Change The Width Of The Columns C H To 14
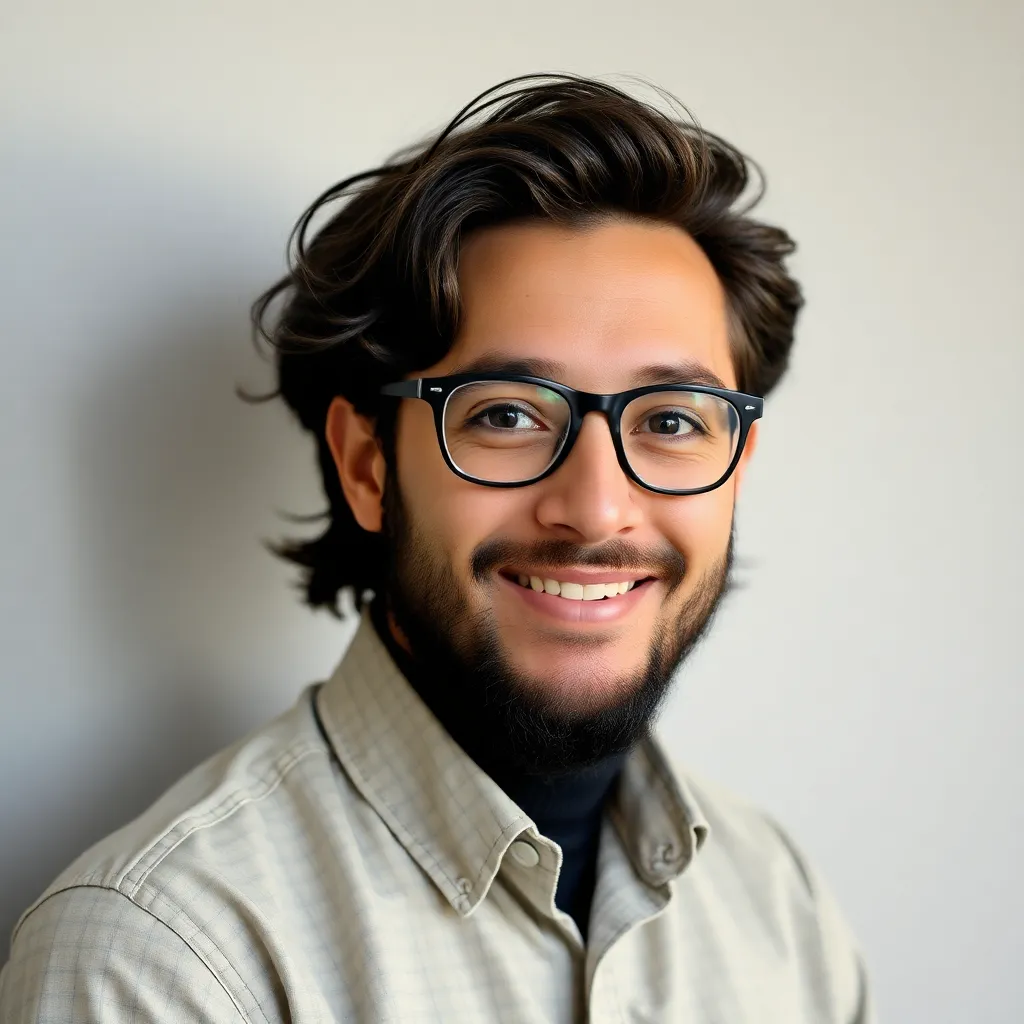
Onlines
May 12, 2025 · 6 min read
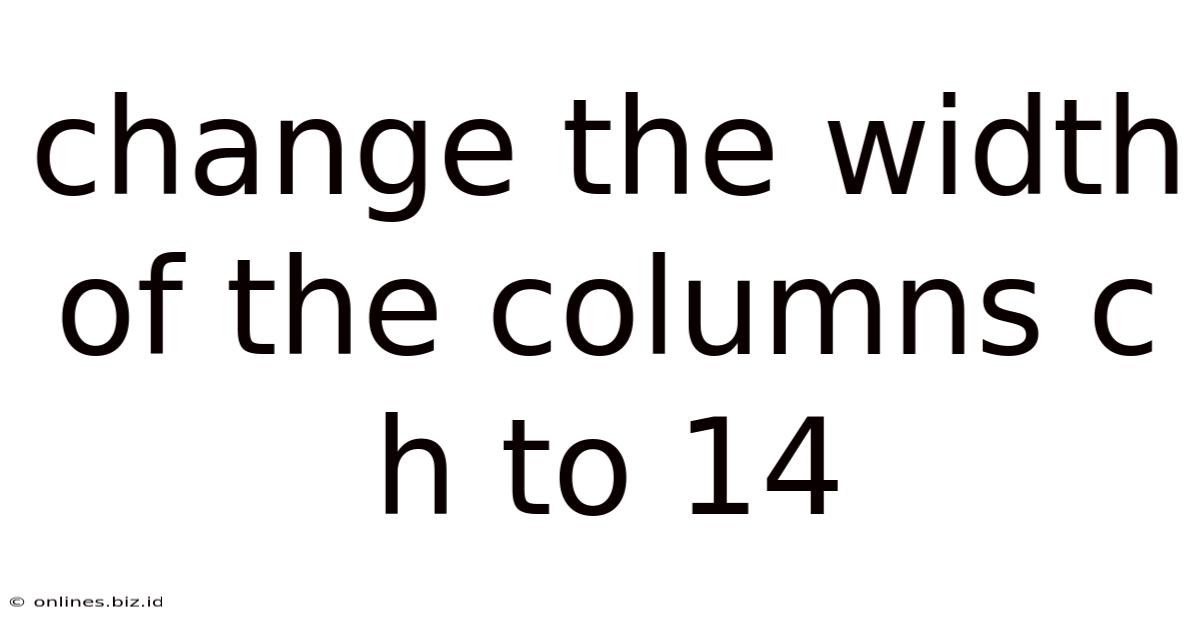
Table of Contents
Changing Column Widths in C#: A Comprehensive Guide
This article provides a thorough exploration of how to modify column widths in various C# contexts, focusing on achieving a column width of 14 units. We'll cover different scenarios, from manipulating data grids and list views to working with more advanced UI frameworks like WPF and WinForms. We'll also discuss best practices for ensuring your applications are both visually appealing and responsive to different screen sizes.
Understanding the Context: What are we changing?
Before diving into code, it's crucial to understand the context of "14 units." This value represents the desired width of a column, but the unit itself depends heavily on the environment:
- Pixels (px): A common unit representing physical screen pixels. This offers precise control but can lead to inconsistent appearance across different screen resolutions.
- Characters (ch): Approximately the width of the digit "0" in the current font. This approach provides relative sizing, adapting better to different fonts and resolutions. Targeting a width of
14ch
means the column should accommodate roughly 14 "0" characters. - Relative Units (e.g., %, em, rem): Percentages relative to the parent container,
em
(relative to the font size of the element), orrem
(relative to the root font size). These provide flexible and responsive layouts. - Grid Units (e.g., WPF, WinForms): In frameworks like WPF and WinForms, columns often use specific unit systems defined by the framework itself. These units might be independent of pixels or characters, offering abstraction and consistency within the framework.
Therefore, the approach to setting a column width to "14" will depend heavily on which unit "14" represents. We'll explore several common scenarios.
Modifying Column Width in Data Grids (WinForms)
WinForms applications frequently use DataGridView
controls for displaying tabular data. Changing column widths here involves directly accessing the column object and setting its Width
property.
// Assuming 'dataGridView1' is your DataGridView control
// and 'column1' is the column you want to modify.
dataGridView1.Columns["column1"].Width = 14; // Assuming '14' is in pixels.
// Or, to set it in characters (requires a bit more work):
// This example is illustrative and might need adjustment based on your font and system settings.
int charWidth = (int)dataGridView1.Font.SizeInPoints; // Approximate character width
dataGridView1.Columns["column1"].Width = 14 * charWidth;
//Alternatively, using a percentage width (this example uses an approximation to 14 characters - needs calibration)
int charWidth = (int)dataGridView1.Font.SizeInPoints; // Approximate character width
dataGridView1.Columns["column1"].Width = (int)(dataGridView1.Width * (14.0 * charWidth / dataGridView1.Width));
Remember to replace "column1"
with the actual name of your column and adjust the calculation of character width for better accuracy based on your application's font settings. Using character width offers more responsiveness than pure pixel values, but pixel values provide exact control.
Adjusting Column Width in ListViews (WinForms)
ListView
controls offer a different approach. While you can't directly set a width in "characters," you can manage the column width in pixels:
// Assuming 'listView1' is your ListView control and 'columnHeader1' is your column header.
listView1.Columns[0].Width = 14; // 14 pixels
The index [0]
refers to the column's position; replace it with the appropriate index for your target column. Similar to DataGridView
, pixel values provide precise but less responsive control.
Column Width Manipulation in WPF
WPF, being a more advanced framework, offers greater flexibility in layout control using DataGrid
or custom layouts. You can set column widths using various units.
Using DataGrid:
Here, Width="14*"
assigns a proportional width relative to the available space. The asterisk implies that the column takes 14 units of space in a star-sizing model. Consider using relative sizing (*
) for better responsiveness.
Using a custom layout (Grid):
This is a powerful, more low-level approach. The ColumnDefinition
with Width="14*"
creates a column that takes 14 proportional units of the available grid width.
Alternatively, you could use pixels:
Here, "14" will be interpreted as device-independent units (1/96th of an inch). While precise, this approach is less responsive to different screen sizes and resolutions than relative units.
For finer control, you could use Width="140"
and combine this with other UI elements to achieve a visual effect similar to 14 characters, given your specific font and style. This requires more calibration to your exact requirements.
Handling Different Screen Sizes and Responsiveness
The key to creating visually appealing applications lies in designing for adaptability. Avoid hardcoding pixel values whenever possible. Using proportional units like *
in WPF or percentages in WinForms allows columns to adjust to varying screen sizes and resolutions.
Consider employing media queries (if working with web-based C# applications or integrating with web technologies), to adjust column widths based on screen size dynamically.
Best Practices for Column Width Management
-
Prioritize User Experience: Choose units that enhance user experience (e.g., using character-based sizing for textual data or proportional sizing for consistent layouts).
-
Responsiveness: Employ relative units to ensure the application adapts effectively across devices with differing screen sizes.
-
Consistency: Maintain consistent sizing across similar columns within your application.
-
Testing: Thoroughly test your application across various screen resolutions and devices to identify any potential layout issues.
-
Accessibility: Ensure your column widths are sufficiently large for easy readability and accessibility compliance. Don't make columns unnecessarily narrow.
Advanced Techniques and Considerations
For complex scenarios, you might need to implement custom logic to handle column width adjustments dynamically. This could involve event handlers that respond to resizing events or even more sophisticated algorithms that calculate optimal column widths based on content and available space. Libraries like MeasureString
(WinForms) can assist in calculating the necessary width for text content to avoid truncation.
If working with databases or external data sources, you might need to consider the data itself when setting column widths. For instance, you could analyze the maximum length of strings in a particular column and use this information to set a suitable width.
Conclusion
Modifying column widths in C# requires adapting your approach to the specific UI framework and the desired unit of measurement. While precise pixel-based control offers accuracy, prioritizing responsive design with relative units like *
(WPF) or percentage-based sizes (WinForms) results in a more user-friendly experience. By understanding the nuances of different unit systems and employing the best practices outlined above, you can create C# applications with robust and adaptable column layouts. Remember that testing across different devices and screen sizes is crucial for ensuring a positive user experience. Finally, consider leveraging advanced techniques for dynamic adjustment and handling content variation for the most robust and efficient solutions.
Latest Posts
Latest Posts
-
Quincia Is Studying How The Lack Of
May 12, 2025
-
Interdependency Between Various Segments Of The Hospitality Industry Means
May 12, 2025
-
And Then There Were None Cliff Notes
May 12, 2025
-
Gummy Bear Dissection Lab Answer Key
May 12, 2025
-
Energy Control Programs Involve What Integral Steps
May 12, 2025
Related Post
Thank you for visiting our website which covers about Change The Width Of The Columns C H To 14 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.