The Index 1 Identifies The Last Element In A List
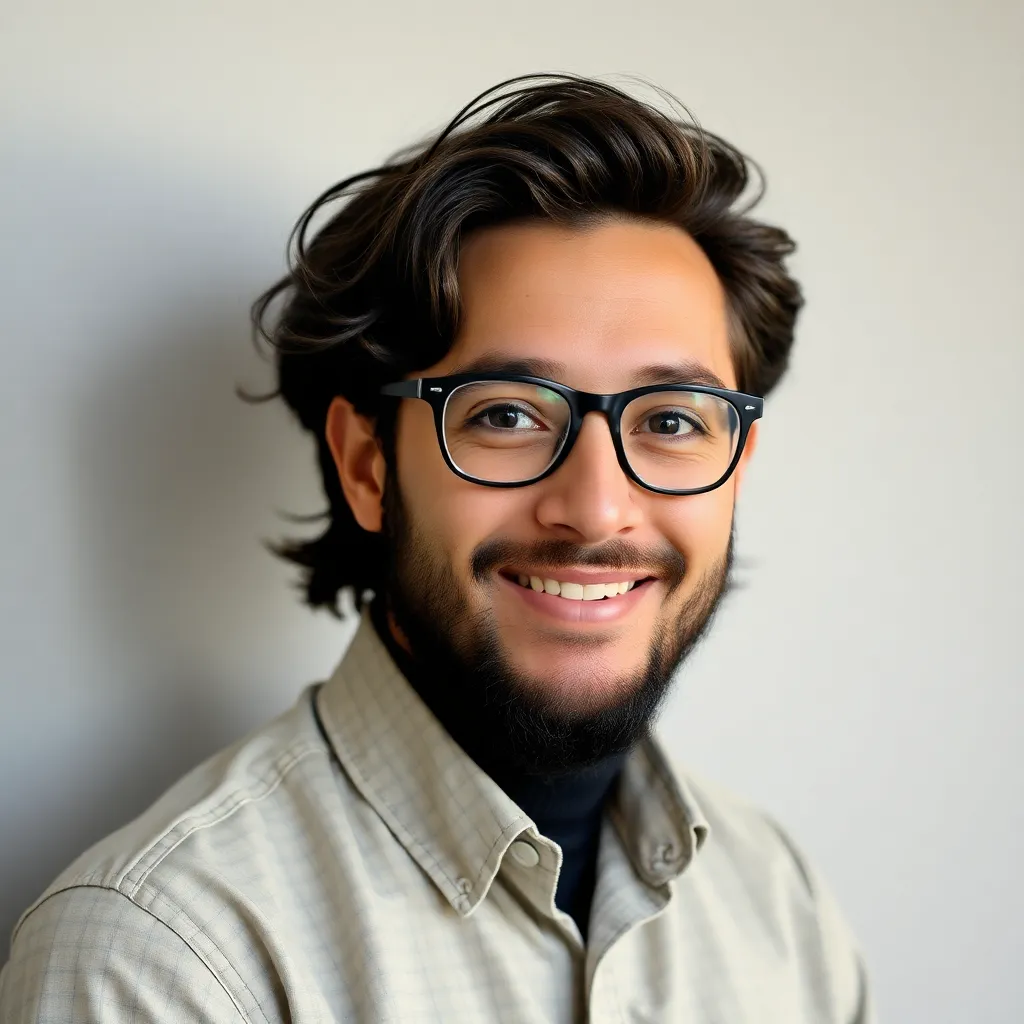
Onlines
May 08, 2025 · 5 min read
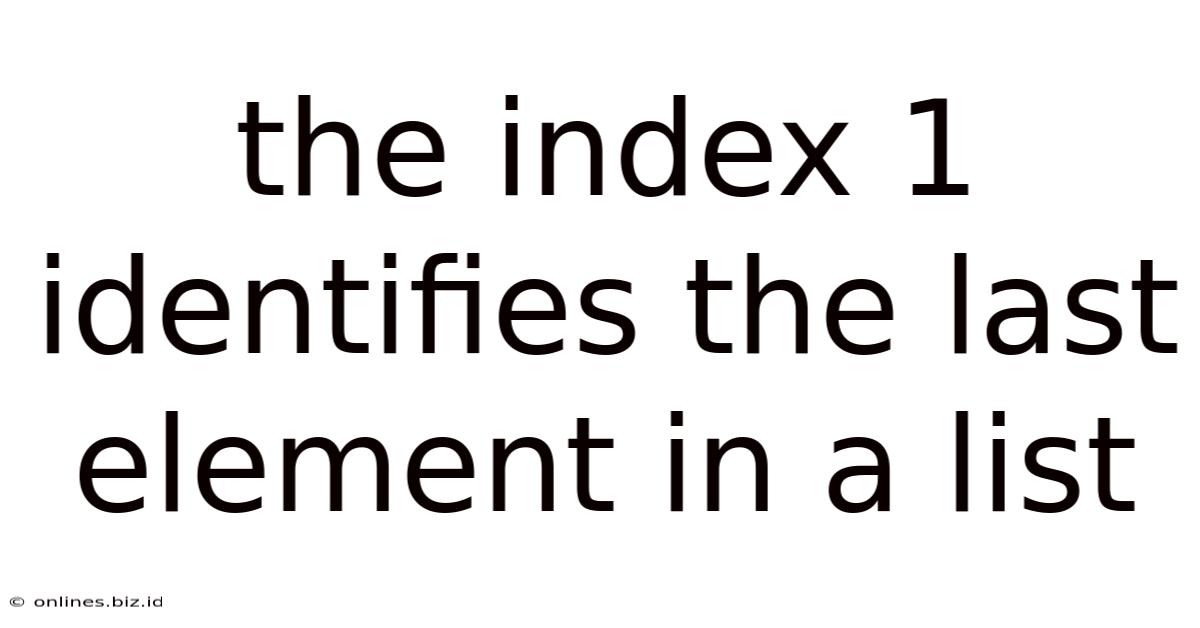
Table of Contents
The Index 1 Identifies the Last Element in a List: A Deep Dive into Indexing in Programming
The statement "the index 1 identifies the last element in a list" is incorrect in most common programming languages. This misunderstanding stems from a confusion around how indexing works in various programming paradigms. Understanding indexing is crucial for efficient and error-free programming. This article will explore the nuances of list indexing, debunking the misconception and providing a clear understanding of how to access elements within lists and other data structures.
Understanding List Indexing: The Zero-Based Index
The vast majority of programming languages, including Python, Java, C++, JavaScript, and many others, utilize a zero-based indexing system. This means the first element in a list is located at index 0, the second element at index 1, and so on. The last element's index is always one less than the total number of elements in the list.
Let's illustrate this with an example in Python:
my_list = ["apple", "banana", "cherry", "date"]
print(len(my_list)) # Output: 4 (Number of elements)
print(my_list[0]) # Output: apple (First element, index 0)
print(my_list[1]) # Output: banana (Second element, index 1)
print(my_list[3]) # Output: date (Last element, index 3)
As you can see, the last element "date" is accessed using the index 3, not 1. The index 1 accesses the second element, "banana". This zero-based indexing is a fundamental concept in programming and understanding it is key to avoiding common off-by-one errors.
Why Zero-Based Indexing?
The choice of zero-based indexing is not arbitrary. It offers several advantages:
-
Simplicity in Memory Management: Computers work with memory addresses, which are essentially numerical locations. The first element in a data structure naturally resides at the starting memory address. Indexing from zero directly aligns with this memory representation, simplifying memory access calculations.
-
Consistent Pointer Arithmetic: Many operations involve calculating the memory location of elements relative to the start. Zero-based indexing makes these calculations straightforward. If indexing started from 1, extra subtractions would be necessary, increasing computational overhead.
-
Mathematical Elegance: Many algorithms and formulas in computer science are naturally expressed using zero-based indexing. For example, calculating the position of an element in a two-dimensional array often involves simpler formulas with zero-based indexing.
-
Improved Code Readability: While it may seem counterintuitive at first, experienced programmers find zero-based indexing surprisingly intuitive and enhances code clarity over time.
Common Indexing Errors and How to Avoid Them
Incorrect indexing is a frequent source of bugs. Here are some common mistakes and how to prevent them:
-
Off-by-One Errors: These are the most prevalent errors. Remember that the index of the last element is always
len(list) - 1
. Double-checking your index calculations before accessing elements can significantly reduce these errors. -
Negative Indexing: Many languages support negative indexing, allowing access to elements from the end of the list.
my_list[-1]
accesses the last element,my_list[-2]
accesses the second-to-last element, and so on. While convenient, misuse of negative indexing can lead to errors if not carefully handled. -
Index Out of Bounds Errors: Attempting to access an element using an index that is outside the valid range (less than 0 or greater than or equal to the length of the list) will result in an
IndexError
in many languages. Always validate your indices before accessing list elements to avoid this.
Beyond Lists: Indexing in Other Data Structures
The concept of indexing isn't limited to lists. Other data structures like arrays, tuples (in languages like Python), and strings also utilize indexing to access their elements. However, the specific indexing mechanisms might differ slightly depending on the data structure and the programming language.
-
Arrays: Arrays typically use zero-based indexing similar to lists.
-
Tuples: Tuples in Python, which are immutable sequences, also use zero-based indexing.
-
Strings: Strings are sequences of characters, and they too employ zero-based indexing. You can access individual characters using their indices.
Practical Applications and Advanced Indexing Techniques
Indexing plays a critical role in many algorithms and programming tasks.
-
Searching Algorithms: Linear search and binary search heavily rely on accessing elements by their indices.
-
Sorting Algorithms: Many sorting algorithms, like bubble sort and insertion sort, use indexing to compare and swap elements.
-
Data Manipulation: Indexing enables tasks like modifying individual elements, inserting or deleting elements at specific positions, and slicing parts of a list or array.
-
Multidimensional Arrays: Multidimensional arrays (matrices) use multiple indices to specify the row and column positions of elements.
-
Slicing: Many languages support slicing, which allows accessing a sub-sequence of elements using a range of indices. For instance,
my_list[1:3]
in Python would return a new list containing elements at indices 1 and 2.
Debugging Indexing Problems
When debugging code involving list or array indexing, consider these strategies:
-
Print Statements: Insert
print
statements to display the values of indices and the lengths of lists before accessing elements. This helps pinpoint errors in index calculations. -
Debuggers: Use a debugger to step through your code line by line, observing the values of variables and inspecting the state of your data structures.
-
Code Reviews: Have another programmer review your code to identify potential indexing errors. A fresh pair of eyes can often spot subtle mistakes.
-
Test Cases: Write unit tests to thoroughly exercise your code with various input values and test edge cases to verify the correctness of indexing operations.
Conclusion: Mastering Indexing for Efficient Programming
The accurate use of indexing is fundamental to efficient and error-free programming. The common misconception that index 1 represents the last element is incorrect in most programming languages due to the prevalent use of zero-based indexing. Understanding this core principle, along with common indexing errors and debugging techniques, will greatly enhance your programming skills and lead to more robust and maintainable code. Always remember to verify index calculations, handle potential IndexError
exceptions, and leverage debugging tools to identify and resolve indexing-related issues. With a solid grasp of indexing, you can confidently navigate complex data structures and build sophisticated applications.
Latest Posts
Latest Posts
-
Ap Bio Unit 6 Progress Check Frq
May 09, 2025
-
Why Is Modeling Optimism Helpful To Families
May 09, 2025
-
House On Mango Street One Pager
May 09, 2025
-
Summary Of To Kill A Mockingbird Chapter 29
May 09, 2025
-
Algeria My Father Writes To My Mother
May 09, 2025
Related Post
Thank you for visiting our website which covers about The Index 1 Identifies The Last Element In A List . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.