Translating An Algorithm Into A Programming Language Is Called
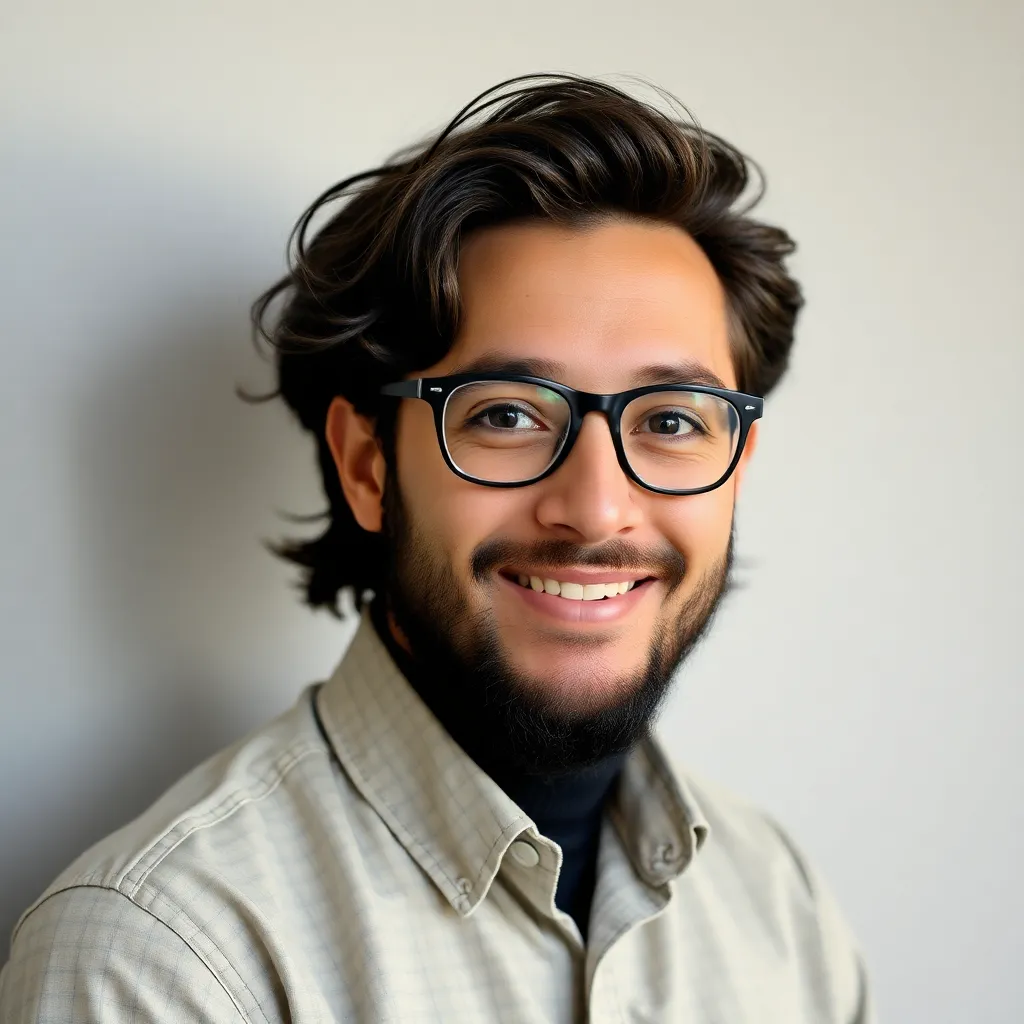
Onlines
May 06, 2025 · 6 min read

Table of Contents
Translating an Algorithm into a Programming Language is Called: Implementation
The process of transforming a theoretical algorithm into a functional program within a specific programming language is called implementation. This crucial step bridges the gap between abstract problem-solving and tangible, executable code. While seemingly straightforward, algorithm implementation involves a multitude of considerations that directly impact the efficiency, readability, and overall success of the final software. This article delves deep into the intricacies of algorithm implementation, exploring various aspects, challenges, and best practices.
Understanding Algorithms and Their Role
Before diving into implementation, let's briefly revisit the concept of algorithms. An algorithm is a finite sequence of well-defined, computer-implementable instructions, typically to solve a class of problems or to perform a computation. It's a step-by-step procedure, much like a recipe, that takes an input, processes it according to specified rules, and produces a desired output. Algorithms are the backbone of any computer program; they define the what and how of a program's functionality.
Key Characteristics of a Good Algorithm
A well-designed algorithm possesses several key characteristics:
- Correctness: It produces the intended output for all valid inputs.
- Efficiency: It utilizes computational resources (time and memory) optimally. This is often measured using Big O notation.
- Readability: It's easy to understand and maintain, especially important for collaborative projects.
- Robustness: It handles unexpected inputs or errors gracefully, avoiding crashes or incorrect outputs.
- Generality: It can be adapted to solve similar problems with minimal modification.
The Implementation Process: From Algorithm to Code
Implementing an algorithm involves translating its abstract steps into the specific syntax and semantics of a chosen programming language. This is more than just a mechanical translation; it requires a deep understanding of both the algorithm and the target language.
Steps in Algorithm Implementation
-
Understanding the Algorithm: This is the critical first step. Thoroughly analyze the algorithm's logic, data structures, and the relationships between different parts. Ensure you grasp the algorithm's purpose and how it achieves its goals.
-
Choosing a Programming Language: The choice of programming language depends on several factors, including the nature of the problem, performance requirements, developer expertise, and available libraries. Some languages excel at specific tasks: Python might be preferred for data science due to its rich libraries, while C++ might be chosen for performance-critical applications.
-
Data Structure Selection: Algorithms often rely on specific data structures (arrays, linked lists, trees, graphs, etc.) to store and manipulate data efficiently. Choosing the appropriate data structure is crucial for optimization. The algorithm's efficiency is heavily influenced by the data structure's characteristics.
-
Code Writing: This is where the actual translation takes place. Break down the algorithm's steps into smaller, manageable chunks of code, ensuring each piece accurately reflects the corresponding step. Pay meticulous attention to syntax, variable naming, and code formatting for readability.
-
Testing and Debugging: Once the code is written, thorough testing is essential to verify its correctness and identify any bugs. Use a variety of test cases, including edge cases and boundary conditions, to ensure robustness. Debugging tools and techniques become invaluable during this stage.
-
Optimization: After initial testing, analyze the code's performance. Identify bottlenecks and areas for improvement. Optimization techniques might involve using more efficient data structures, algorithms, or low-level language features.
-
Documentation: Well-documented code is crucial for maintainability and collaboration. Clearly describe the algorithm's purpose, functionality, input/output specifications, and any assumptions made during implementation.
Challenges in Algorithm Implementation
Implementing algorithms is rarely a straightforward process. Several challenges can arise:
-
Complexity: Complex algorithms with intricate logic can be difficult to translate accurately into code. Breaking down the algorithm into smaller, modular functions can greatly improve manageability.
-
Data Structures: Selecting the appropriate data structure requires careful consideration. An inefficient choice can severely impact performance.
-
Error Handling: Robust error handling is crucial. Unexpected inputs or errors should be handled gracefully to prevent program crashes.
-
Debugging: Identifying and fixing bugs can be time-consuming, especially in large and complex programs. Systematic debugging techniques and the use of debugging tools are vital.
-
Performance Optimization: Achieving optimal performance often requires advanced optimization techniques, such as algorithm optimization, data structure optimization, and code profiling.
Best Practices for Algorithm Implementation
To ensure successful and efficient algorithm implementation, follow these best practices:
-
Modular Design: Break down the algorithm into smaller, independent modules or functions. This enhances readability, maintainability, and reusability.
-
Meaningful Variable Names: Use descriptive variable names that clearly indicate their purpose. This improves code readability and understandability.
-
Code Comments: Add comments to explain complex parts of the code and clarify the algorithm's logic. This aids in understanding and future maintenance.
-
Testing and Validation: Rigorous testing using diverse test cases is essential to ensure the code's correctness and robustness. Employ unit testing, integration testing, and system testing.
-
Version Control: Use a version control system (like Git) to track changes, manage different versions of the code, and facilitate collaboration.
-
Code Reviews: Have other developers review your code to identify potential errors, improve readability, and ensure adherence to coding standards.
-
Profiling and Optimization: Profile the code to identify performance bottlenecks and apply optimization techniques to enhance efficiency.
Examples of Algorithm Implementation Across Languages
Let's consider a simple example: implementing an algorithm to find the maximum element in an array. This can be implemented in various languages, highlighting the differences in syntax but underlying similarity in logic.
Python:
def find_max(arr):
"""Finds the maximum element in an array."""
if not arr:
return None # Handle empty array case
max_element = arr[0]
for element in arr:
if element > max_element:
max_element = element
return max_element
my_array = [1, 5, 2, 8, 3]
max_value = find_max(my_array)
print(f"The maximum element is: {max_value}")
C++:
#include
#include
#include //for std::max_element
int findMax(const std::vector& arr) {
if (arr.empty()) {
return -1; // Handle empty array case
}
return *std::max_element(arr.begin(), arr.end());
}
int main() {
std::vector myArray = {1, 5, 2, 8, 3};
int maxValue = findMax(myArray);
std::cout << "The maximum element is: " << maxValue << std::endl;
return 0;
}
Java:
import java.util.Arrays;
class FindMax {
public static int findMax(int[] arr) {
if (arr.length == 0) {
return -1; // Handle empty array case
}
return Arrays.stream(arr).max().getAsInt();
}
public static void main(String[] args) {
int[] myArray = {1, 5, 2, 8, 3};
int maxValue = findMax(myArray);
System.out.println("The maximum element is: " + maxValue);
}
}
These examples demonstrate how the same algorithm can be implemented in different languages, showcasing the importance of understanding the algorithm's core logic, independent of the chosen programming language's syntax.
Conclusion
Translating an algorithm into a programming language, or implementation, is a fundamental process in software development. It requires a deep understanding of both algorithmic principles and programming language specifics. By following best practices, addressing potential challenges, and utilizing efficient techniques, developers can create robust, efficient, and maintainable software that effectively solves real-world problems. The choice of programming language, data structures, and optimization strategies all contribute to the overall success and performance of the implemented algorithm. Careful planning, rigorous testing, and continuous refinement are essential for creating high-quality software based on well-defined algorithms.
Latest Posts
Latest Posts
-
Which Historical Event Was Greatly Responsible For Global Stratification
May 06, 2025
-
List The Standards A Dental Material Must Meet
May 06, 2025
-
How To Write A Food Stamp Letter
May 06, 2025
-
In A Reality Therapy Group The Leader
May 06, 2025
-
You Are Transporting A Stable Patient With A Possible Pneumothorax
May 06, 2025
Related Post
Thank you for visiting our website which covers about Translating An Algorithm Into A Programming Language Is Called . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.