Echoes From The Past Unit Test
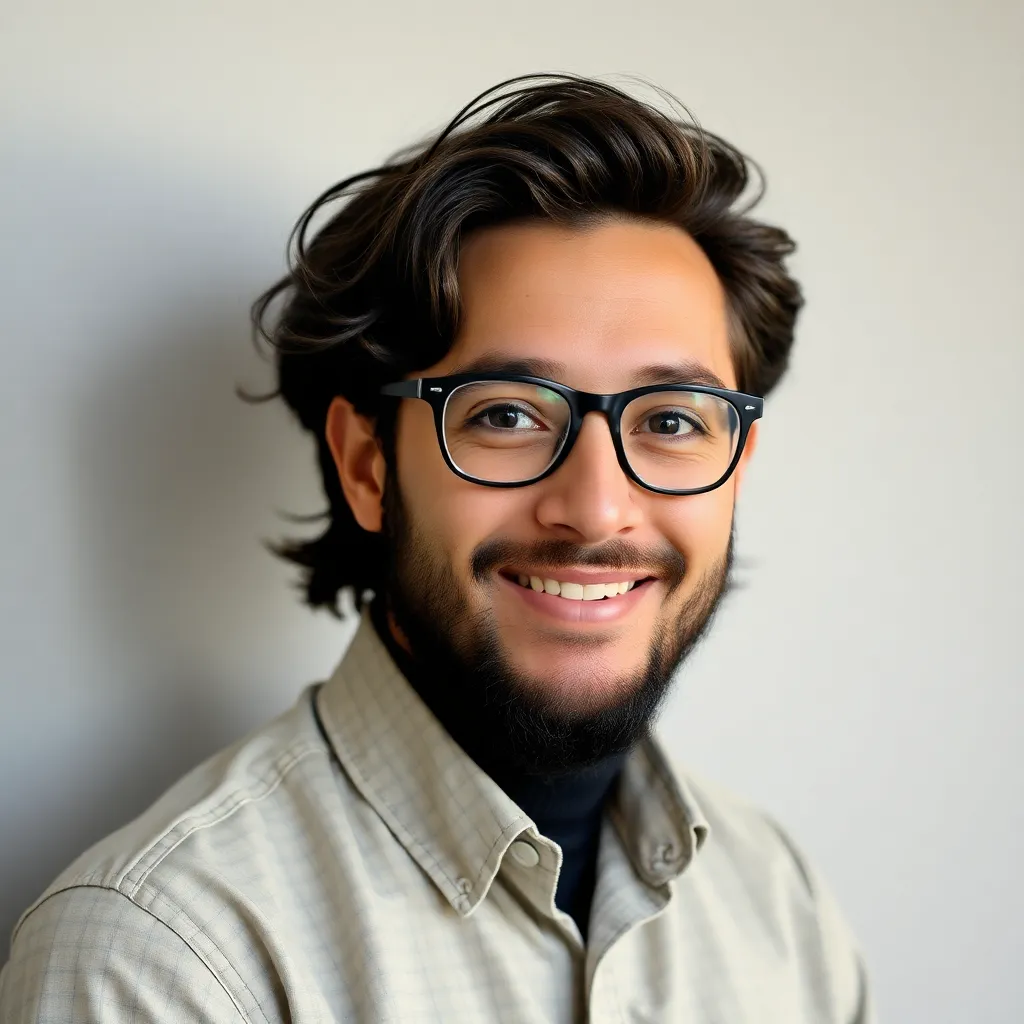
Onlines
Mar 30, 2025 · 6 min read
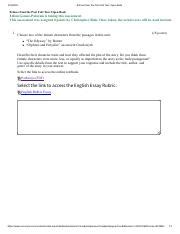
Table of Contents
Echoes from the Past: A Comprehensive Guide to Unit Testing
Unit testing is the cornerstone of robust software development. It's the process of testing individual components, or units, of your code in isolation to ensure they function correctly. When dealing with complex projects, or projects with a legacy codebase like "Echoes from the Past" (a fictional project used for illustrative purposes throughout this article), a thorough unit testing strategy is paramount. This comprehensive guide explores various aspects of unit testing, focusing on practical strategies, common pitfalls, and best practices to effectively test even the most intricate parts of a software project.
Understanding the "Echoes from the Past" Scenario
Imagine "Echoes from the Past" is a historical simulation game. It might involve complex game logic, intricate data structures representing historical figures and events, and a user interface. Testing such a system requires a multifaceted approach, with unit testing forming the foundation. Without proper unit tests, even minor changes can introduce unexpected bugs and regressions, especially in a project with a history (hence the name!).
Why Unit Testing Matters in "Echoes from the Past"
The benefits of unit testing in a project like "Echoes from the Past" are numerous:
-
Early Bug Detection: Identifying and fixing bugs early in the development cycle is significantly cheaper and easier than addressing them later. Unit tests help find these bugs before they escalate into larger, more complex problems.
-
Improved Code Quality: The process of writing unit tests forces developers to think more carefully about their code's design and structure. This leads to cleaner, more maintainable code.
-
Faster Development: While writing unit tests takes time upfront, it ultimately speeds up the development process by reducing the time spent debugging and fixing bugs later on.
-
Facilitates Refactoring: With a strong suite of unit tests, developers can confidently refactor their code – improving its design without fear of introducing new bugs. The tests act as a safety net, ensuring that the functionality remains intact after changes.
-
Enhanced Code Documentation: Well-written unit tests can serve as a form of living documentation, illustrating how different parts of the code are intended to be used.
Essential Aspects of Unit Testing in "Echoes from the Past"
Let's dive into the practical aspects of implementing unit testing within the context of our fictional "Echoes from the Past" game.
1. Choosing a Testing Framework
The first step is selecting a suitable testing framework. Popular choices include:
-
JUnit (Java): A widely used framework for Java projects. Its simple syntax and extensive features make it a great choice for many applications.
-
pytest (Python): A powerful and flexible Python testing framework known for its ease of use and extensive plugin ecosystem.
-
NUnit (.NET): A popular unit testing framework for .NET languages, providing a similar feature set to JUnit.
-
Jest (JavaScript): A widely adopted framework for JavaScript, particularly in React and other front-end projects. Its focus on simplicity and speed makes it a compelling option.
The best framework depends on the programming language used in "Echoes from the Past". For example, if the game's back-end is written in Java, JUnit would be an excellent choice.
2. Defining Test Cases
Creating effective test cases is crucial. For "Echoes from the Past", this might involve:
-
Testing Game Logic: Unit tests should verify the game's core mechanics. For example, a test could check if a battle simulation correctly calculates the outcome based on troop strengths and other factors.
-
Data Structure Validation: Test cases should validate the integrity of data structures. This could involve testing the functionality of classes representing historical figures, ensuring their attributes are correctly stored and manipulated.
-
User Interface (UI) Testing (Indirect): While UI testing usually falls under integration testing, some aspects can be partially covered by unit testing components that interact directly with the UI, such as data validation functions or functions that format data for display.
Example Test Case (Python with pytest):
import pytest
from echoes_from_the_past.game_logic import calculate_battle_outcome
def test_battle_outcome_victory():
"""Test a battle where Player 1 wins."""
player1_strength = 100
player2_strength = 50
outcome = calculate_battle_outcome(player1_strength, player2_strength)
assert outcome == "Player 1 wins"
def test_battle_outcome_defeat():
"""Test a battle where Player 1 loses."""
player1_strength = 50
player2_strength = 100
outcome = calculate_battle_outcome(player1_strength, player2_strength)
assert outcome == "Player 2 wins"
def test_battle_outcome_draw():
"""Test a battle that ends in a draw."""
player1_strength = 100
player2_strength = 100
outcome = calculate_battle_outcome(player1_strength, player2_strength)
assert outcome == "Draw"
This example shows simple tests for a calculate_battle_outcome
function. More complex scenarios will require more sophisticated test cases.
3. Mocking and Stubbing
In a project as large as "Echoes from the Past", many components interact. To isolate a unit during testing, techniques like mocking and stubbing are crucial. Mocking replaces a dependency with a simulated version, while stubbing provides canned responses to function calls.
This allows you to focus solely on the behaviour of the unit under test without worrying about external factors. For example, you might mock a database connection to prevent test failures from database errors.
4. Test-Driven Development (TDD)
Consider adopting TDD, a development process where you write tests before writing the actual code. This ensures that you have a clear understanding of the required functionality and helps to design more testable code.
5. Continuous Integration (CI)
Integrate unit testing into your CI/CD pipeline. This ensures that every code change is automatically tested, preventing regressions and improving the overall stability of the project. Tools like Jenkins, GitLab CI, or GitHub Actions can automate this process.
Advanced Techniques and Considerations
As "Echoes from the Past" grows, you'll likely encounter more complex testing scenarios:
-
Property-Based Testing: This involves generating random test data to verify that the code behaves correctly across a wide range of inputs. This can reveal edge cases that might be missed by manually crafted test cases.
-
Integration Testing: While this goes beyond unit testing, it's crucial for verifying the interaction between different components in "Echoes from the Past". Integration tests would cover scenarios such as the interaction between the game's battle simulation and the UI.
-
Performance Testing: As the game grows, you may need to implement performance tests to ensure the game remains responsive even under heavy load.
-
Code Coverage: Tools measure the percentage of code covered by unit tests. Aim for high code coverage (ideally above 80%), but remember that high coverage doesn't guarantee perfect quality; focus on testing critical paths and edge cases.
Common Pitfalls to Avoid
Several common mistakes can hinder your unit testing efforts:
-
Overly Complex Tests: Keep your tests concise and focused. Avoid creating overly complex test cases that test multiple things at once.
-
Ignoring Edge Cases: Pay special attention to edge cases and boundary conditions. These are often sources of subtle bugs.
-
Insufficient Test Coverage: Ensure a reasonable level of code coverage. Untested code is a potential source of bugs.
-
Ignoring Test Maintainability: As the project evolves, keep your tests updated and maintainable. Outdated or poorly written tests become a burden rather than an asset.
Conclusion: Building a Resilient "Echoes from the Past"
Thorough unit testing is essential for building a robust and maintainable project like "Echoes from the Past". By following best practices, choosing appropriate testing frameworks, and addressing potential pitfalls, you can create a stable foundation for future development and minimize the risks of regressions. Investing time and effort in unit testing from the start will pay dividends in the long run, resulting in a higher-quality game and a more efficient development process. Remember, consistent and comprehensive testing is not just a good practice; it is a critical element in creating software that stands the test of time, much like the historical echoes your game seeks to evoke.
Latest Posts
Latest Posts
-
Select The True Statements Regarding Federalism And Its Political Ramifications
Apr 01, 2025
-
What Is Not Likely To Happen At A Divergent Boundary
Apr 01, 2025
-
4 27 Unit Test Astronomy Part 1
Apr 01, 2025
-
Import The Text File Pb Participants Txt As A Table
Apr 01, 2025
-
Chapter 10 Summary Of The Scarlet Letter
Apr 01, 2025
Related Post
Thank you for visiting our website which covers about Echoes From The Past Unit Test . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.