C Is Trying To Determine Whether To Convert
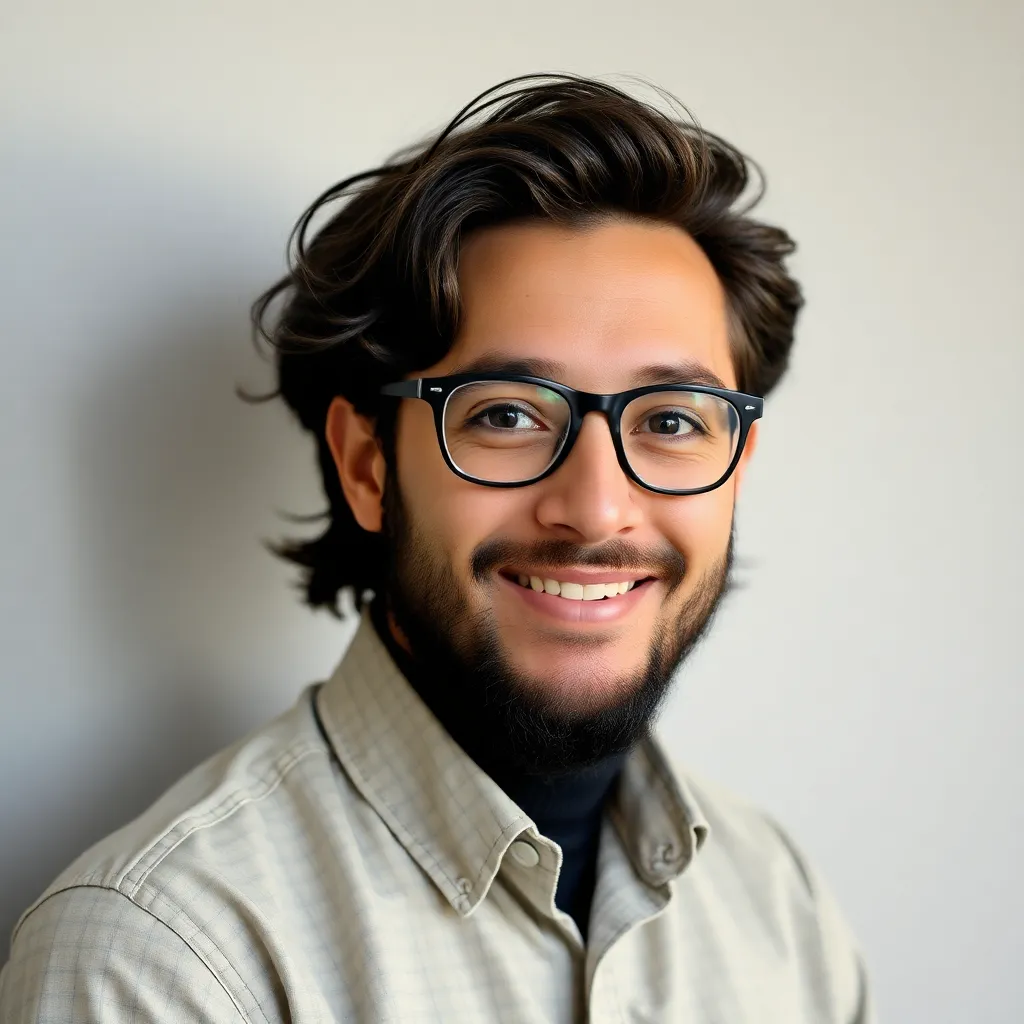
Onlines
Mar 29, 2025 · 6 min read
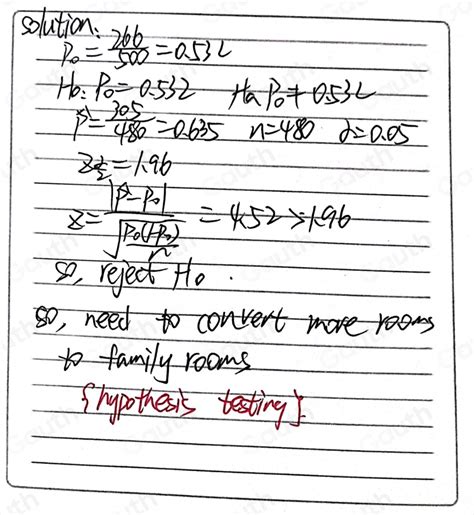
Table of Contents
C is Trying to Determine Whether to Convert: A Comprehensive Guide to Data Type Conversion
C programming, renowned for its efficiency and control over hardware, often requires meticulous management of data types. Converting between different data types—a process known as type casting or type conversion—is a fundamental aspect of C programming. However, the decision of whether or not to convert, and which conversion method to use, is crucial for avoiding errors and ensuring the reliability of your code. This comprehensive guide explores the complexities of data type conversion in C, providing a structured approach to making informed decisions about when and how to perform these conversions.
Understanding Data Types in C
Before diving into conversion, let's refresh our understanding of fundamental C data types. These types dictate how much memory a variable occupies and how the data within is interpreted. The primary types include:
int
: Stores integers (whole numbers). The size varies depending on the system (typically 4 bytes).float
: Stores single-precision floating-point numbers (numbers with decimal points). Typically 4 bytes.double
: Stores double-precision floating-point numbers, offering greater precision thanfloat
. Typically 8 bytes.char
: Stores a single character. Usually 1 byte.void
: Represents the absence of a type. Used for functions that don't return a value.
Implicit vs. Explicit Type Conversion
C offers two approaches to type conversion: implicit (automatic) and explicit (manual).
Implicit Type Conversion (Automatic Type Casting)
The compiler automatically performs implicit type conversion when an operation involves different data types. This usually happens during mixed-type arithmetic expressions. For instance:
int a = 5;
float b = 2.5;
float c = a + b; // Implicit conversion of 'a' to float before addition
In this example, the integer a
is implicitly converted to a float before adding it to the float b
. The result c
is a float. While convenient, implicit conversions can sometimes lead to unexpected results, especially when dealing with truncation (loss of fractional part) or potential overflow (exceeding the range of a data type).
Explicit Type Conversion (Casting)
Explicit type conversion, also called casting, gives the programmer complete control over the conversion process. It uses casting operators: (type)
placed before the variable or expression to be converted.
float x = 3.14;
int y = (int) x; // Explicit conversion of 'x' to int (truncation occurs)
Here, the float x
is explicitly cast to an integer y
. Note that the fractional part is truncated – it's not rounded. This is a crucial aspect to remember when performing explicit conversions.
Common Scenarios Requiring Type Conversion
Numerous situations demand careful consideration of type conversions in C:
1. Arithmetic Operations with Mixed Data Types
As previously illustrated, when arithmetic operations involve operands of different types, implicit conversion often occurs. Explicit casting provides greater precision and control. For example:
int num1 = 10;
double num2 = 3.14159;
double result = (double) num1 / num2; // Explicit casting avoids integer division
Casting num1
to double
before division ensures floating-point division, avoiding truncation that would occur with integer division.
2. Function Arguments and Return Values
Functions may expect specific data types as arguments or return values. Conversion might be necessary to ensure compatibility.
int myFunction(float arg) {
int result = (int) arg; // Convert float argument to int
return result;
}
This function takes a float
argument and explicitly converts it to an int
before returning the value.
3. Memory Manipulation and Pointers
Type conversion is crucial when working with pointers and memory addresses. For instance, converting a void
pointer (generic pointer) to a specific pointer type is essential for accessing the memory location correctly.
void *genericPtr;
int *intPtr = (int *) genericPtr; //Casting void pointer to int pointer. Use with extreme caution!
Caution: Incorrect pointer casting can lead to program crashes or unpredictable behavior. Always ensure you understand the memory layout and type of the data being pointed to.
4. Interfacing with External Libraries or Hardware
When interacting with external libraries or hardware devices, type conversions are often necessary to match the data types expected by those external components. Consult the documentation of the library or hardware to determine the correct types and conversions.
5. Character Manipulation
Converting between characters (char
) and integers (int
) is frequently needed. Characters are essentially represented as numerical values (their ASCII codes).
char myChar = 'A';
int myInt = (int) myChar; // Get the ASCII value of 'A'
char newChar = (char) 66; // Convert ASCII value 66 to character 'B'
Potential Pitfalls and Best Practices
Several potential problems can arise during type conversion:
- Data Loss: Converting from a type with higher precision (e.g.,
double
toint
) can lead to the loss of fractional parts or significant digits. - Overflow: Attempting to store a value that exceeds the range of the target type results in overflow. This can produce unpredictable or erroneous results.
- Underflow: The opposite of overflow, underflow occurs when a value is too small to be represented by the target type.
- Alignment Issues: Improper type conversion with pointers can lead to alignment problems, potentially causing memory access violations.
To minimize these risks, observe these best practices:
- Understand Type Sizes: Be aware of the sizes of different data types on your system. This helps prevent overflow and underflow.
- Use Explicit Casting Strategically: Employ explicit casting when necessary for clarity and to exert precise control over the conversion.
- Check for Errors: Include error-checking mechanisms to handle potential issues like overflow or data loss.
- Document Conversions: Clearly document all type conversions in your code to enhance readability and maintainability.
- Prioritize Safety: When dealing with pointers, exercise extreme caution and validate memory accesses rigorously.
Advanced Type Conversion Scenarios
Beyond the basics, certain scenarios demand a more nuanced approach to type conversion:
1. Using union
s for Type Punning
union
s allow storing different data types in the same memory location. While efficient, type punning (interpreting the same memory location as different types) should be approached cautiously due to potential alignment issues and compiler-specific behaviors.
2. Handling enum
Types
Enumerations (enum
) provide named integer constants. Conversions between enum
types and integers are often straightforward but require attention to potential range issues.
3. Custom Data Structures
When dealing with custom data structures (structs), you might need to convert between different struct types or between structs and fundamental types. Careful consideration of the memory layout is crucial.
Conclusion
Type conversion is a ubiquitous aspect of C programming that presents both powerful capabilities and potential pitfalls. By understanding the different types of conversions, their implications, and best practices, you can effectively manage data types, enhance code reliability, and prevent subtle bugs. Remember to use explicit casting thoughtfully, prioritize data integrity, and always consider the potential consequences of each conversion to ensure your C programs function correctly and efficiently. Thorough understanding and careful execution are key to mastering this critical aspect of C development.
Latest Posts
Latest Posts
-
The Services Mobilize Train Equip And Prepare Forces
Mar 31, 2025
-
The Aliens By Annie Baker Pdf
Mar 31, 2025
-
Life As A Hunter Answer Key
Mar 31, 2025
-
Color By Number Molecular Geometry And Polarity Answer Key
Mar 31, 2025
-
Unit 7 Polygons And Quadrilaterals Homework 2 Parallelograms Answer Key
Mar 31, 2025
Related Post
Thank you for visiting our website which covers about C Is Trying To Determine Whether To Convert . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.