Declaring A Class Does Not Create Actual Objects
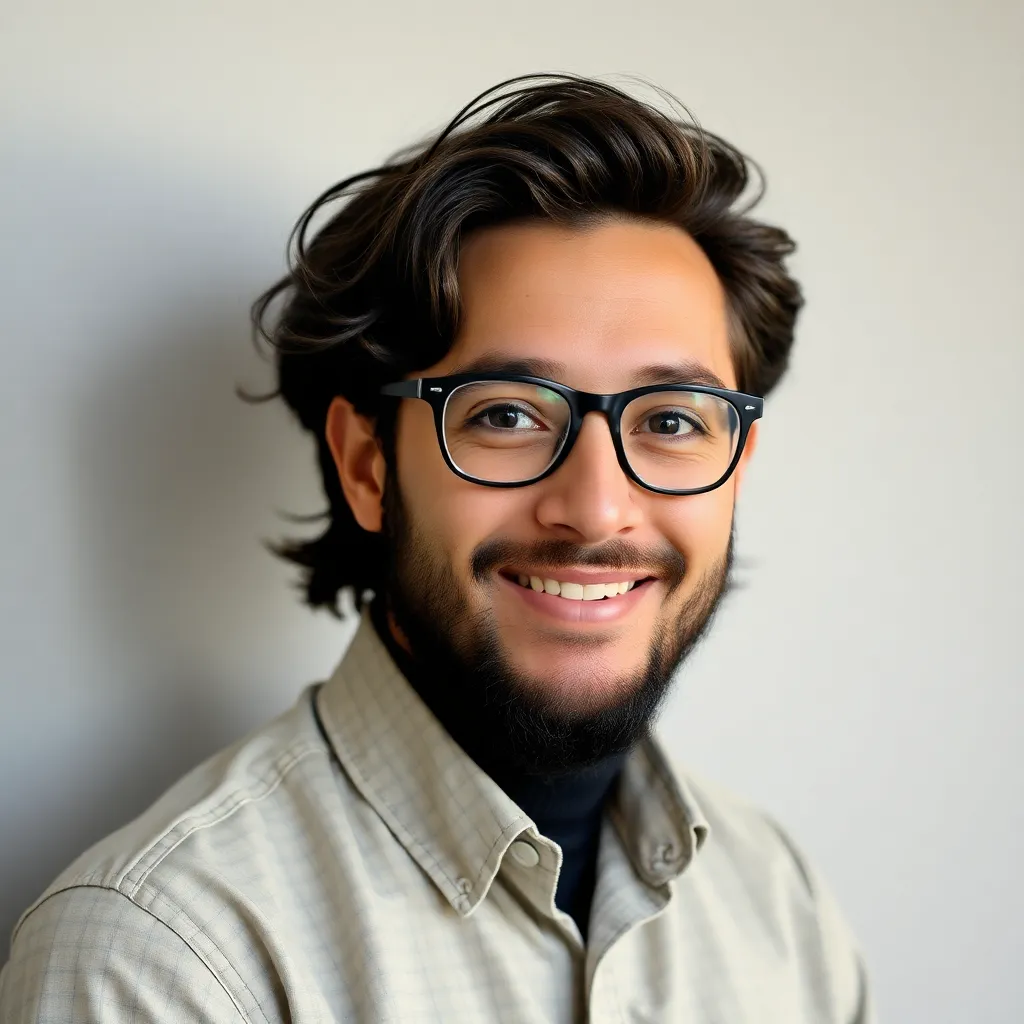
Onlines
May 05, 2025 · 6 min read

Table of Contents
Declaring a Class Does Not Create Actual Objects: Understanding Object-Oriented Programming Fundamentals
In the world of object-oriented programming (OOP), a common point of confusion for beginners lies in the distinction between declaring a class and creating an object. While seemingly intertwined, these two actions are fundamentally different. This article delves into the core concepts, explaining why declaring a class doesn't instantiate objects and clarifying the crucial steps involved in object creation. We'll explore this with examples in several popular programming languages like Python, Java, and C++. Understanding this distinction is crucial for mastering OOP principles and writing efficient, bug-free code.
What is a Class? The Blueprint of Objects
A class serves as a blueprint or template for creating objects. Think of it like an architect's blueprint for a house. The blueprint details the structure, features, and characteristics of the house, but it's not the house itself. Similarly, a class defines the attributes (data) and methods (functions) that objects of that class will possess, but it doesn't create any concrete instances. It merely provides the instructions for object creation.
Key features defined within a class:
-
Attributes (Variables): These represent the data associated with an object. For example, in a
Car
class, attributes might includecolor
,model
, andspeed
. -
Methods (Functions): These define the actions or behaviors an object can perform. In our
Car
example, methods could bestart()
,accelerate()
, andbrake()
.
Example (Python):
class Car:
def __init__(self, color, model): # Constructor
self.color = color
self.model = model
def start(self):
print("The car has started.")
def accelerate(self):
print("The car is accelerating.")
This Python code defines a Car
class. Note that no actual Car
object exists yet. We've only created the blueprint.
The Act of Object Creation: Instantiation
The process of creating an actual object from a class is called instantiation. This is where the blueprint is used to construct a concrete instance of the class. Each instantiated object is a unique entity with its own set of attribute values.
How instantiation works:
-
Memory Allocation: The system allocates memory to store the object's data (attributes).
-
Attribute Initialization: The constructor (
__init__
in Python, similar constructors in other languages) is called to initialize the object's attributes with specific values. -
Object Reference: A reference (variable) is created to point to the allocated memory location holding the object's data.
Example (Python, continuing from above):
my_car = Car("red", "Toyota") # Instantiation
my_car.start() # Calling a method on the object
Here, my_car
is an instance (object) of the Car
class. The Car
constructor is invoked, assigning "red" to my_car.color
and "Toyota" to my_car.model
. We can now interact with my_car
by calling its methods.
Illustrative Examples in Different Programming Languages
Let's look at how class declaration and object creation manifest in other popular languages:
Java:
public class Dog {
String name;
String breed;
public Dog(String name, String breed) {
this.name = name;
this.breed = breed;
}
public void bark() {
System.out.println("Woof!");
}
}
public class Main {
public static void main(String[] args) {
Dog myDog = new Dog("Buddy", "Golden Retriever"); //Instantiation
myDog.bark();
}
}
The Dog
class is declared, but only new Dog(...)
creates an actual Dog
object.
C++:
#include
#include
class Cat {
public:
std::string name;
std::string color;
Cat(std::string name, std::string color) {
this->name = name;
this->color = color;
}
void meow() {
std::cout << "Meow!" << std::endl;
}
};
int main() {
Cat myCat("Whiskers", "Gray"); // Instantiation
myCat.meow();
return 0;
}
Again, the Cat
class is a blueprint; myCat
is the actual object.
Memory Management and the Lifetime of Objects
Understanding memory management is essential when working with objects. When an object is instantiated, memory is allocated to store its data. This memory remains allocated until the object is no longer needed. In many languages, this involves garbage collection, an automatic process that reclaims memory occupied by objects that are no longer referenced.
However, in languages like C++, memory management is often manual, requiring the programmer to explicitly allocate and deallocate memory using new
and delete
(or smart pointers). Failure to manage memory properly can lead to memory leaks or segmentation faults.
Python's Garbage Collection: Python's garbage collector automatically handles memory deallocation. Once an object is no longer referenced, the garbage collector will eventually reclaim the memory it occupies.
Java's Garbage Collection: Similar to Python, Java employs automatic garbage collection. The JVM (Java Virtual Machine) automatically manages memory, freeing up resources when objects are no longer accessible.
C++'s Manual Memory Management: In C++, you need to be mindful of memory management. Using smart pointers can help automate memory deallocation, reducing the risk of memory leaks.
Common Misconceptions
Let's address some common misunderstandings surrounding class declarations and object creation:
-
Declaring a class creates an object: False. A class declaration only defines the structure; it doesn't create an instance.
-
You only need a class to work with objects: False. While classes are fundamental to OOP, you can work with data structures and basic data types without explicitly defining classes (though OOP often provides better organization and reusability).
-
All objects of the same class are identical: False. Objects of the same class have the same structure (attributes and methods), but they can have different attribute values, making them unique instances.
-
Objects are always created using the
new
keyword: False. Whilenew
is commonly used in languages like Java and C++, other languages, like Python, use different mechanisms for instantiation (like simply calling the class name).
Advanced Concepts: Static Members and Class Methods
In some programming languages, classes can have static members (variables and methods). These members belong to the class itself, not to any specific object. They are accessed using the class name, not an object reference. Static methods are often used for utility functions related to the class, but they don't operate on the state of a particular object.
Conclusion: The Foundation of Object-Oriented Programming
Understanding the distinction between declaring a class and creating an object is paramount in object-oriented programming. A class acts as a blueprint, defining the structure and behavior of objects. Object creation (instantiation) involves allocating memory, initializing attributes, and creating a reference to access the object's data and methods. This fundamental understanding forms the bedrock for building complex and efficient applications using OOP principles. Mastering this concept will greatly improve your coding skills and allow you to write more maintainable and scalable software. Remember that each programming language has its nuances in how classes are declared and objects are instantiated, but the underlying principles remain consistent. This knowledge will serve you well as you progress in your OOP journey.
Latest Posts
Latest Posts
-
7 4 Homeostasis And Cells Answer Key
May 05, 2025
-
According To The Cartoonist What Is Found In Alaska
May 05, 2025
-
Imagery In Fahrenheit 451 With Page Numbers
May 05, 2025
-
To Ease The Relocation Of Navy Personnel And Their Families
May 05, 2025
-
Graphics Such As Shapes Diagrams Lines Or Circles
May 05, 2025
Related Post
Thank you for visiting our website which covers about Declaring A Class Does Not Create Actual Objects . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.