Modifiers
Proper use of access modifiers (public
and private
) is crucial for encapsulation and data security. Practice designing classes with appropriate access levels for instance variables and methods. Use private instance variables and public getter/setter methods for controlled access.
3. Implementing Inheritance Correctly
Inheritance can be complex. Focus on understanding the "is-a" relationship. A subclass should be a specialized type of its superclass. Incorrect inheritance leads to design problems. Practice creating inheritance hierarchies with multiple levels of inheritance.
4. Utilizing Polymorphism Effectively
Polymorphism requires careful consideration of method overriding and virtual methods. Understand how different subclasses can respond differently to the same method call. Practice implementing polymorphic behavior in your code.
5. Working with ArrayLists and Wrapper Classes
Familiarize yourself with ArrayList methods (add, remove, get, size, etc.). Understand the necessity of wrapper classes when working with primitive data types in collections. Practice using ArrayLists to store and manipulate objects.
Strategies for Success on the AP CS A Unit 5 Progress Check
Effective preparation is key to succeeding on the progress check. Here are some proven strategies:
1. Active Learning
Don't just passively read the textbook or lecture notes. Actively engage with the material by writing your own code examples, working through practice problems, and experimenting with different approaches.
2. Practice, Practice, Practice
The more you practice, the better you'll become at understanding and applying the concepts. Work through as many practice problems as you can find. Focus on problems that test your understanding of inheritance and polymorphism.
3. Seek Help When Needed
Don't hesitate to ask for help from your teacher, classmates, or online resources if you're struggling with a concept. Collaborating with others can be a very effective way to learn.
4. Break Down Complex Problems
When faced with a complex programming problem, break it down into smaller, more manageable sub-problems. This will make the problem easier to approach and solve.
5. Code Reviews
If possible, have a classmate or teacher review your code. This can help identify potential errors or areas for improvement in your code style and problem-solving approach.
6. Understand the Big Picture
While mastering the details is crucial, it's equally important to understand the overall goals and principles of object-oriented programming. This will give you a more robust framework for your programming endeavors.
Example Problems and Solutions to Illustrate Key Concepts
Let's explore a few example problems that demonstrate the concepts covered in Unit 5:
Problem 1: Designing a BankAccount
Class
Design a BankAccount
class with instance variables for account number, balance, and owner name. Include methods for depositing, withdrawing, and checking the balance. Use access modifiers appropriately to protect the data.
Solution:
public class BankAccount {
private int accountNumber;
private double balance;
private String ownerName;
public BankAccount(int accountNumber, String ownerName) {
this.accountNumber = accountNumber;
this.ownerName = ownerName;
this.balance = 0.0; // Initial balance
}
public void deposit(double amount) {
balance += amount;
}
public void withdraw(double amount) {
if (balance >= amount) {
balance -= amount;
} else {
System.out.println("Insufficient funds.");
}
}
public double getBalance() {
return balance;
}
public String getOwnerName() {
return ownerName;
}
public int getAccountNumber() {
return accountNumber;
}
}
Problem 2: Inheritance with a SavingsAccount
Class
Create a SavingsAccount
class that inherits from the BankAccount
class. Add an instance variable for the interest rate. Override the deposit
method to add interest to the deposit.
Solution:
public class SavingsAccount extends BankAccount {
private double interestRate;
public SavingsAccount(int accountNumber, String ownerName, double interestRate) {
super(accountNumber, ownerName);
this.interestRate = interestRate;
}
@Override
public void deposit(double amount) {
double interest = amount * interestRate;
super.deposit(amount + interest);
}
}
Problem 3: Polymorphism with Different Account Types
Create a CheckingAccount
class that also inherits from BankAccount
. Demonstrate polymorphism by creating an array of BankAccount
objects containing both SavingsAccount
and CheckingAccount
objects.
Solution:
public class CheckingAccount extends BankAccount {
public CheckingAccount(int accountNumber, String ownerName) {
super(accountNumber, ownerName);
}
//Add any checking account specific methods here if needed.
}
public class Main {
public static void main(String[] args) {
BankAccount[] accounts = new BankAccount[2];
accounts[0] = new SavingsAccount(1234, "John Doe", 0.05);
accounts[1] = new CheckingAccount(5678, "Jane Smith");
for (BankAccount account : accounts) {
account.deposit(1000);
System.out.println(account.getBalance());
}
}
}
These examples highlight the importance of understanding classes, objects, inheritance, and polymorphism. By practicing with these types of problems, you will build a strong foundation for success on the AP CS A Unit 5 Progress Check and the AP exam. Remember consistent practice and a deep understanding of core concepts are key to mastering object-oriented programming.
Latest Posts
Latest Posts
-
Claim Evidence Reasoning Cer Model Evaluating The Effect Of Mutation Answers
Mar 03, 2025
-
The Only Way We Can Save Her Cartoon Meaning
Mar 03, 2025
-
Life Of Pi Book Summary Of Each Chapter
Mar 03, 2025
-
Demand Management Is A Strategy For Implementing Category Management
Mar 03, 2025
-
I Am Malala Chapter By Chapter Summary
Mar 03, 2025
Related Post
Thank you for visiting our website which covers about Ap Cs A Unit 5 Progess Check . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.