Pltw 3.1.1 Inputs And Outputs Answer Key
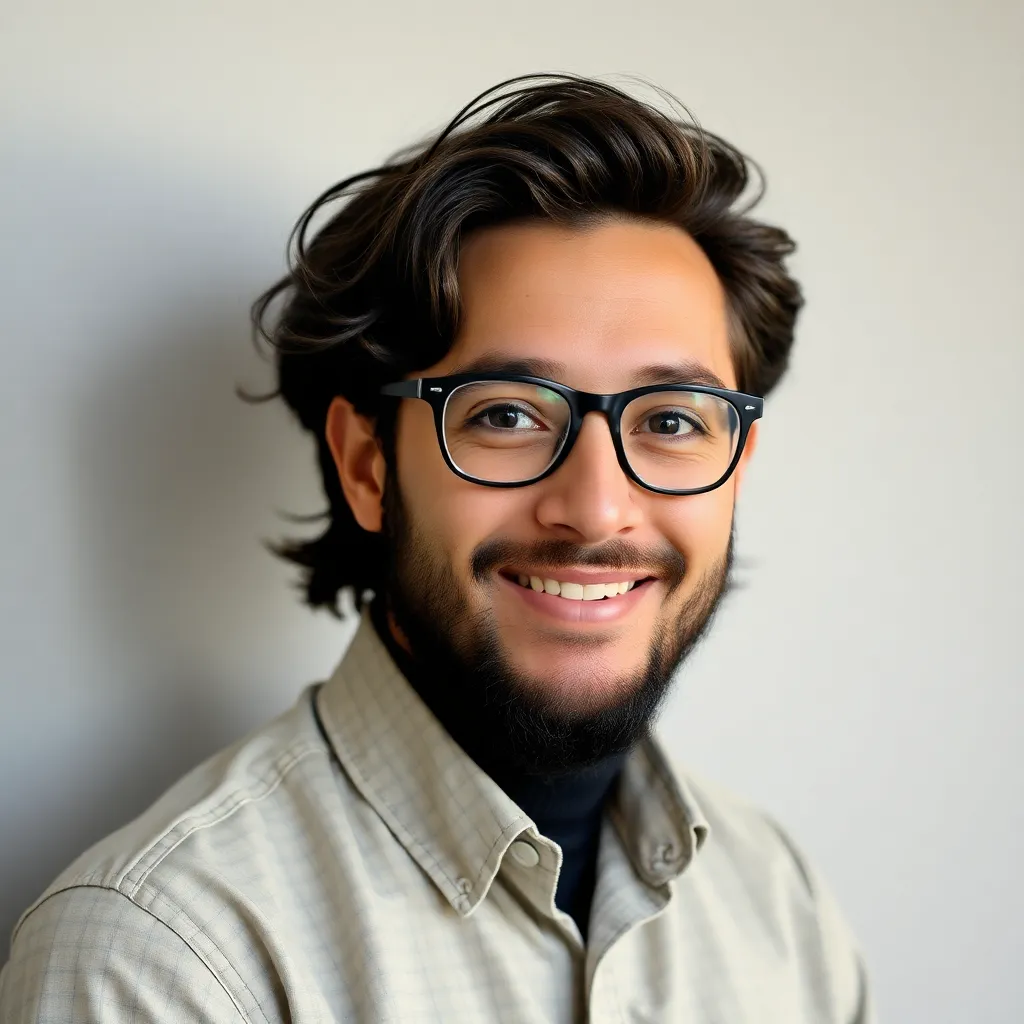
Onlines
Apr 17, 2025 · 6 min read

Table of Contents
PLTW 3.1.1 Inputs and Outputs: A Comprehensive Guide
PLTW (Project Lead The Way) is a renowned curriculum provider for STEM education, and its Computer Science course introduces foundational programming concepts. The 3.1.1 lesson on Inputs and Outputs is a crucial stepping stone in understanding how programs interact with users and the external world. This comprehensive guide delves into the core concepts of this lesson, providing explanations, examples, and practical applications to help students solidify their understanding. We'll explore inputs, outputs, variables, and data types – all vital components of successful programming.
Understanding Inputs and Outputs
At its heart, a computer program is a sequence of instructions designed to perform a specific task. To perform these tasks effectively, programs need to receive information (inputs) and produce results (outputs).
Inputs: The Foundation of Program Interaction
Inputs are the data a program receives to operate. These can come from various sources, including:
-
User Input: This is the most common input method. Users provide data via keyboards (text, numbers), mice (clicks, coordinates), or other input devices like touchscreens. In many programming languages, functions like
input()
orScanner
are used to capture user input. -
Files: Programs can read data from files, providing inputs such as text, numbers, images, or other data stored in various file formats (
.txt
,.csv
,.jpg
, etc.). File handling involves opening, reading, processing, and closing files. -
Sensors: In embedded systems and robotics, programs interact with sensors to receive input from the environment. Sensors can measure temperature, light, pressure, or other physical quantities, providing real-time data for the program to process.
-
Network Connections: Programs can receive data from networks (the internet, local networks). This includes receiving data from web servers, databases, or other connected devices.
Outputs: Presenting Program Results
Outputs are the results a program generates after processing inputs. Common output methods include:
-
Display to the Screen (Console): This is the simplest output method. Programs display text, numbers, or other data directly to the console (the command prompt or terminal). Functions like
print()
(in Python) orSystem.out.println()
(in Java) are commonly used. -
Files: Programs can write data to files, storing the results for later use. This allows for persistent storage of data generated by the program.
-
Graphical User Interfaces (GUIs): More sophisticated programs use GUIs to interact with users. GUIs provide visual elements (buttons, text fields, images) for input and output, presenting information in a user-friendly format.
-
Network Communication: Programs can send data over networks, such as sending emails, uploading data to servers, or transmitting data between devices.
Variables: Storing and Manipulating Data
Variables are essential for storing and manipulating data within a program. A variable is a named storage location in a computer's memory that holds a value.
-
Variable Declaration: Before using a variable, it usually needs to be declared. This involves specifying the variable's name and its data type (e.g., integer, floating-point number, string, boolean).
-
Variable Assignment: A value is assigned to a variable using an assignment operator (usually
=
). For example,age = 25
assigns the value 25 to the variable namedage
. -
Variable Types: Different data types represent different kinds of information.
- Integers (
int
): Whole numbers (e.g., 10, -5, 0). - Floating-Point Numbers (
float
,double
): Numbers with decimal points (e.g., 3.14, -2.5). - Strings (
str
): Sequences of characters (e.g., "Hello, world!"). - Booleans (
bool
): Represent truth values (True or False).
- Integers (
Data Types and Their Significance
Understanding data types is critical for writing correct and efficient programs. Each data type has specific properties and operations that can be performed on it. Using the wrong data type can lead to errors or unexpected results. For example, trying to perform arithmetic operations on a string will likely result in an error.
Practical Examples (Illustrative, not specific to PLTW 3.1.1 materials)
Let's illustrate inputs and outputs with simple Python code examples. Remember, these are illustrative; the exact syntax and functions may differ slightly depending on the PLTW curriculum's specific programming environment.
Example 1: Getting user input and displaying it
name = input("Enter your name: ")
print("Hello, " + name + "!")
This program prompts the user to enter their name, stores it in the name
variable, and then displays a personalized greeting.
Example 2: Calculating the area of a rectangle
length = float(input("Enter the length of the rectangle: "))
width = float(input("Enter the width of the rectangle: "))
area = length * width
print("The area of the rectangle is:", area)
This program gets the length and width of a rectangle as user input, calculates the area, and displays the result. Note the use of float()
to convert the input strings to floating-point numbers, allowing for decimal values.
Example 3: Working with files
This example is conceptually illustrative, as actual file I/O requires more extensive code, but captures the idea:
# (Simplified, conceptual illustration – actual file handling requires error checks and closing)
file = open("my_data.txt", "r") # Open a file for reading
data = file.read()
print("Data from the file:\n", data)
file.close()
#Writing to a file (conceptual)
output_data = "This is some new data."
file = open("output.txt", "w")
file.write(output_data)
file.close()
This illustrates reading data from and writing data to text files. In a real program, robust error handling (e.g., checking if the file exists, handling potential exceptions) is crucial.
Addressing Potential Challenges in PLTW 3.1.1
Students often face challenges in understanding:
-
Data Type Conversion: Converting between different data types (e.g., string to integer) is essential but can be error-prone if not handled correctly.
-
Input Validation: Ensuring that the input provided by the user is valid and in the expected format is crucial to prevent errors. For instance, a program expecting a number should handle cases where the user inputs text.
-
Error Handling: Programs should gracefully handle errors (e.g., file not found, invalid input) rather than crashing.
-
Debugging: Identifying and fixing errors (bugs) in code is an essential skill. Using debugging tools and techniques to track down errors is crucial for successful programming.
Advanced Concepts (Beyond the Basics)
While PLTW 3.1.1 focuses on fundamental inputs and outputs, more advanced concepts build upon these foundations:
-
Command-Line Arguments: Programs can receive input directly from the command line when they are executed.
-
Modular Programming: Breaking down large programs into smaller, manageable modules (functions, classes) improves code organization and readability.
-
Object-Oriented Programming (OOP): OOP is a powerful programming paradigm that uses objects to model real-world entities. It is frequently used in more advanced programming projects.
-
Data Structures: More advanced data structures (arrays, lists, dictionaries) allow efficient storage and manipulation of large amounts of data.
Conclusion
Understanding inputs and outputs is fundamental to programming. PLTW's 3.1.1 lesson provides a strong foundation for students to grasp this crucial concept. By mastering the concepts of input, output, variables, and data types, students lay a solid base for tackling more complex programming challenges in subsequent lessons and future projects. Remember to practice consistently, experiment with different input and output methods, and always strive to write clean, well-documented, and robust code. This guide provides a comprehensive overview and hopefully enhances your understanding and ability to tackle the PLTW 3.1.1 activities effectively. Remember to consult your course materials and instructor for specific details and guidance related to your curriculum's requirements.
Latest Posts
Latest Posts
-
The Services Acquisition Process Begins With Planning
Apr 19, 2025
-
9 3 3 Packet Tracer Hsrp Configuration Guide
Apr 19, 2025
-
The Guideline For Programming Hypertrophy Is
Apr 19, 2025
-
Lets Focus On Pathos Answer Key
Apr 19, 2025
-
Pirate Riddle 2 Dividing Fractions Answer Key
Apr 19, 2025
Related Post
Thank you for visiting our website which covers about Pltw 3.1.1 Inputs And Outputs Answer Key . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.