Which Data Type Stores Only One Of Two Values
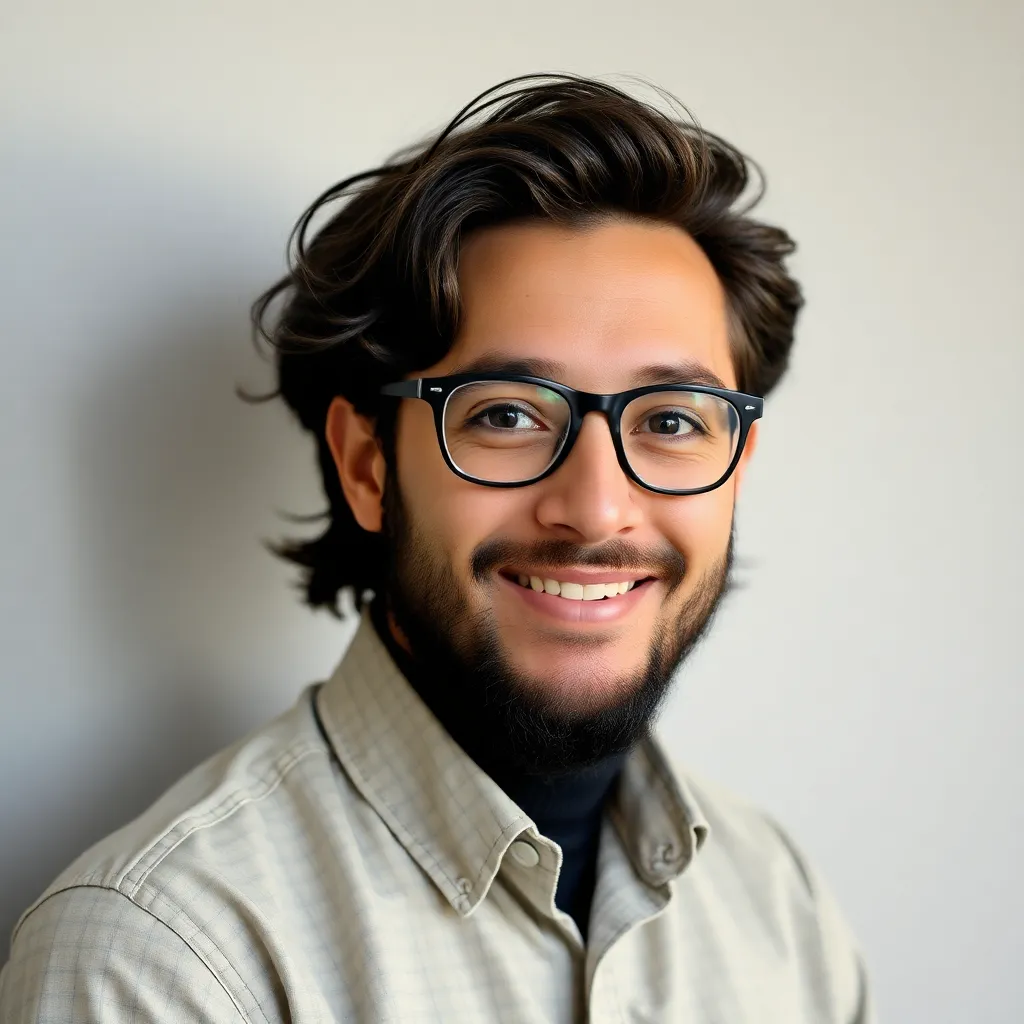
Onlines
Apr 05, 2025 · 6 min read
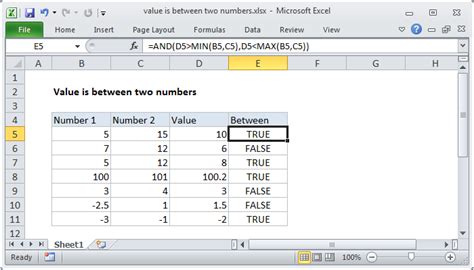
Table of Contents
Which Data Type Stores Only One of Two Values? A Deep Dive into Boolean Data Types
The question, "Which data type stores only one of two values?" has a straightforward answer: the Boolean data type. However, understanding Boolean data types goes far beyond this simple definition. This comprehensive guide explores Boolean data types across various programming languages, their applications, advantages, limitations, and best practices for effective use in your projects. We'll delve into the nuances of how Booleans function, their importance in logic, and how they underpin complex algorithms and data structures.
Understanding Boolean Data Types: The Foundation of Logic
At its core, a Boolean data type can hold only one of two possible values: true or false. These values represent the fundamental concepts of truth and falsehood, forming the building blocks of logical operations and conditional statements in programming. Think of a light switch: it's either on (true) or off (false); there's no in-between state. This simplicity belies their incredible power and versatility in shaping the behavior of software.
Representation in Different Programming Languages
While the underlying concept remains consistent, the specific syntax and implementation of Boolean data types vary slightly across different programming languages:
- Python: Uses the keywords
True
andFalse
(case-sensitive). - JavaScript: Employs the keywords
true
andfalse
(case-sensitive). - Java: Utilizes the keywords
true
andfalse
(case-sensitive). - C++: Uses the keywords
true
andfalse
(case-sensitive), often implicitly converted from integer values (0 for false, non-zero for true). - C#: Similar to Java and JavaScript, using
true
andfalse
. - SQL: Frequently uses
TRUE
,FALSE
, or1
and0
depending on the specific database system.
The consistency across languages underscores the universality of the Boolean concept. Regardless of the specific keyword used, the core functionality – representing a binary state – remains the same.
Beyond True and False: Practical Applications of Boolean Data Types
Boolean data types are not just theoretical concepts; they're fundamental to almost every aspect of software development. Their applications are extensive and span a wide range of functionalities:
1. Conditional Statements and Control Flow
This is arguably the most common use of Boolean values. Conditional statements, such as if
, else if
, and else
blocks, rely heavily on Boolean expressions to determine which code path to execute. For example:
is_adult = True
if is_adult:
print("You can vote!")
else:
print("You are not yet eligible to vote.")
This simple example demonstrates how a Boolean variable (is_adult
) controls the flow of execution, altering the output based on its truth value.
2. Flags and Status Indicators
Boolean variables often serve as flags or status indicators within a program. They efficiently track the state of various components or processes. For example, a program might use a Boolean variable to indicate whether a file has been saved, a network connection is active, or a user is logged in:
let fileSaved = false;
// ... some code that saves the file ...
fileSaved = true;
if (fileSaved) {
console.log("File saved successfully!");
}
The fileSaved
flag clearly communicates the state of the file saving operation.
3. Boolean Logic and Operations
Boolean algebra, a branch of algebra dealing with logical operations, forms the backbone of many algorithms and data structures. Boolean operators (AND, OR, NOT, XOR) combine Boolean values to produce new Boolean results. These operators are crucial for creating complex conditional logic and decision-making processes:
- AND: Returns
true
only if both operands aretrue
. - OR: Returns
true
if at least one operand istrue
. - NOT: Inverts the value of its operand (
true
becomesfalse
, and vice versa). - XOR (Exclusive OR): Returns
true
if exactly one operand istrue
.
Understanding and applying these operators is essential for developing robust and efficient software.
4. Data Validation and Error Handling
Boolean variables are invaluable in data validation and error handling. They can indicate whether data meets certain criteria or if an operation was successful. This allows programs to respond appropriately to different scenarios, preventing unexpected crashes or incorrect results.
boolean isValidEmail = validateEmail(email);
if (isValidEmail) {
// Proceed with email processing
} else {
// Handle invalid email
}
The isValidEmail
variable provides a clear indication of the email's validity, guiding the program's behavior.
5. Database Design and Querying
In database systems, Boolean values are frequently used to represent attributes like "active/inactive," "enabled/disabled," or "true/false" flags within database tables. This facilitates efficient querying and data manipulation. For instance, a database might store a "subscribed" flag as a Boolean to indicate whether a user is subscribed to a newsletter.
Advantages and Limitations of Boolean Data Types
While highly useful, Boolean data types have certain advantages and limitations:
Advantages:
- Simplicity: Their binary nature makes them easy to understand and use.
- Efficiency: They require minimal storage space.
- Clarity: They improve code readability and maintainability by clearly representing true/false conditions.
- Foundation for Logic: They are the bedrock of logical operations and conditional statements.
Limitations:
- Limited Values: They only represent two states, which may not suffice for situations requiring more nuanced representation. In such cases, enums or other data types might be more appropriate.
- Potential for Ambiguity: Without clear naming conventions, the meaning of Boolean variables can become ambiguous, especially in larger codebases.
Best Practices for Using Boolean Data Types
To maximize the effectiveness and clarity of Boolean variables, consider these best practices:
- Meaningful Names: Choose descriptive names that clearly convey the purpose of the Boolean variable (e.g.,
is_connected
,isValidData
,has_errors
). Avoid generic names likeflag1
orboolX
. - Consistency: Maintain consistent naming conventions (e.g., using
is_
prefix for Boolean variables). - Avoid Overuse: Don't overuse Booleans when other data types could provide a clearer representation.
- Comments: Add comments when the purpose of a Boolean variable is not immediately obvious.
- Careful Logic: Pay close attention to Boolean logic, ensuring that your AND, OR, NOT, and XOR operations achieve the desired results. Test thoroughly.
Conclusion: The Indispensable Boolean
Boolean data types, despite their simplicity, are indispensable components of modern programming. Their ability to represent binary states makes them crucial for controlling program flow, managing flags, performing logical operations, and enhancing code clarity. By understanding their properties, applications, and best practices, developers can harness the power of Boolean data types to build more efficient, robust, and maintainable software. Their seemingly simple nature belies their pervasive and critical role in the complex world of computer science. From simple conditional statements to sophisticated algorithms, the Boolean data type continues to be a fundamental building block in the construction of virtually all software applications. Remember to apply the best practices discussed to ensure clean, understandable, and efficient code. The careful and thoughtful use of Boolean data types is a hallmark of high-quality software development.
Latest Posts
Latest Posts
-
Summary Of Act 3 Scene 2
Apr 06, 2025
-
Blood At The Root Dominique Morisseau Pdf
Apr 06, 2025
-
Match The Appropriate Constitutional Convention Plan With Its Features
Apr 06, 2025
-
In Countries Like The Command Economy Predominates
Apr 06, 2025
-
The Vindication Of The Rights Of A Woman Summary
Apr 06, 2025
Related Post
Thank you for visiting our website which covers about Which Data Type Stores Only One Of Two Values . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.