Declare An Integer Variable Named Degreescelsius
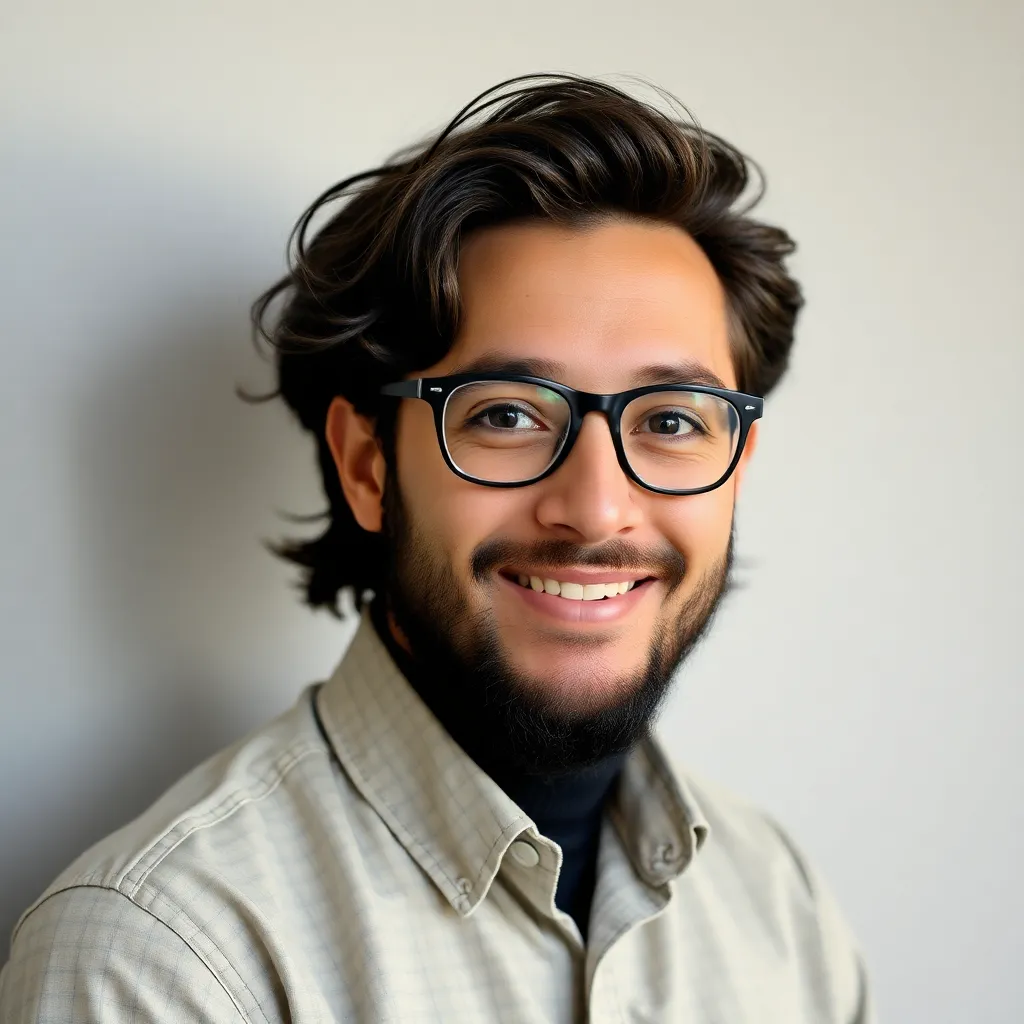
Onlines
May 06, 2025 · 6 min read
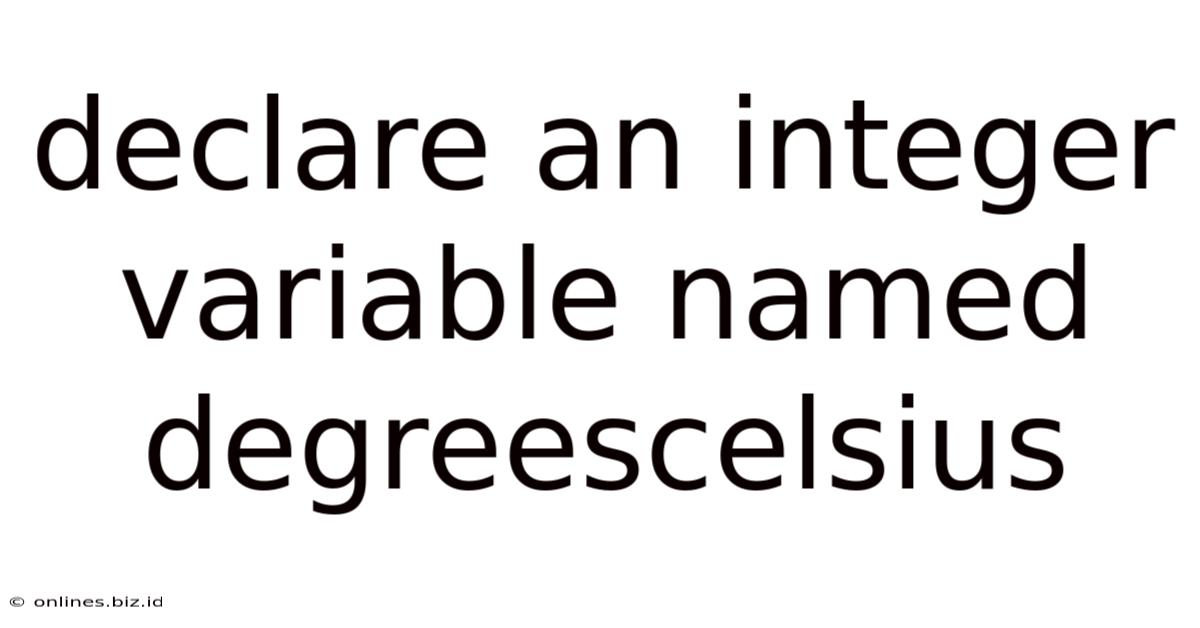
Table of Contents
- Declare An Integer Variable Named Degreescelsius
- Table of Contents
- Declaring an Integer Variable Named degreesCelsius: A Deep Dive into Data Types and Best Practices
- Understanding Integer Data Types
- Declaring degreesCelsius in Various Languages
- C++
- Java
- Python
- JavaScript
- C#
- Go
- Best Practices for Variable Naming
- Beyond Declaration: Variable Usage and Scope
- Error Handling and Data Validation
- Advanced Concepts and Related Topics
- Conclusion
- Latest Posts
- Related Post
Declaring an Integer Variable Named degreesCelsius
: A Deep Dive into Data Types and Best Practices
Declaring variables is a fundamental aspect of programming. This seemingly simple act – assigning a name and data type to a memory location – forms the bedrock of any program's logic and functionality. This article delves into the specifics of declaring an integer variable named degreesCelsius
, exploring the nuances of integer data types, best practices for variable naming, and the broader context of variable declaration within different programming languages.
Understanding Integer Data Types
Before diving into the declaration itself, let's clarify what an integer is in the context of programming. An integer is a whole number, positive or negative, without any fractional or decimal component. Examples include -3, 0, 10, 1000, and so on. Integer data types are crucial for representing quantities that can't be fractional, such as the number of items in a list, the count of events, or, in our case, a temperature measured in whole degrees Celsius.
Different programming languages offer variations on integer data types, often categorized by the range of values they can hold:
-
int
(or similar): A standard integer type, usually 32 bits (capable of holding values from roughly -2 billion to +2 billion). The exact range depends on the specific system and compiler. -
short
(orint16
): A shorter integer type, typically 16 bits, offering a smaller range but requiring less memory. -
long
(orint64
): A longer integer type, typically 64 bits, providing a much wider range of values suitable for very large numbers. -
unsigned int
: An integer type that can only hold non-negative values (0 and positive integers), effectively doubling the positive range compared to a signedint
.
The choice of which integer type to use depends on the expected range of values. For degreesCelsius
, a standard int
is usually sufficient, as temperatures are unlikely to exceed the typical range of an int
. However, for applications dealing with extremely high or low temperatures, a long
might be more appropriate.
Declaring degreesCelsius
in Various Languages
The syntax for declaring a variable, including its data type, varies across programming languages. Here are examples in some popular languages:
C++
int degreesCelsius;
This single line declares an integer variable named degreesCelsius
. No initial value is assigned; its value is undefined until it's explicitly set.
int degreesCelsius = 25; // Initializing the variable
This example declares and initializes degreesCelsius
to 25. Initialization is good practice, as it prevents accidental use of uninitialized variables.
Java
int degreesCelsius;
Similar to C++, this declares an integer variable without initialization.
int degreesCelsius = 0; // Initializing to 0
This initializes degreesCelsius
to 0. This is often preferred for numerical variables to establish a default value.
Python
Python is dynamically typed, meaning you don't explicitly declare the data type. The interpreter infers the type based on the assigned value.
degreesCelsius = 20
This assigns the integer value 20 to the variable degreesCelsius
. The interpreter understands this as an integer variable.
JavaScript
Like Python, JavaScript is dynamically typed.
let degreesCelsius = 15;
This declares a variable named degreesCelsius
using let
(allowing reassignment) and assigns it the integer value 15. You could also use const
if you intend the variable to remain constant after assignment.
C#
int degreesCelsius;
This declares an integer variable in C#.
int degreesCelsius = 30; // Initialization
This declares and initializes the variable.
Go
var degreesCelsius int
Go uses the var
keyword to declare variables. The type is specified after the variable name.
degreesCelsius := 27 // Short declaration, type is inferred.
Go also allows a short declaration syntax where the type is inferred from the assigned value.
Best Practices for Variable Naming
Choosing meaningful and consistent names for your variables is crucial for code readability and maintainability. The name degreesCelsius
adheres to several best practices:
-
Descriptive: The name clearly indicates the variable's purpose – storing a temperature in degrees Celsius.
-
Camel Case: The name uses camel case (capitalizing the first letter of each word except the first), a widely adopted convention. Alternatives include snake_case (using underscores) or PascalCase (capitalizing the first letter of every word). Consistency is key.
-
Avoid Abbreviations: Using full words improves understanding. Abbreviations can lead to ambiguity.
-
No Reserved Keywords: Avoid using language keywords (like
int
,for
,while
) as variable names. -
Context Specific: The name is suitable within the context of temperature measurement. A different application might require a different name.
Beyond Declaration: Variable Usage and Scope
Once declared, the degreesCelsius
variable can be used in various operations within your program. This might involve:
-
Assignment:
degreesCelsius = 35;
-
Arithmetic Operations:
fahrenheit = (degreesCelsius * 9/5) + 32;
-
Comparisons:
if (degreesCelsius > 25) { ... }
-
Input/Output: Reading the value from user input or displaying it on the screen.
The scope of a variable determines where in your program it's accessible. Variables declared within a function are typically only accessible within that function (local scope). Variables declared outside any function have global scope and are accessible from anywhere in the program. Careful management of scope is crucial for preventing naming conflicts and improving code organization.
Error Handling and Data Validation
When working with user input or external data sources, it's important to incorporate error handling and data validation to ensure the integrity of your program. For instance, if you're reading degreesCelsius
from user input, you should check if the input is a valid integer and falls within a reasonable temperature range.
while True:
try:
degreesCelsius = int(input("Enter temperature in degrees Celsius: "))
if -273 <= degreesCelsius <= 1000: #Example reasonable range
break
else:
print("Temperature out of reasonable range.")
except ValueError:
print("Invalid input. Please enter an integer.")
This Python code uses a while
loop and try-except
block to handle potential errors. It also adds a range check to ensure that input value is within a reasonable range. Similar error-handling mechanisms exist in other languages.
Advanced Concepts and Related Topics
Understanding the declaration of degreesCelsius
extends to more complex concepts:
-
Data Structures: Integers can be elements within arrays, lists, or other data structures.
-
Object-Oriented Programming (OOP): In OOP languages, integers can be attributes (member variables) of objects.
-
Type Safety: Statically typed languages (like C++, Java) perform type checking during compilation, helping catch errors before runtime. Dynamically typed languages (like Python, JavaScript) perform type checking during runtime.
-
Memory Management: Understanding how memory is allocated and managed for integer variables is crucial for optimizing performance, especially when dealing with large datasets.
-
Bitwise Operations: Integer variables can be manipulated at the bit level using bitwise operators (AND, OR, XOR, etc.).
Conclusion
Declaring an integer variable named degreesCelsius
, though seemingly simple, touches upon fundamental aspects of programming. Choosing appropriate data types, adhering to best practices for variable naming, and incorporating error handling and data validation are essential for creating robust, maintainable, and readable code. This understanding serves as a foundation for building more complex programs and mastering advanced programming concepts. Remember that clarity, consistency, and a focus on good programming practices are vital for success in software development.
Latest Posts
Related Post
Thank you for visiting our website which covers about Declare An Integer Variable Named Degreescelsius . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.