Part A Drag The Appropriate Labels To Their Respective Targets.
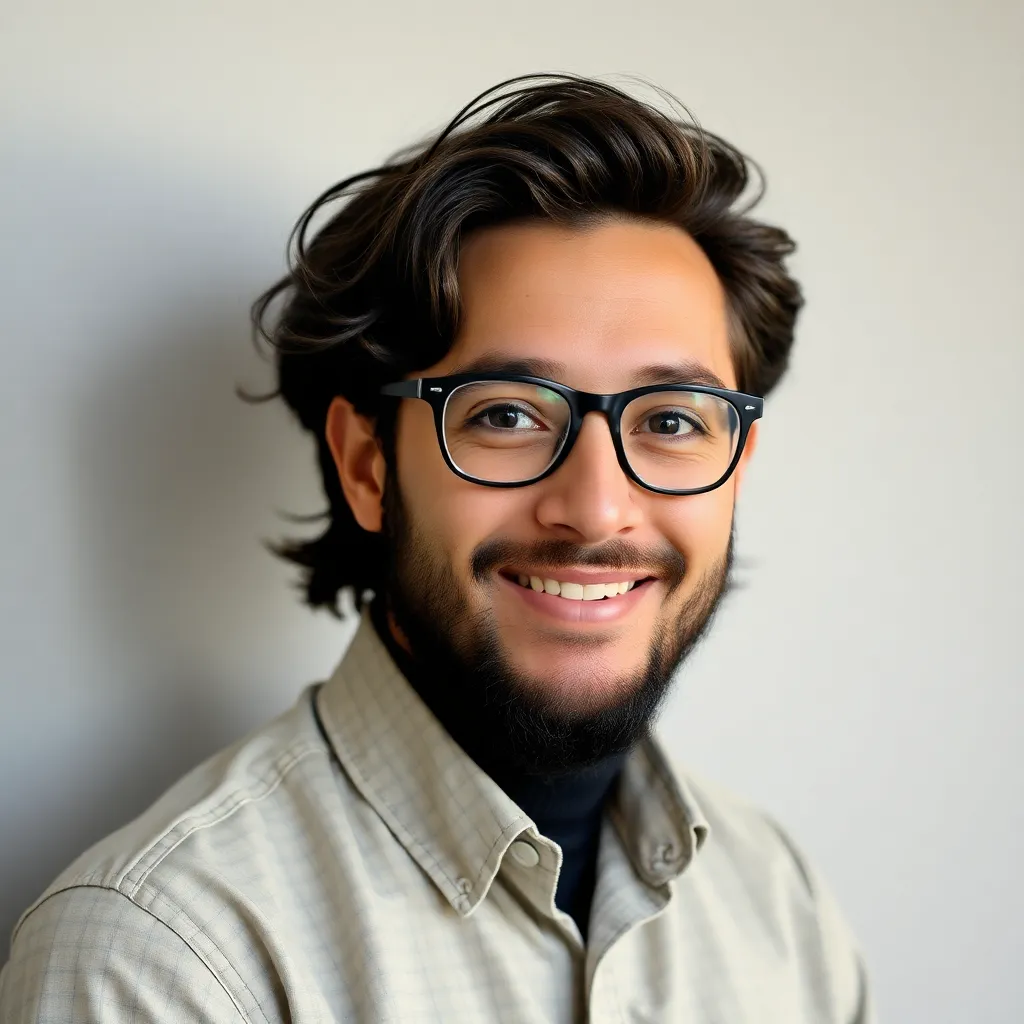
Onlines
May 08, 2025 · 6 min read
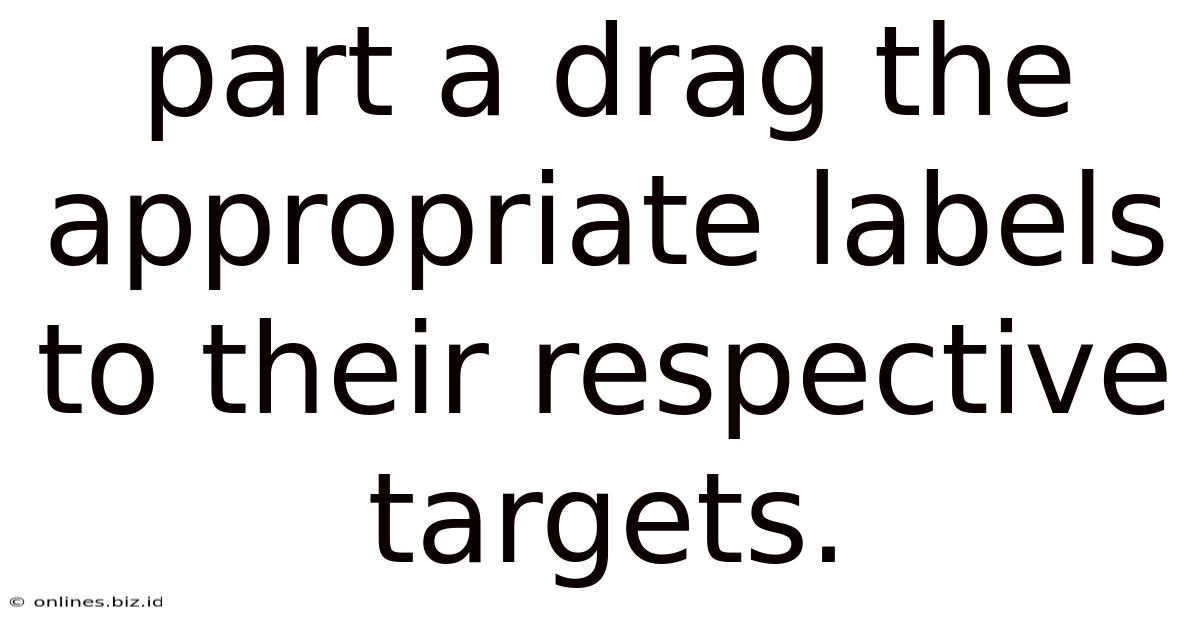
Table of Contents
- Part A Drag The Appropriate Labels To Their Respective Targets.
- Table of Contents
- Mastering the Art of Drag-and-Drop Interactions: A Comprehensive Guide
- Part A: Understanding the Drag-and-Drop Mechanism
- Part B: Implementing Drag-and-Drop Functionality: A Practical Example (Conceptual)
- Part C: Advanced Techniques and Best Practices
- Part D: Frameworks and Libraries
- Part E: Troubleshooting Common Issues
- Part F: Beyond the Basics: Creative Applications of Drag-and-Drop
- Conclusion: Mastering the Art of Drag and Drop
- Latest Posts
- Related Post
Mastering the Art of Drag-and-Drop Interactions: A Comprehensive Guide
Drag-and-drop interactions are ubiquitous in modern user interfaces. From simple file management to complex data visualizations, this intuitive interaction pattern enhances user experience significantly. Understanding the intricacies of drag-and-drop functionality, however, is crucial for developers and designers alike. This comprehensive guide delves into the mechanics, best practices, and considerations involved in effectively implementing drag-and-drop features. We'll explore the technical aspects, user experience implications, and accessibility considerations, providing a holistic understanding of this powerful interaction design element.
Part A: Understanding the Drag-and-Drop Mechanism
The seemingly simple act of dragging and dropping an element involves a complex interplay of events and actions. Let's break down the core components:
1. The Draggable Element: This is the element the user interacts with directly. It can be anything from a file icon to a complex UI component. Crucially, this element needs to be explicitly designated as draggable. This usually involves setting a specific attribute or property in the relevant programming language (e.g., draggable="true"
in HTML).
2. The Drag Event: When the user initiates a drag action (typically by clicking and holding the mouse button), a series of drag events are triggered. These events allow the application to track the movement of the draggable element and respond accordingly. Key drag events include:
dragstart
: Fired when the drag operation begins. This is where you typically set the data being dragged (usingdataTransfer
object) and potentially initiate visual feedback (like a ghost image).drag
: Fired repeatedly as the user drags the element. This allows for real-time updates and feedback.dragend
: Fired when the drag operation ends, regardless of whether a drop occurred. This is where cleanup tasks, like removing temporary visual effects, are usually handled.
3. The Drop Target: This is the element or area where the user intends to drop the draggable element. Similar to the draggable element, the drop target needs to be explicitly defined. This usually involves setting event listeners for drop-related events.
4. The Drop Event: When the user releases the mouse button over a valid drop target, a series of drop events are fired. Key drop events include:
dragenter
: Fired when the draggable element enters the drop target's area.dragover
: Fired repeatedly as the draggable element moves over the drop target. This event is crucial for providing visual feedback to the user, indicating whether the drop is allowed. Crucially, this event's default behavior needs to be prevented (preventDefault()
) to allow the drop to occur.drop
: Fired when the draggable element is released over the drop target. This event is where the actual data transfer happens. The data set in thedragstart
event usingdataTransfer
is accessed here.dragleave
: Fired when the draggable element leaves the drop target's area. This event is used for removing visual feedback.
5. Data Transfer (dataTransfer
Object): This crucial object acts as a bridge between the draggable element and the drop target, allowing the transfer of data during the drag-and-drop operation. You can add data to this object in various formats (text, URLs, files, etc.) using methods like setData()
, and access this data in the drop event using getData()
.
Part B: Implementing Drag-and-Drop Functionality: A Practical Example (Conceptual)
Let's outline a conceptual implementation using JavaScript and HTML. Note: This is a simplified example and would require adjustments depending on the specific framework or library used.
HTML Structure:
Drag me!
Drop here
JavaScript Logic:
// Draggable element
const draggable = document.getElementById('draggable');
draggable.addEventListener('dragstart', (e) => {
e.dataTransfer.setData('text/plain', 'Dragged item!');
});
// Dropzone
const dropzone = document.getElementById('dropzone');
dropzone.addEventListener('dragover', (e) => {
e.preventDefault(); // Crucial for allowing the drop
});
dropzone.addEventListener('drop', (e) => {
e.preventDefault();
const data = e.dataTransfer.getData('text/plain');
dropzone.innerText = 'Dropped: ' + data;
});
This code snippet demonstrates the basic principles. In a real-world scenario, you would need to incorporate more robust error handling, visual feedback, and potentially data validation.
Part C: Advanced Techniques and Best Practices
1. Custom Drag Images: Instead of the default browser-generated drag image, you can use a custom image to represent the dragged item, enhancing the user experience. This is done by setting the dataTransfer.setDragImage()
method.
2. Multiple Drop Targets: Handling multiple drop targets involves adding event listeners to each target and implementing logic to determine which target receives the dropped element.
3. Data Validation: Before accepting a drop, you should validate the data being transferred to ensure it meets your application's requirements. This could involve checking data types, formats, or other criteria.
4. Visual Feedback: Providing clear visual feedback during the drag-and-drop process is crucial for usability. This could involve highlighting the drop target, displaying a preview of the dragged item, or showing progress indicators.
5. Handling Different Data Types: The dataTransfer
object allows transferring data in various formats. Your implementation should handle different data types appropriately.
6. Accessibility Considerations: Ensure that your drag-and-drop interaction is accessible to users with disabilities. This involves providing keyboard alternatives and clear screen reader support.
Part D: Frameworks and Libraries
Several frameworks and libraries simplify the implementation of drag-and-drop functionality, handling complexities like cross-browser compatibility and providing advanced features. Popular choices include:
- React DnD: A popular library for building drag-and-drop interfaces in React applications.
- Angular CDK Drag and Drop: Provides drag-and-drop capabilities within the Angular framework.
- Vue.js Drag and Drop Libraries: Several libraries offer drag-and-drop functionality for Vue.js applications.
- HTML5 Drag and Drop API: While not a framework, understanding the native HTML5 drag-and-drop API is fundamental for any implementation.
Part E: Troubleshooting Common Issues
1. preventDefault()
Not Working: Failure to call preventDefault()
in the dragover
event is a common mistake, preventing the drop from occurring.
2. Data Not Transferring: Incorrect use of setData()
and getData()
methods can lead to data transfer issues. Double-check data types and method calls.
3. Browser Compatibility: While the HTML5 drag-and-drop API is widely supported, some older browsers might have limited functionality. Consider using a framework or library to handle cross-browser compatibility.
4. Performance Issues: For large datasets or complex interactions, performance can become an issue. Optimize your code and consider using techniques like virtualization or chunking to improve efficiency.
Part F: Beyond the Basics: Creative Applications of Drag-and-Drop
Drag-and-drop interactions are not limited to simple file transfers. They can be used to create highly engaging and intuitive user experiences in a variety of applications:
- Interactive Data Visualization: Drag-and-drop can be used to manipulate data points, filter information, or rearrange elements in visualizations, enhancing user interaction and exploration.
- Kanban Boards: Many project management tools use drag-and-drop to move tasks between different stages of a workflow.
- E-commerce Applications: Drag-and-drop is used for adding items to shopping carts, customizing products, or arranging items in a wish list.
- Form Builders: Drag-and-drop allows users to create custom forms by dragging and dropping form elements.
- Game Development: Drag-and-drop is used to create interactive game elements and user interfaces.
Conclusion: Mastering the Art of Drag and Drop
Drag-and-drop interactions, though seemingly simple, represent a powerful tool for creating engaging and intuitive user interfaces. By understanding the underlying mechanisms, employing best practices, and leveraging available frameworks and libraries, developers and designers can craft seamless and effective drag-and-drop experiences that enhance user engagement and productivity. Remember to always prioritize accessibility and carefully consider the overall user experience when implementing this powerful interaction design element. The key to success lies in a deep understanding of the technical aspects and a keen eye for user-centered design.
Latest Posts
Related Post
Thank you for visiting our website which covers about Part A Drag The Appropriate Labels To Their Respective Targets. . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.