Ap Csa Unit 9 Progress Check Frq
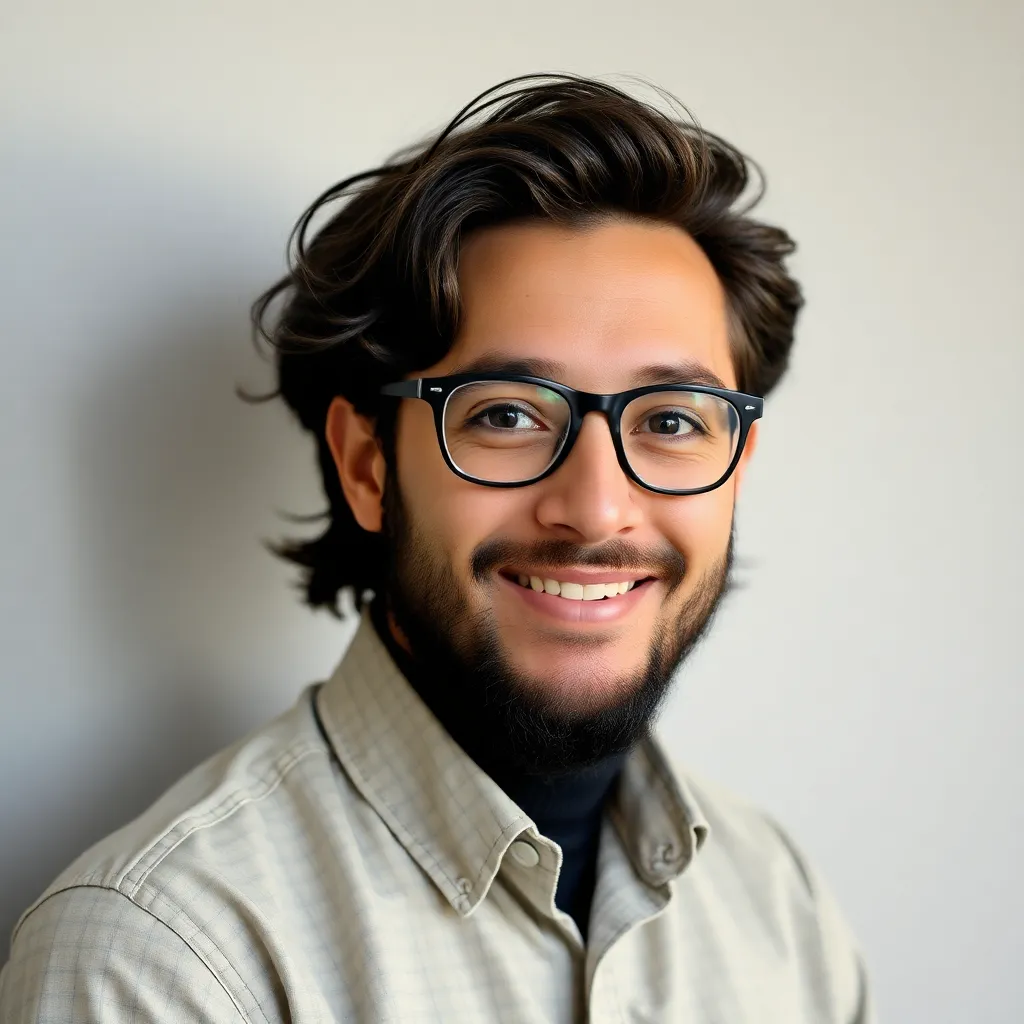
Onlines
Mar 29, 2025 · 7 min read
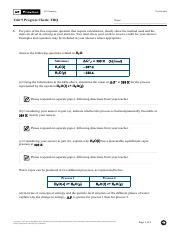
Table of Contents
AP CSA Unit 9 Progress Check: FRQ Deep Dive and Strategies for Success
Unit 9 of the AP Computer Science A curriculum delves into the fascinating world of object-oriented programming (OOP). This unit introduces crucial concepts like classes, objects, methods, and constructors, building upon the foundational programming principles you've learned thus far. The progress check, particularly the free-response questions (FRQs), often proves challenging for students. This comprehensive guide will dissect the typical FRQ structure, identify common pitfalls, and provide actionable strategies to master Unit 9 and ace the progress check.
Understanding the AP CSA Unit 9 FRQ Landscape
The Unit 9 FRQs typically involve designing and implementing classes, creating objects of those classes, and utilizing methods to manipulate data. Expect questions focusing on:
-
Class Design: You'll likely be asked to design a class from scratch, considering appropriate instance variables (attributes), constructors (to initialize objects), and methods (to perform actions). This necessitates a solid understanding of encapsulation, where data is protected within the class and accessed through methods.
-
Method Implementation: A significant portion of the FRQ will involve writing the code for methods. These methods might involve calculations, manipulating data within the class, or interacting with other objects. The quality of your code, its efficiency, and its correctness are heavily weighted.
-
Object Interaction: You'll frequently see questions involving multiple classes interacting with each other. This tests your understanding of how objects of different classes can collaborate to achieve a larger task. This involves proper use of object references and method calls.
-
Inheritance and Polymorphism (Advanced): While not always present, some FRQs might introduce inheritance (creating subclasses from superclasses) and polymorphism (using a single interface to represent multiple data types). These concepts are more advanced but are crucial for a higher score.
Common Mistakes to Avoid in Unit 9 FRQs
Many students stumble on seemingly simple aspects of class design and implementation. Here are some common mistakes to watch out for:
-
Incorrect Instance Variable Declaration: Forgetting the
private
keyword when declaring instance variables is a major error. This violates the principles of encapsulation and can lead to unexpected behavior. Remember,private
access modifiers restrict direct access to instance variables from outside the class, promoting data integrity. -
Faulty Constructor Implementation: A poorly written constructor fails to properly initialize the instance variables. This can lead to unpredictable results, especially when methods rely on those variables having specific initial values. Ensure your constructors correctly assign values to all instance variables.
-
Method Logic Errors: Incorrectly implementing method logic is a frequent source of point loss. Double-check your algorithms, conditions, loops, and return statements. Thoroughly test your code with various inputs to identify and correct errors.
-
Ignoring Method Specifications: Carefully read the problem statement and understand precisely what each method is expected to do. Failing to meet the method's specifications (e.g., returning the wrong data type, not handling edge cases) will result in a loss of points.
-
Lack of Comments: Clear comments explain your code's purpose and logic. Effective commenting significantly improves readability and allows graders to understand your thought process, even if your code contains minor errors.
-
Inappropriate Use of Static Members: Static variables and methods belong to the class itself, not to individual objects. Use them sparingly and only when appropriate (e.g., for counters or utility methods). Overuse can lead to incorrect object behavior.
-
Insufficient Testing: Testing your code thoroughly is crucial. Create multiple test cases that cover various scenarios, including edge cases and boundary conditions. This helps identify bugs early on and increases the likelihood of a correct solution.
Strategies for Conquering the Unit 9 FRQs
To excel in the Unit 9 FRQs, employ these strategies:
-
Master the Fundamentals: Before tackling the FRQs, ensure you have a firm grasp of the basic OOP concepts. Practice writing simple classes, constructors, and methods. Use online resources, textbooks, and practice problems to strengthen your understanding.
-
Break Down Complex Problems: If the FRQ seems daunting, break it down into smaller, manageable tasks. Focus on one method or part of the class at a time. This reduces the cognitive load and allows for more organized code development.
-
Plan Your Code Before Writing: Don't jump straight into coding. Start by sketching out the class structure, instance variables, and methods. Consider the relationships between different classes if the problem involves multiple classes. This planning step significantly improves code organization and reduces errors.
-
Use Pseudocode: For complex methods, writing pseudocode (a high-level description of the algorithm) can help clarify your thinking before translating it into actual Java code.
-
Test Incrementally: As you implement methods, test them individually. This helps pinpoint errors quickly and prevents cascading failures. Use a combination of manual testing (tracing code execution) and automated testing (using JUnit or similar frameworks) whenever feasible.
-
Review and Refactor: Once you've completed the FRQ, review your code carefully. Look for ways to improve its clarity, efficiency, and correctness. Refactoring (improving existing code without changing its functionality) is a crucial skill that demonstrates a deeper understanding of programming principles.
-
Practice, Practice, Practice: The key to mastering Unit 9 FRQs is consistent practice. Work through numerous practice problems, focusing on different aspects of OOP. The more you practice, the more comfortable and confident you'll become.
-
Seek Feedback: If possible, have someone review your code to identify potential areas for improvement. Constructive feedback from a teacher, classmate, or tutor can be invaluable.
Example FRQ and Solution Strategy
Let's analyze a hypothetical FRQ to illustrate the application of these strategies:
Hypothetical FRQ:
Design a class BankAccount
that represents a bank account. The class should have the following:
- Instance variables:
accountNumber
(String),balance
(double),ownerName
(String). - Constructor: A constructor that initializes all instance variables.
- Methods:
deposit(double amount)
: Deposits the specified amount into the account.withdraw(double amount)
: Withdraws the specified amount from the account. If the withdrawal amount exceeds the balance, print an error message and do not perform the withdrawal.getBalance()
: Returns the current balance of the account.toString()
: Returns a String representation of the account information (account number, owner name, balance).
Solution Strategy:
-
Plan: Start by outlining the class structure. Identify the instance variables and their data types. Sketch out the methods and their functionality.
-
Write the Code: Begin by implementing the constructor to initialize the instance variables. Then, implement each method one by one, focusing on clear, concise code. Remember to handle potential errors, such as attempting to withdraw more money than is available.
-
Test: Create several test cases to verify the functionality of each method. Test with various inputs, including edge cases such as zero deposits and withdrawals, and situations where withdrawal exceeds balance.
-
Refactor: Once the code is working, review it for clarity and efficiency. Are there any redundant parts? Can the code be made more readable?
Partial Code Example (Java):
public class BankAccount {
private String accountNumber;
private double balance;
private String ownerName;
public BankAccount(String accountNumber, String ownerName, double initialBalance) {
this.accountNumber = accountNumber;
this.ownerName = ownerName;
this.balance = initialBalance;
}
public void deposit(double amount) {
if (amount > 0) {
balance += amount;
} else {
System.out.println("Invalid deposit amount.");
}
}
public void withdraw(double amount) {
if (amount > 0 && amount <= balance) {
balance -= amount;
} else {
System.out.println("Insufficient funds or invalid withdrawal amount.");
}
}
public double getBalance() {
return balance;
}
public String toString() {
return "Account Number: " + accountNumber + ", Owner: " + ownerName + ", Balance: $" + balance;
}
}
This example demonstrates a basic implementation. A complete solution would require more robust error handling and thorough testing. Remember to add comprehensive comments to explain your code's logic.
Conclusion
Mastering Unit 9 of AP Computer Science A requires a deep understanding of object-oriented programming principles and consistent practice. By understanding the common pitfalls, employing effective strategies, and practicing diligently, you can significantly improve your performance on the progress check FRQs and build a strong foundation for future success in computer science. Remember that consistent effort and a methodical approach are key to achieving a high score.
Latest Posts
Related Post
Thank you for visiting our website which covers about Ap Csa Unit 9 Progress Check Frq . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.