In A C++ Program Two Slash Marks Indicate
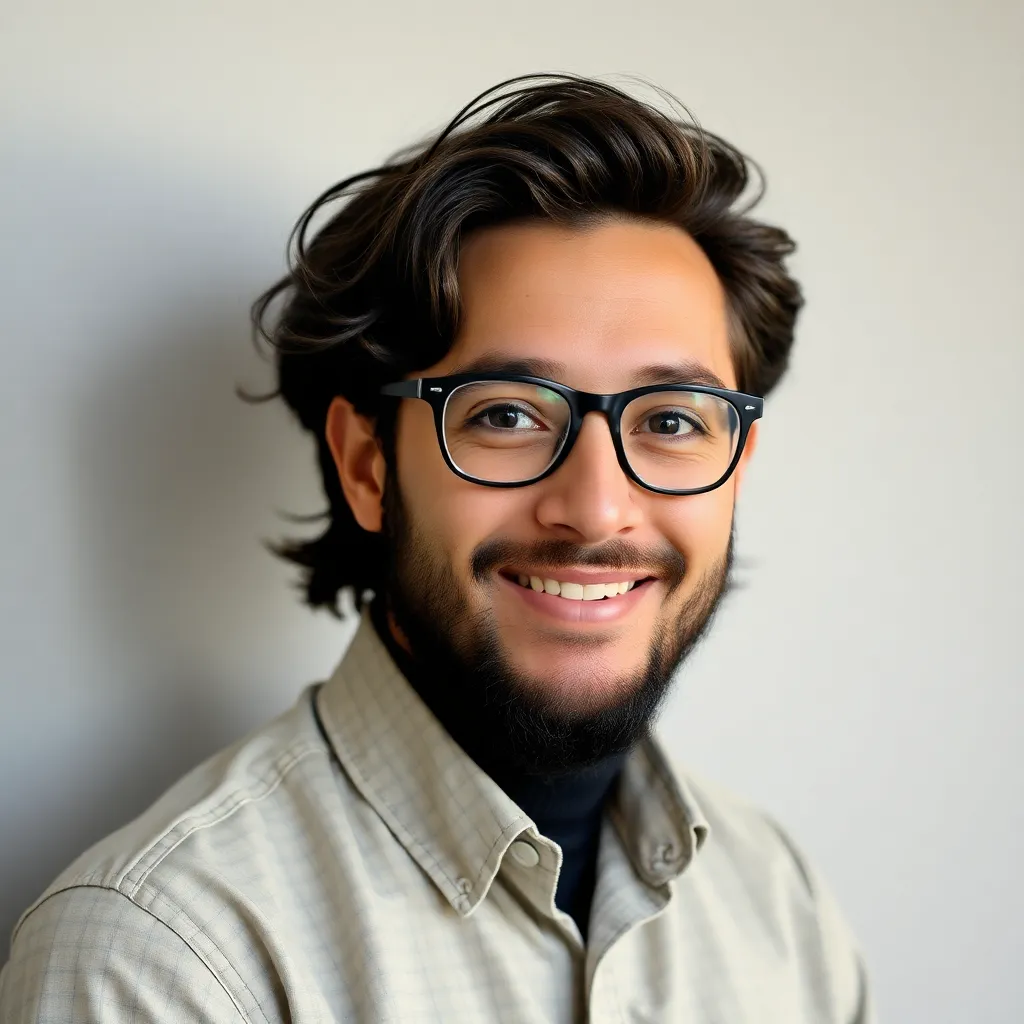
Onlines
May 09, 2025 · 5 min read
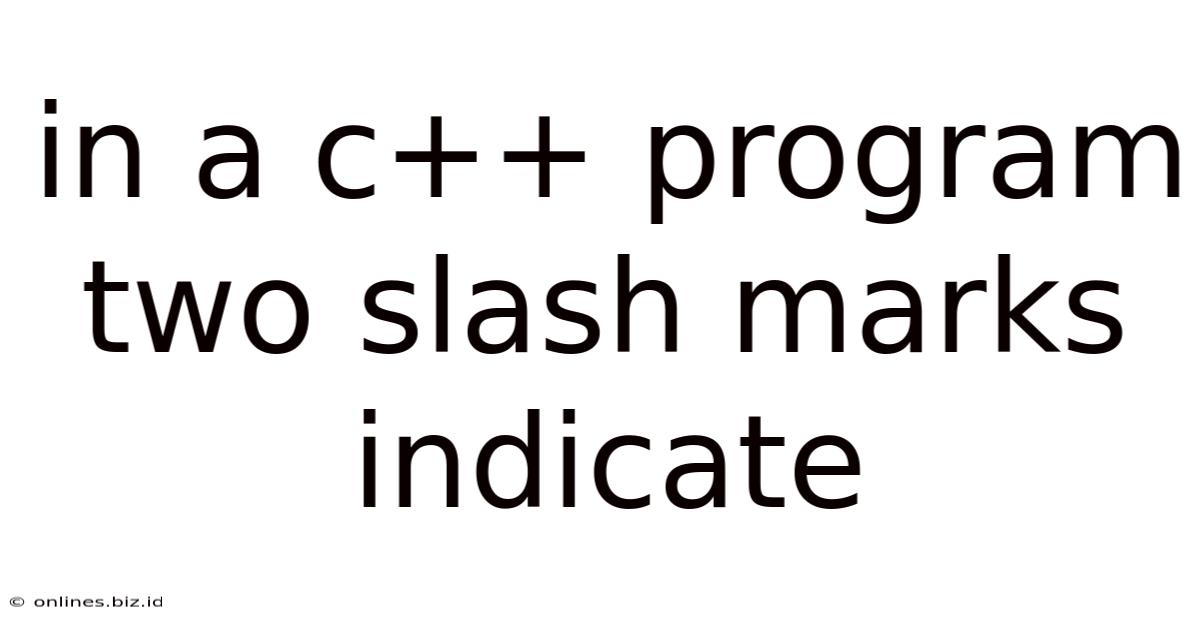
Table of Contents
In a C++ Program, Two Slash Marks Indicate a Single-Line Comment
In the world of C++, the humble double forward slash (//
) plays a crucial role, far beyond its simple appearance. Understanding its function is fundamental for writing clean, efficient, and maintainable code. This article delves deep into the significance of //
in C++, exploring its purpose, best practices for its use, and how it differs from other commenting techniques. We'll also look at common misconceptions and advanced scenarios where understanding comments becomes even more important.
The Primary Function: Single-Line Comments
At its core, //
in C++ denotes a single-line comment. This means that anything written after //
on a single line of code is ignored by the compiler. The compiler effectively treats the commented-out text as if it were not present in the source code. This feature is invaluable for several reasons:
1. Explaining Code Logic:
Comments are crucial for clarifying complex algorithms or non-obvious code snippets. Let's illustrate:
// Calculate the factorial of a number using a loop
int factorial(int n) {
int result = 1;
for (int i = 1; i <= n; i++) {
result *= i; // Multiply result by the current number
}
return result;
}
In this example, the comments clearly explain the purpose of the factorial
function and the steps involved in calculating the factorial. This makes the code far easier to understand, even for someone unfamiliar with the specific implementation.
2. Documenting Code Changes:
When modifying existing code, comments are essential for tracking changes and explaining the reasons behind those changes. This is especially helpful in collaborative programming environments.
// Modified on 2024-10-27 to handle negative input values
// Previous version did not account for this edge case
int factorial(int n) {
if (n < 0) {
return -1; // Indicate an error for negative input
}
// ... rest of the factorial calculation ...
}
Here, the comments clearly document the date of the modification and the reason for the change. This information is invaluable for debugging and maintaining the codebase over time.
3. Temporarily Disabling Code:
During development, you might want to temporarily disable a section of code without deleting it. Comments are perfectly suited for this purpose:
// This function is currently under development
//int calculateComplexValue(int a, int b) {
// // ... implementation ...
//}
By simply adding //
at the beginning of the lines, the function becomes inactive without requiring the programmer to delete the code, making it readily available for future use or debugging.
4. Adding Metadata or Notices:
Comments can be used to include information such as copyright notices, author details, or version numbers.
// Copyright 2024 Example Corp. All rights reserved.
// Author: John Doe
// Version: 1.0
This helps to maintain proper attribution and version control of the code.
Best Practices for Using Single-Line Comments
While comments are beneficial, excessive or poorly written comments can clutter the code and hinder readability. Therefore, following best practices is crucial:
- Be Concise and Clear: Avoid lengthy, rambling comments. Strive for clarity and precision.
- Comment the "Why," Not the "What": Comments should explain the purpose of the code, not simply restate what the code already does.
- Keep Comments Up-to-Date: If code changes, update the corresponding comments to reflect those changes. Out-of-date comments are worse than no comments at all.
- Use Consistent Formatting: Maintain a consistent style for your comments, both in terms of spacing and capitalization.
- Avoid Over-Commenting: Don't comment every single line of code; focus on sections that require explanation or clarification.
- Use Meaningful Variable Names: Well-chosen variable names often reduce the need for excessive comments. For example,
customerName
is more descriptive thanx
. - Use TODO Comments: Use
// TODO: ...
to mark sections of code that need to be implemented or revisited later.
Multi-Line Comments (/* ... */
) and Their Differences
C++ also supports multi-line comments using /*
to start and */
to end the comment block. These comments can span multiple lines, making them suitable for longer explanations or documenting larger sections of code. Here's an example:
/*
This function performs a complex calculation involving
multiple steps. The algorithm is based on ...
Further details can be found in the accompanying documentation.
*/
int complexCalculation(int a, int b, int c) {
// ... implementation ...
}
While multi-line comments offer flexibility, they should be used judiciously. Single-line comments (//
) are generally preferred for brevity and clarity, especially for shorter explanations. Avoid nesting multi-line comments (/* ... /* ... */ ... */
) as this can lead to confusion and errors.
Advanced Scenarios and Important Considerations
Understanding comments becomes even more critical in advanced scenarios:
- Code Refactoring: When refactoring code, comments help to track changes and understand the implications of those changes.
- Debugging: Comments can help to isolate problematic sections of code and aid in the debugging process.
- Code Reviews: Comments facilitate code reviews by providing context and explaining the design choices made by the programmer.
- Legacy Code Maintenance: Comments are vital in maintaining legacy code by explaining the logic and functionality of older code segments.
Common Misconceptions
- Comments as a Replacement for Clean Code: Comments should not be used as a crutch for poorly written code. If the code is difficult to understand, the best solution is to improve the code itself, not just add more comments.
- Over-Commenting: Excessive comments can make code harder to read and maintain. Strive for balance.
- Ignoring Existing Comments: Always review existing comments before modifying code. Out-of-date comments can lead to errors.
Conclusion
In C++, the double forward slash (//
) represents a powerful yet often underestimated tool. Used effectively, single-line comments (//
) significantly enhance code readability, maintainability, and collaboration. However, remember that well-written, concise, and up-to-date comments are essential. Over-commenting or neglecting comments altogether can equally hinder code quality. By adhering to best practices and understanding the nuances of comment usage, you can contribute to developing high-quality, robust, and easily maintainable C++ software. Mastering the use of //
is a key step towards becoming a proficient C++ programmer.
Latest Posts
Latest Posts
-
Which Statement Below Is Not An Example Of Ethnocentrism
May 10, 2025
-
Catcher In The Rye Chapter 20
May 10, 2025
-
According To Hindson In Everyday Biblical Worldview Salvation Must Include
May 10, 2025
-
In Behavior Modification A Research Design Involves
May 10, 2025
-
Germanys Mittelstand Companies Have Been Extremely Successful Pursuing
May 10, 2025
Related Post
Thank you for visiting our website which covers about In A C++ Program Two Slash Marks Indicate . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.