Import The Comma Delimited File Accessories.csv
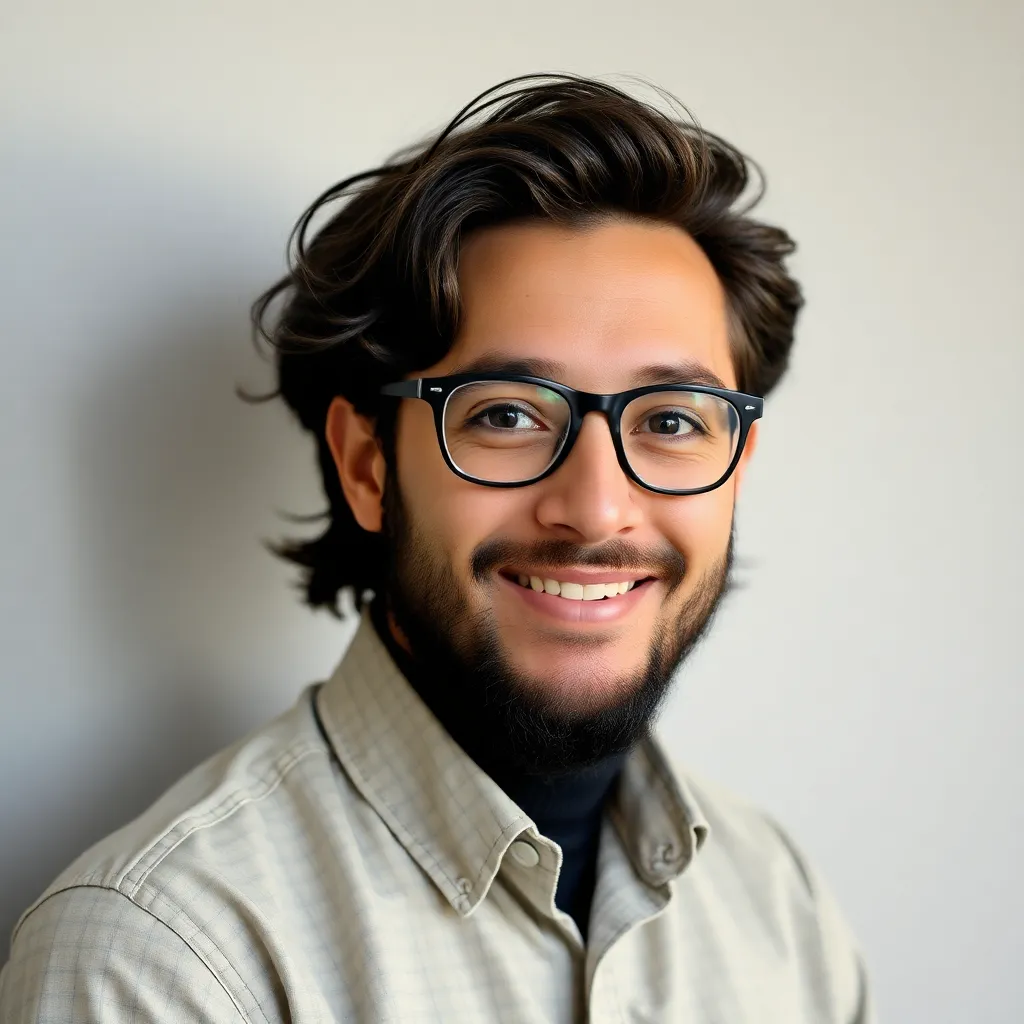
Onlines
May 10, 2025 · 4 min read
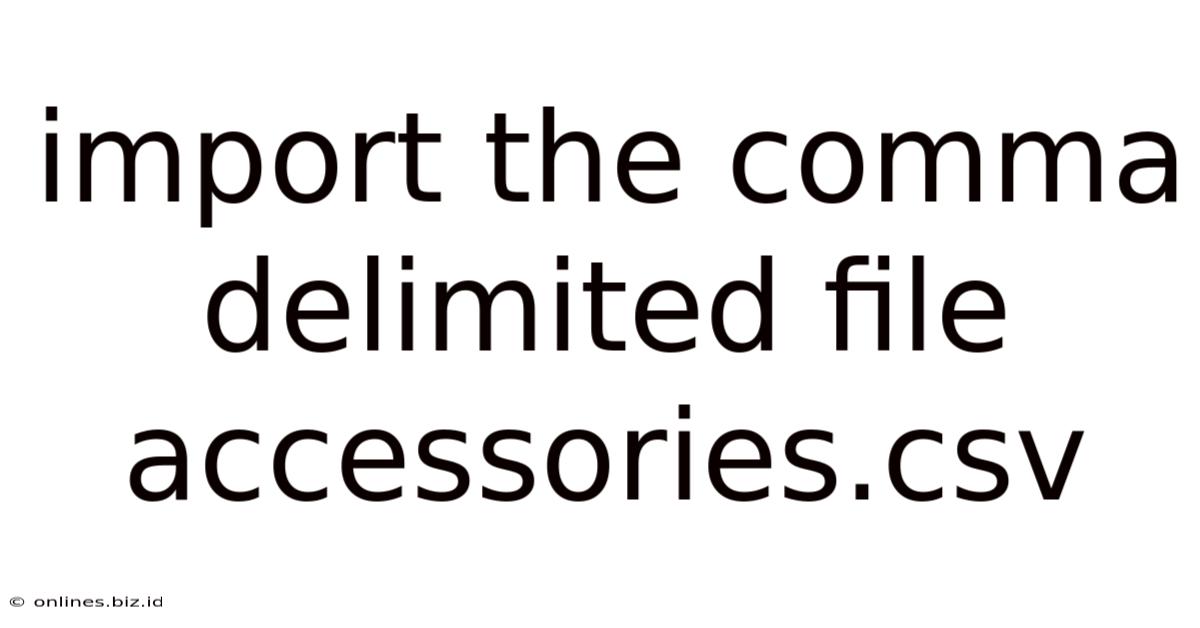
Table of Contents
Importing and Working with Comma-Delimited Files: A Comprehensive Guide to accessories.csv
Importing and processing data from comma-separated value (CSV) files is a fundamental task in data analysis and programming. This comprehensive guide will walk you through the process of importing accessories.csv
(assuming it's a file containing accessory data) and manipulating the data within it, covering various programming languages and approaches. We'll explore efficient techniques for handling large datasets and potential challenges you might encounter.
Understanding CSV Files and accessories.csv
A CSV file is a simple text file that stores tabular data (like a spreadsheet) where each line represents a row, and values within a row are separated by commas. accessories.csv
, in this context, likely contains information about different accessories, possibly including attributes like:
- Accessory ID: A unique identifier for each accessory.
- Accessory Name: The name of the accessory.
- Description: A brief description of the accessory.
- Price: The cost of the accessory.
- Category: The type of accessory (e.g., phone case, screen protector).
- Stock Level: The number of units currently in stock.
- Supplier: The company supplying the accessory.
Importing accessories.csv
in Python
Python, with its rich ecosystem of libraries, offers excellent capabilities for handling CSV data. The most common library is the csv
module, built into Python's standard library. However, for larger datasets or more complex data manipulation, pandas
is often preferred.
Using the csv
Module
import csv
with open('accessories.csv', 'r', newline='') as csvfile:
reader = csv.reader(csvfile)
# Skip the header row if present
next(reader, None)
for row in reader:
accessory_id = row[0]
accessory_name = row[1]
# ... process other columns ...
print(f"Accessory ID: {accessory_id}, Name: {accessory_name}")
This code opens accessories.csv
, reads it row by row, and then processes each row. The newline=''
argument prevents potential issues with line endings. Remember to adapt column indexing (e.g., row[0]
, row[1]
) to match the structure of your accessories.csv
file.
Leveraging the Power of Pandas
Pandas provides a more powerful and efficient way to work with CSV data, especially for larger files.
import pandas as pd
df = pd.read_csv('accessories.csv')
# Accessing data:
print(df.head()) # Display the first few rows
print(df['Accessory Name']) # Access a specific column
print(df[df['Price'] > 50]) # Filtering data based on a condition
# Data manipulation:
df['Price'] = df['Price'] * 1.1 # Increase prices by 10%
df.to_csv('updated_accessories.csv', index=False) # Save the changes
Pandas automatically handles header rows and provides many built-in functions for data cleaning, transformation, and analysis. The read_csv()
function efficiently handles large files, and the DataFrame object allows for easy data manipulation.
Importing accessories.csv
in R
R is another powerful statistical computing language with excellent capabilities for data manipulation and analysis. The read.csv()
function is the primary tool for importing CSV data.
# Import the CSV file
accessories_data <- read.csv("accessories.csv", header = TRUE, stringsAsFactors = FALSE)
# Explore the data
head(accessories_data) # View the first few rows
summary(accessories_data) # Get summary statistics
# Accessing specific columns:
accessories_data$Accessory.Name # Access the "Accessory Name" column
# Data manipulation:
accessories_data$Price <- accessories_data$Price * 1.1 # Increase prices by 10%
# Saving the modified data:
write.csv(accessories_data, "updated_accessories.csv", row.names = FALSE)
Similar to Pandas in Python, read.csv()
in R handles header rows automatically and provides various options to control the import process. The stringsAsFactors = FALSE
argument prevents R from automatically converting character columns into factors.
Handling Large accessories.csv
Files
For exceptionally large accessories.csv
files, memory efficiency becomes crucial. Here are some strategies:
-
Iterative Processing: Instead of loading the entire file into memory at once, process it row by row or in chunks using iterators (Python's
csv
module or Pandas'chunksize
parameter inread_csv()
). -
Data Sampling: If you don't need to analyze the entire dataset, randomly sample a subset for faster processing.
-
Database Integration: For very large datasets, consider storing the data in a database (e.g., SQLite, PostgreSQL) and querying it using SQL. This offers better performance and scalability.
Error Handling and Data Cleaning
Real-world CSV files are often imperfect. Expect and handle potential issues:
-
Missing Values: Use appropriate techniques (e.g., imputation, removal) to deal with missing data. Pandas and R offer functions for this.
-
Inconsistent Data Formats: Ensure data consistency (e.g., dates, numbers). Data cleaning might involve reformatting or converting data types.
-
Encoding Issues: Incorrect file encoding can lead to errors. Specify the encoding (e.g., UTF-8, Latin-1) during file import.
Advanced Techniques
-
Data Transformation: Apply transformations like normalization, standardization, or feature engineering to improve data quality and analysis results.
-
Data Visualization: Use libraries like Matplotlib (Python) or ggplot2 (R) to create informative charts and graphs based on the data.
-
Data Modeling: Build statistical models (regression, classification) to extract insights from the data.
Conclusion
Importing and working with CSV files like accessories.csv
is an essential skill for anyone dealing with data. This guide provides a comprehensive overview of the techniques and considerations involved in various programming languages. By choosing the right tools and understanding potential challenges, you can efficiently process and analyze your data to gain valuable insights. Remember to adapt the code snippets provided to the specific structure and contents of your accessories.csv
file. Always prioritize data quality and apply appropriate error handling techniques for robust and reliable data analysis.
Latest Posts
Latest Posts
-
The Interaction Between Information Technology And Organizations Is Influenced
May 10, 2025
-
Art Labeling Activity Structure Of A Skeletal Muscle Fiber
May 10, 2025
-
Potential Gdp In The U S Will Be Unaffected By
May 10, 2025
-
Select This Action Type For A Transfer Par
May 10, 2025
-
Which Statements About Tornadoes Are True Check All That Apply
May 10, 2025
Related Post
Thank you for visiting our website which covers about Import The Comma Delimited File Accessories.csv . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.