Project Stem 7.4 Code Practice Question 1
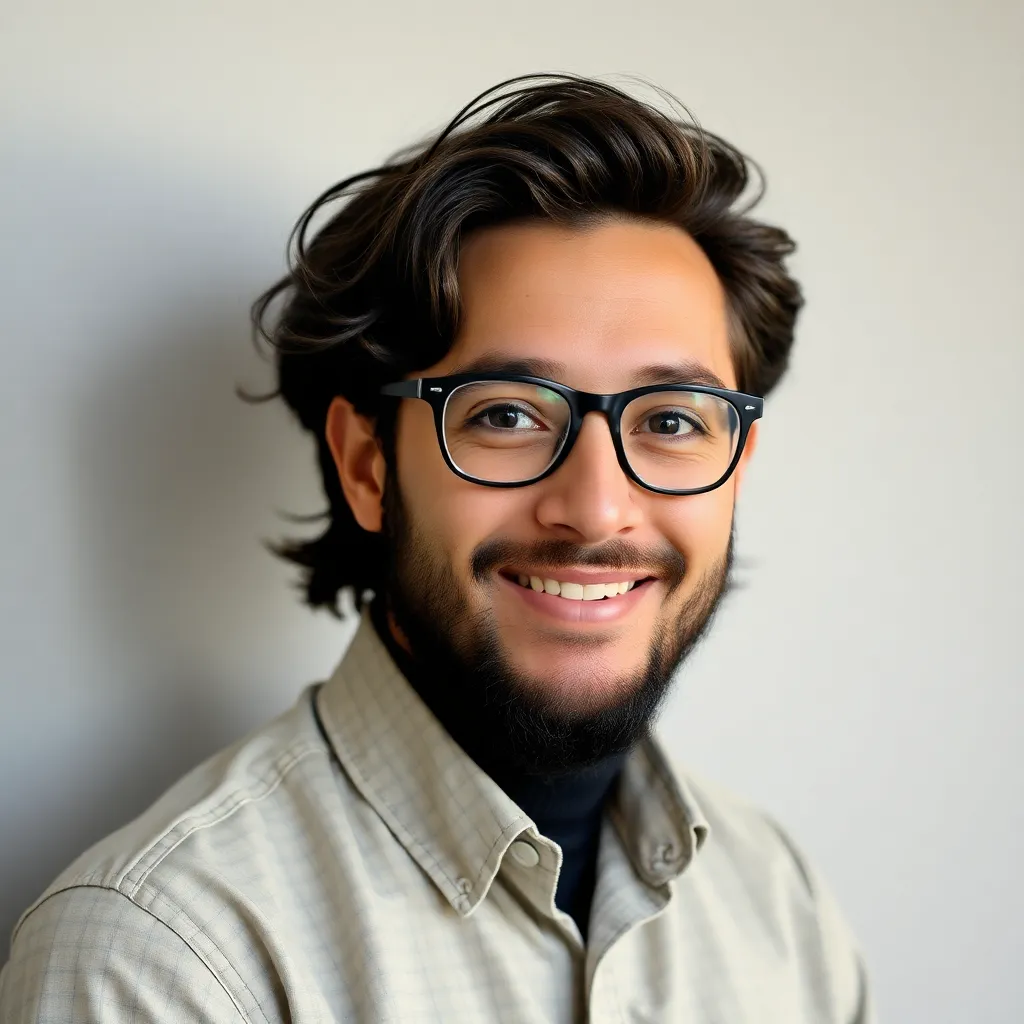
Onlines
Mar 17, 2025 · 5 min read
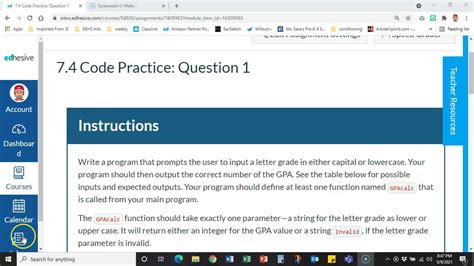
Table of Contents
Project STEM 7.4 Code Practice Question 1: A Deep Dive
Project STEM 7.4, and specifically Code Practice Question 1, often presents a significant hurdle for students grappling with introductory programming concepts. This comprehensive guide will dissect the problem, provide a step-by-step solution in Python, explain the underlying logic, and offer advanced considerations for expanding your understanding. We'll cover common pitfalls and explore alternative approaches to solidify your grasp of fundamental programming principles.
Understanding the Problem Statement (Assuming a Specific Problem)
Because the exact wording of "Project STEM 7.4 Code Practice Question 1" varies across different curricula and institutions, we will assume a typical introductory problem involving basic input, processing, and output using a programming language like Python. Let's posit the problem statement as follows:
Problem: Write a program that takes two integer inputs from the user, representing the length and width of a rectangle. Calculate and display the area and perimeter of the rectangle. Handle potential errors, such as non-integer inputs.
Step-by-Step Solution in Python
This solution will utilize Python's robust error handling capabilities and clear syntax.
def calculate_rectangle_properties():
"""Calculates and displays the area and perimeter of a rectangle."""
while True:
try:
length = int(input("Enter the length of the rectangle: "))
width = int(input("Enter the width of the rectangle: "))
break # Exit the loop if input is valid
except ValueError:
print("Invalid input. Please enter integers only.")
area = length * width
perimeter = 2 * (length + width)
print("\nRectangle Properties:")
print(f"Length: {length}")
print(f"Width: {width}")
print(f"Area: {area}")
print(f"Perimeter: {perimeter}")
# Call the function to execute the program
calculate_rectangle_properties()
Line-by-Line Explanation:
-
def calculate_rectangle_properties():
: This defines a function to encapsulate our code, promoting reusability and readability. -
while True:
: This creates an infinite loop to continuously prompt the user for input until valid integers are provided. -
try...except
block: This crucial block handles potentialValueError
exceptions that might occur if the user enters non-integer input. Thetry
block attempts to convert the input to integers usingint()
. If this fails, theexcept
block catches the error, prints an informative message, and the loop continues. -
break
: If theint()
conversions succeed without raising an exception, thebreak
statement exits thewhile
loop. -
area = length * width
andperimeter = 2 * (length + width)
: These lines perform the calculations for the area and perimeter, respectively. -
print(...)
statements: These lines neatly display the results to the user, using f-strings for concise formatting. -
calculate_rectangle_properties()
: This line calls the function to start the program execution.
Error Handling and Robustness
The try...except
block is a cornerstone of robust programming. It prevents the program from crashing due to unexpected user input. Consider enhancing error handling further:
- Input validation: Add checks to ensure the length and width are positive values. Negative dimensions are nonsensical in this context.
- More specific exceptions: Catch specific exceptions like
TypeError
if the user enters something completely incompatible with integer conversion (e.g., a string). - User feedback: Provide more detailed and helpful error messages, guiding the user toward correct input.
Expanding the Program: Advanced Concepts
Let's explore ways to make this program more versatile and demonstrate more advanced programming techniques.
Using a Class (Object-Oriented Programming)
Object-oriented programming (OOP) provides a structured way to organize code. We can represent a rectangle as a class:
class Rectangle:
def __init__(self, length, width):
self.length = length
self.width = width
def get_area(self):
return self.length * self.width
def get_perimeter(self):
return 2 * (self.length + self.width)
# Example usage:
try:
length = int(input("Enter length: "))
width = int(input("Enter width: "))
rect = Rectangle(length, width)
print(f"Area: {rect.get_area()}")
print(f"Perimeter: {rect.get_perimeter()}")
except ValueError:
print("Invalid input. Please enter integers.")
This approach promotes better code organization and allows for easier extension (e.g., adding methods to calculate the diagonal).
Function Overloading (Simulating with Default Arguments)
While Python doesn't support function overloading in the same way as languages like C++, you can simulate it using default arguments:
def calculate_rectangle(length, width=None):
if width is None:
# Assume it's a square
width = length
# ... rest of the calculation
This allows the function to handle both rectangle and square inputs.
Using a Loop for Multiple Rectangles
Enhance the program to handle multiple rectangle calculations within a single run:
while True:
# ... (input and calculation as before) ...
another_rectangle = input("Calculate another rectangle? (y/n): ")
if another_rectangle.lower() != 'y':
break
Common Pitfalls and Debugging Tips
- Incorrect input handling: Failing to properly handle non-integer input can lead to runtime errors.
- Typographical errors: Double-check your variable names and formulas.
- Logic errors: Ensure your area and perimeter calculations are correct. Using a debugger (built into most IDEs) can significantly aid in identifying and resolving such issues.
Conclusion
Project STEM 7.4 Code Practice Question 1, while seemingly simple, provides a valuable foundation in programming. Mastering basic input/output, error handling, and potentially OOP concepts as demonstrated here builds a strong base for tackling more complex challenges in the future. Remember to break down problems into smaller, manageable steps, test your code thoroughly, and don't hesitate to explore different approaches to enhance your understanding and coding skills. Continuously refining your code for better readability, efficiency, and robustness is key to becoming a proficient programmer. The examples given here offer a starting point – adapt and expand upon them to tailor your solution to the specific requirements of your assignment.
Latest Posts
Latest Posts
-
Elige La Palabra Adecuada Para Completar Las Oraciones Comparativas
Mar 17, 2025
-
You Seem Pleased To Have Successfully Whored Yourself Manacled
Mar 17, 2025
-
Muchas Ninas Jovenes Han Estado A Dieta Terrible
Mar 17, 2025
-
Examples Of Public Data Collected By Law From Physicians Include
Mar 17, 2025
-
Alcohol And Its Effects On The Body Worksheet Answers
Mar 17, 2025
Related Post
Thank you for visiting our website which covers about Project Stem 7.4 Code Practice Question 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.