Code Standards And Practices 4 Lesson 1
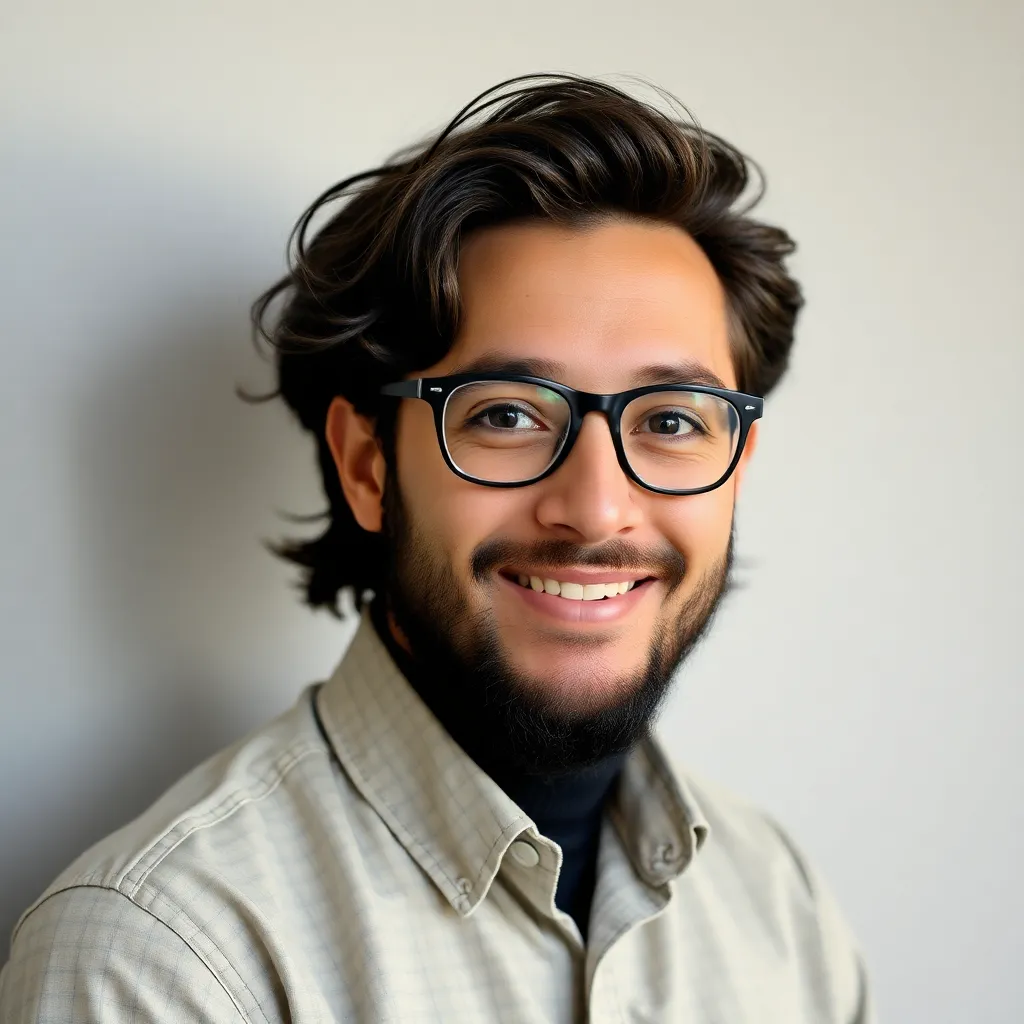
Onlines
Mar 23, 2025 · 6 min read
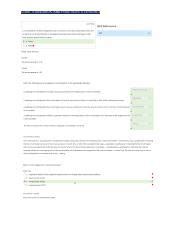
Table of Contents
Code Standards and Practices: Lesson 1 - Laying the Foundation for Clean, Maintainable Code
Clean, well-structured code isn't just aesthetically pleasing; it's the cornerstone of successful software development. This first lesson in code standards and practices will lay the groundwork for writing code that's not only functional but also readable, maintainable, and scalable. We'll explore fundamental principles applicable across various programming languages, focusing on concepts that transcend specific syntax.
Why Code Standards Matter
Before diving into specific practices, let's understand the why. Why bother with strict adherence to code standards when the program seems to work perfectly fine? The answer lies in the long-term implications:
-
Maintainability: Imagine trying to debug or extend code written haphazardly by someone else (or even yourself six months later!). Consistent formatting, clear naming conventions, and well-documented code significantly reduce the time and effort required for maintenance and updates. This translates directly to cost savings and improved productivity.
-
Collaboration: In team-based projects, consistent coding styles ensure that everyone is on the same page. This prevents conflicts, improves collaboration efficiency, and reduces the likelihood of errors introduced due to inconsistent approaches. Effective teamwork relies on shared understanding and consistent processes.
-
Readability: Clean code is easy to understand. This is crucial for both collaboration and individual debugging. Code that's easy to read is also easier to review, ensuring higher quality and fewer bugs. Readability directly impacts the efficiency of the development process.
-
Scalability: Well-structured code is much easier to scale. As projects grow in complexity and size, adherence to standards simplifies the process of adding new features or migrating to new environments. Scalability is essential for the long-term success of any software project.
-
Reduced Bugs: Consistent coding practices and thorough documentation reduce the likelihood of introducing bugs. Clear code is less prone to errors during development and easier to identify and fix during testing and maintenance. Bug reduction means higher quality software and reduced costs associated with bug fixes.
Fundamental Principles of Good Code
Several core principles underpin effective code standards. These principles serve as a guideline for writing code that is clean, efficient, and maintainable:
1. Consistency is King
The most important principle is consistency. Choose a style guide (many are available online for various languages) and stick to it religiously. Consistent indentation, naming conventions, commenting styles, and formatting make a huge difference in code readability. Inconsistency leads to confusion and makes the code difficult to understand.
2. Meaningful Names
Use descriptive and meaningful names for variables, functions, classes, and modules. Avoid abbreviations unless they're widely understood within the context of your project. A well-chosen name immediately conveys the purpose of the code element. Names should clearly reflect the functionality or purpose. For instance, instead of x
, use customerID
or productPrice
.
3. Comments and Documentation
While self-documenting code is ideal, comments are crucial for explaining complex logic, clarifying non-obvious sections, or providing context. Comments should be concise, accurate, and up-to-date. Similarly, comprehensive project documentation (e.g., using tools like JSDoc or Sphinx) is vital for understanding the overall architecture and functionality of the codebase. Documentation bridges the gap between the code and the human understanding of its purpose.
4. Modularity and Abstraction
Break down complex tasks into smaller, more manageable modules or functions. This improves code organization, readability, and reusability. Abstraction hides unnecessary complexity, allowing developers to focus on the essential aspects of the code. Modular design promotes better code structure and maintainability. This means avoiding large, monolithic functions and instead breaking them down into smaller, more focused units.
5. Error Handling and Exception Management
Implement robust error handling mechanisms to gracefully manage exceptions and prevent program crashes. Use try-catch blocks (or equivalent constructs in other languages) to handle potential errors and provide informative error messages. Proper error handling ensures the stability and reliability of the software.
6. Code Reviews
Regular code reviews are essential for identifying potential problems and improving code quality. Having another developer review your code helps catch errors, inconsistencies, and potential improvements. Code reviews are an invaluable part of ensuring high-quality code.
Specific Code Standards and Practices
Let's delve into some specific examples of code standards and best practices, though these will vary slightly based on the programming language.
Indentation and Formatting
Consistent indentation is crucial for readability. Most languages recommend using spaces (typically 4) for indentation, rather than tabs. Maintain consistent line lengths (e.g., under 80 characters) to improve readability on different screen sizes.
# Good indentation
def calculate_area(length, width):
area = length * width
return area
# Bad indentation
def calculate_area(length, width):
area = length * width
return area
Naming Conventions
Adopt a consistent naming convention for variables, functions, and classes. Common conventions include:
- Camel Case:
myVariableName
- Snake Case:
my_variable_name
- Pascal Case:
MyVariableName
(often used for classes)
Commenting Styles
Use clear and concise comments to explain the purpose of code sections. Avoid redundant comments that simply restate the obvious.
// Calculate the area of a rectangle
public double calculateArea(double length, double width) {
return length * width;
}
Error Handling
Use try-catch
blocks (or equivalent) to handle potential exceptions gracefully and prevent unexpected crashes. Provide informative error messages to help with debugging.
try {
// Code that might throw an error
let result = 10 / 0;
} catch (error) {
console.error("An error occurred:", error);
}
Code Reviews Best Practices
- Establish a clear checklist: Define specific points to check during reviews, such as coding style compliance, security vulnerabilities, and functionality.
- Focus on both code quality and maintainability: Evaluate not only the functionality but also how easy it will be to maintain and modify the code in the future.
- Be respectful and constructive: Provide feedback in a positive and helpful manner, focusing on improving the code rather than criticizing the developer.
- Use a collaborative tool: Utilize code review tools to streamline the process and facilitate discussions.
Language-Specific Considerations
While the general principles remain consistent, specific standards and best practices vary across different programming languages. For example:
- Python: Emphasizes readability and uses a specific style guide (PEP 8).
- Java: Stricter syntax rules and a focus on object-oriented programming principles.
- JavaScript: Widely varying styles but increasing adoption of standardized frameworks and linters.
- C++: Focus on memory management and performance optimization, leading to more complex coding standards.
Tools and Resources
Several tools can help enforce code standards and improve code quality:
- Linters: Static code analysis tools that automatically detect potential issues such as style violations or bugs. (e.g., ESLint for JavaScript, Pylint for Python)
- Formatters: Automatically format code according to a specified style guide. (e.g., Prettier, Black)
- Static Analysis Tools: More advanced tools that can detect more complex issues like potential vulnerabilities.
Conclusion
Adhering to code standards and practices is not just a matter of aesthetics; it's a fundamental aspect of writing high-quality software. By consistently applying the principles and practices outlined in this lesson, developers can significantly improve code readability, maintainability, scalability, and reduce the likelihood of errors. Remember, clean code is a key ingredient for successful software projects. This first lesson provided a strong foundation; future lessons will explore more advanced techniques and best practices. Start incorporating these principles into your projects today, and experience the positive impact on your coding workflow and the quality of your software.
Latest Posts
Latest Posts
-
Unit 1 Geometry Basics Homework 2 Segment Addition Postulate
Mar 25, 2025
-
The Advertising Director For A Guitar Manufacturer
Mar 25, 2025
-
Time Values In Music Are Expressed In Absolute Terms
Mar 25, 2025
-
Answer Each Question Affirmatively Using The Correct Possessive Adjective
Mar 25, 2025
-
What Does Set And Coordinate Distribution Objectives Mean
Mar 25, 2025
Related Post
Thank you for visiting our website which covers about Code Standards And Practices 4 Lesson 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.