Complete The Doubledown Function To Return Twice The Initialvalue
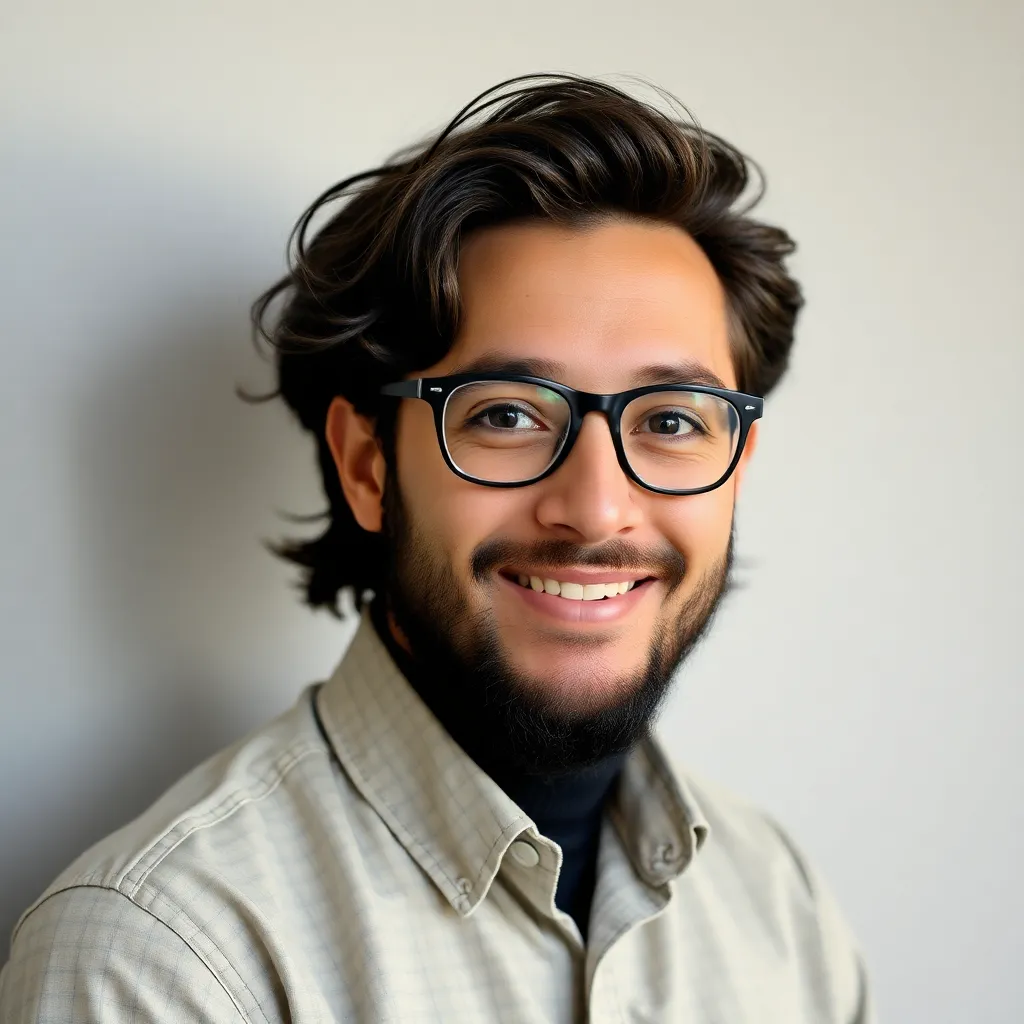
Onlines
May 10, 2025 · 5 min read
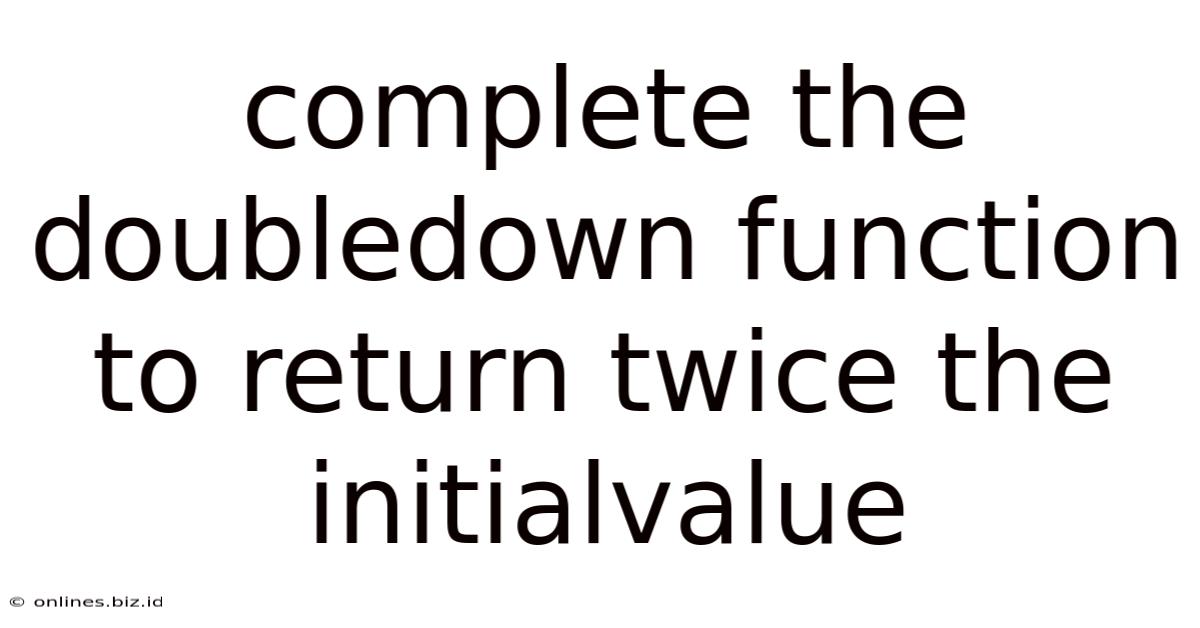
Table of Contents
Completing the DoubleDown Function: A Deep Dive into Doubling Initial Values
This comprehensive guide delves into the intricacies of a function designed to double an initial value. We'll explore various programming paradigms, implementation strategies, and potential challenges while focusing on the core functionality of doubling the input. This article aims to be a complete resource for understanding and implementing a robust "doubleDown" function.
Understanding the Core Functionality
At its heart, the doubleDown
function is remarkably simple: it takes a single input value and returns a value that is double the initial input. While seemingly straightforward, the nuances lie in how efficiently and reliably this doubling is achieved, handling various data types, and managing potential errors.
Data Type Considerations
The choice of programming language significantly impacts how we handle data types. Languages like Python offer dynamic typing, allowing flexibility in input types (integers, floats, etc.), while statically-typed languages like C++ or Java demand explicit type declarations.
Let's examine a few implementations:
Python (Dynamic Typing):
def doubleDown(initial_value):
"""Doubles the initial value. Handles integers and floats."""
try:
return 2 * initial_value
except TypeError:
return "Invalid input type. Please provide a number."
This Python implementation leverages the power of dynamic typing. The try-except
block gracefully handles potential TypeError
exceptions if the input is not a number.
C++ (Static Typing):
#include
template
T doubleDown(T initial_value) {
return 2 * initial_value;
}
int main() {
int num = 5;
double dec = 3.14;
std::cout << "Doubled integer: " << doubleDown(num) << std::endl;
std::cout << "Doubled double: " << doubleDown(dec) << std::endl;
return 0;
}
The C++ example uses a template function to handle various numeric types. This improves code reusability without sacrificing type safety. Error handling might require more elaborate mechanisms, such as checking for NaN
(Not a Number) or Inf
(Infinity) values.
JavaScript (Dynamic Typing):
function doubleDown(initialValue) {
if (typeof initialValue !== 'number') {
return "Invalid input: Please provide a number.";
}
return 2 * initialValue;
}
console.log(doubleDown(5)); // Output: 10
console.log(doubleDown("hello")); // Output: Invalid input: Please provide a number.
JavaScript, similar to Python, handles various numeric types dynamically. Explicit type checking improves robustness and prevents unexpected behavior.
Advanced Considerations and Error Handling
While the basic implementation is simple, robust error handling is crucial for production-ready code. Consider these scenarios:
- Non-numeric input: The function should gracefully handle cases where the input is not a number. This could involve returning an error message, raising an exception, or returning a default value (e.g., 0).
- Overflow errors: In languages with fixed-size integers, very large inputs might cause overflow. For example, if you double the largest possible integer, you exceed the maximum representable value. This can lead to unexpected behavior or program crashes. Solutions might involve using arbitrary-precision arithmetic libraries or checking input values against maximum limits.
- Null or undefined values: Some programming languages allow
null
orundefined
values. The function needs to explicitly handle these cases, perhaps by returning a default value or throwing an exception. - Floating-point precision: When working with floating-point numbers, remember that calculations might not be perfectly accurate due to limitations in how floating-point numbers are represented in computer memory. For example,
0.1 + 0.2
might not precisely equal0.3
. This can subtly affect the results of doubling a floating-point number.
Extending Functionality: Beyond Simple Doubling
The core concept can be expanded to create more versatile functions. Here are some possibilities:
- Multiplier parameter: Instead of hardcoding the multiplier as 2, you could make it a parameter, allowing the function to multiply by any desired value.
def multiplyValue(initial_value, multiplier):
"""Multiplies the initial value by a given multiplier."""
try:
return initial_value * multiplier
except TypeError:
return "Invalid input type. Please provide numbers."
-
Multiple input values: The function could be extended to accept multiple input values and return an array or list containing the doubled values.
-
Object-Oriented Approach: In object-oriented programming languages, you could create a class where the
doubleDown
function is a method. This allows for encapsulation of data and methods.
public class Doubler {
private double value;
public Doubler(double value) {
this.value = value;
}
public double doubleDown() {
return 2 * this.value;
}
}
Testing and Validation
Thorough testing is critical for ensuring the accuracy and reliability of your doubleDown
function. Unit tests are an excellent way to verify that the function works correctly for various inputs, including boundary conditions (e.g., zero, negative numbers, very large numbers), edge cases, and error scenarios. Consider using testing frameworks like pytest (Python), JUnit (Java), or Jest (JavaScript).
Optimizations and Performance
For most applications, the basic implementation of doubleDown
will be sufficiently efficient. However, for performance-critical scenarios involving a vast number of operations, consider these potential optimizations:
-
Bitwise operations (for integers): Multiplying by 2 is equivalent to a left bit shift operation (
<<
). This can be significantly faster than standard multiplication, especially for integer types. However, this optimization is only applicable to integers and not floating-point numbers. -
Vectorization (for numerical computing): If you're working with large arrays or matrices of numbers, consider using vectorized operations (e.g., NumPy in Python) to perform the doubling operation on all elements simultaneously. This can drastically improve performance compared to iterating through the array element by element.
Conclusion
The seemingly simple task of doubling an initial value can lead to a surprising depth of considerations. From robust error handling and data type management to performance optimizations and testing strategies, creating a production-ready doubleDown
function requires attention to detail and a comprehensive approach. This guide provides a strong foundation for understanding and implementing such functions across various programming languages, promoting code quality and efficiency. Remember to always prioritize clear, readable code and comprehensive testing to ensure your function operates reliably and predictably under diverse conditions.
Latest Posts
Latest Posts
-
Racism Tends To Intensify During Periods Of Economic Uncertainty
May 10, 2025
-
What Does Jefferson State Directly As The Reason
May 10, 2025
-
Apply The Preset 4 Picture Effect To The Picture
May 10, 2025
-
Chapter 4 Checking Accounts Lesson 4 3 Check Registers Answer Key
May 10, 2025
-
Stella Is Driving Down A Steep Hill
May 10, 2025
Related Post
Thank you for visiting our website which covers about Complete The Doubledown Function To Return Twice The Initialvalue . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.