1.06 Unit Test All About Jobs
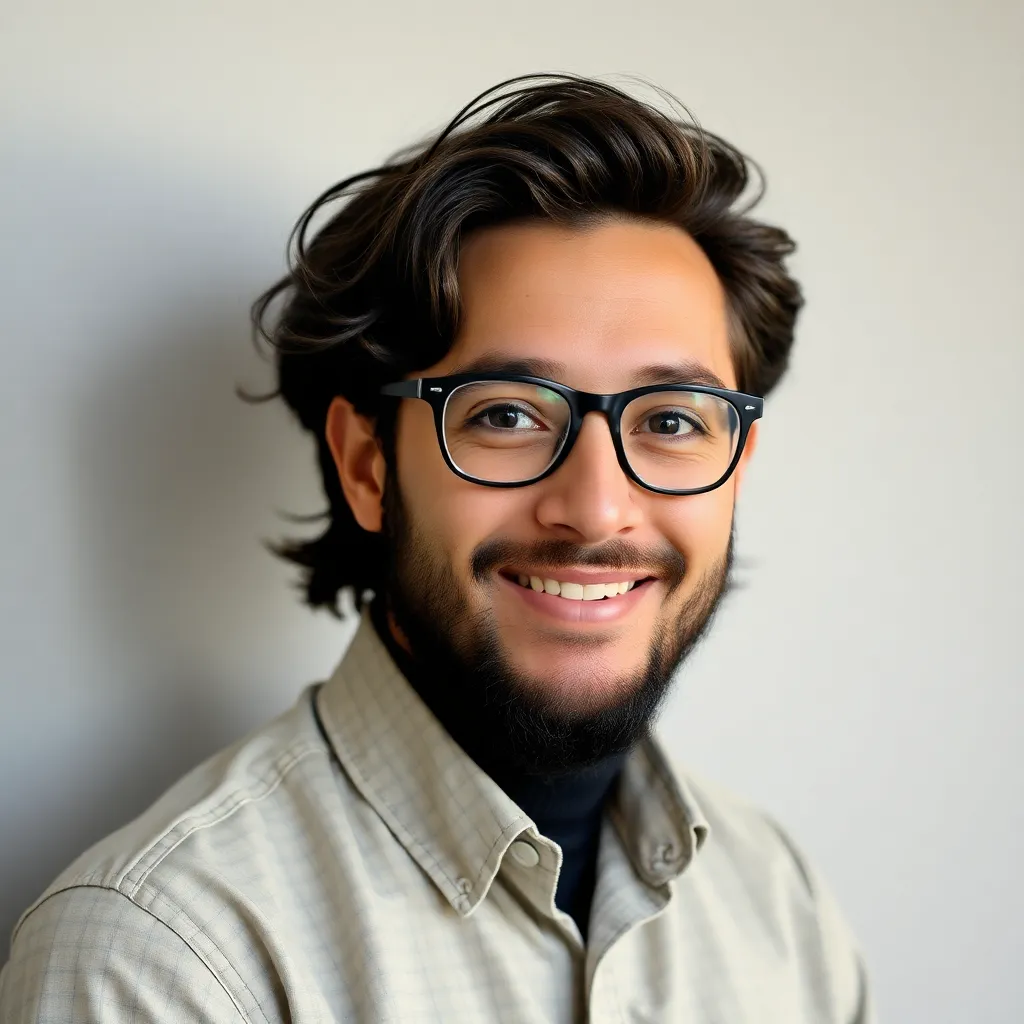
Onlines
Mar 25, 2025 · 6 min read
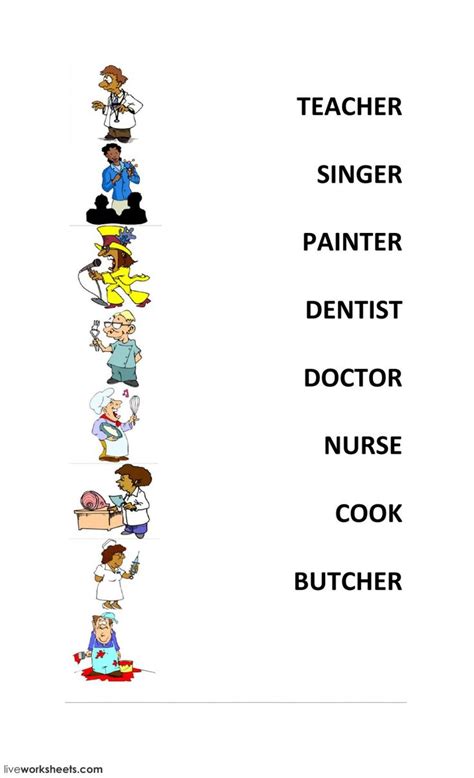
Table of Contents
1.06 Unit Test: All About Jobs - A Comprehensive Guide
The world of software testing is vast and intricate, with various methodologies and approaches designed to ensure the quality and reliability of software applications. Among these, unit testing plays a pivotal role, verifying the smallest testable parts – units – of an application in isolation. This article delves into the intricacies of unit testing, specifically focusing on a hypothetical scenario represented by "1.06 Unit Test" within the context of a job application process or a larger software project related to job functionalities. We'll explore best practices, common pitfalls, and how to effectively utilize unit tests to showcase your skills during job interviews or within a professional development setting.
Understanding the "1.06 Unit Test" Context
Let's assume "1.06 Unit Test" refers to a specific test case or a suite of tests within a larger project related to job management or recruitment. This could involve aspects such as:
- Job Posting Creation: Testing the functionality of creating new job postings, including validation of input fields (title, description, location, salary, etc.).
- Job Application Submission: Testing the process of applicants submitting their applications, including file uploads (resumes, cover letters), and data validation.
- Candidate Management: Testing functionalities related to managing candidate profiles, including searching, filtering, and updating candidate information.
- Interview Scheduling: Testing the system's ability to schedule and manage interviews, including sending reminders and managing availability.
- Offer Management: Testing the process of generating and managing job offers, including acceptance and rejection tracking.
Each of these functionalities would require a series of unit tests to ensure that every component works as expected in isolation. "1.06" could represent a specific test case within this larger framework, perhaps focused on a particular aspect of one of these functionalities. For example, it might test the validation of a specific field within a job posting form or a particular step in the application submission process.
Key Principles of Effective Unit Testing
Before diving into specific examples related to "1.06 Unit Test," let's establish some core principles of effective unit testing:
-
Isolation: Each unit test should focus on a single, isolated unit of code. Avoid testing multiple functionalities simultaneously. This makes it easier to pinpoint the source of errors.
-
Testability: Design your code with testability in mind. This often involves using dependency injection and keeping functions concise and focused.
-
Accuracy: Unit tests should be accurate and reliable. A failing test should always indicate a genuine bug, and a passing test should confidently confirm the correct functioning of the unit.
-
Maintainability: Keep your unit tests organized, well-documented, and easy to understand. This is crucial for long-term maintenance and collaboration.
-
Readability: Write tests that are clear, concise, and easy to follow. Use descriptive names for test methods and assertions.
Example Unit Tests for Job-Related Functionalities
Let's consider some concrete examples of unit tests within the "1.06 Unit Test" context, assuming we are using a testing framework like JUnit (Java) or pytest (Python).
1. Job Posting Creation:
// Example using JUnit (Java)
@Test
public void testCreateJobPosting_ValidInput() {
JobPosting jobPosting = new JobPosting("Software Engineer", "Develop amazing software", "New York", 100000);
assertTrue(jobPosting.getTitle().equals("Software Engineer"));
assertEquals(jobPosting.getDescription(), "Develop amazing software");
// ... other assertions ...
}
@Test(expected = IllegalArgumentException.class)
public void testCreateJobPosting_InvalidTitle() {
new JobPosting("", "Develop amazing software", "New York", 100000);
}
This example demonstrates two tests: one for a valid job posting and another that expects an exception for an invalid title.
2. Job Application Submission:
# Example using pytest (Python)
import pytest
def test_submit_application_valid_data():
application = {"name": "John Doe", "email": "[email protected]", "resume": "resume.pdf"}
result = submit_application(application)
assert result == "Application submitted successfully"
def test_submit_application_missing_data():
application = {"name": "John Doe"}
with pytest.raises(ValueError):
submit_application(application)
This Python example showcases tests for successful submission and handling of missing data.
3. Candidate Management:
// Example using JUnit (Java)
@Test
public void testFindCandidateByEmail() {
Candidate candidate = candidateRepository.findByEmail("[email protected]");
assertNotNull(candidate);
assertEquals(candidate.getName(), "Test User");
}
@Test
public void testUpdateCandidateProfile() {
Candidate candidate = candidateRepository.findById(1L).get();
candidate.setPhone("123-456-7890");
candidateRepository.save(candidate);
Candidate updatedCandidate = candidateRepository.findById(1L).get();
assertEquals(updatedCandidate.getPhone(), "123-456-7890");
}
This Java example illustrates tests for finding a candidate by email and updating their profile.
4. Interview Scheduling:
# Example using pytest (Python)
def test_schedule_interview_valid_timeslot():
interview = schedule_interview("John Doe", "2024-03-15 10:00")
assert interview["status"] == "Scheduled"
def test_schedule_interview_conflicting_timeslot():
with pytest.raises(ValueError):
schedule_interview("Jane Doe", "2024-03-15 10:00") # Assuming a conflict
These tests check for successful scheduling and handling of scheduling conflicts.
Advanced Unit Testing Techniques
For more complex scenarios, advanced techniques can be applied:
-
Mocking: Replacing external dependencies (databases, APIs) with mock objects to isolate the unit under test. This is crucial for testing interactions with external systems without actually interacting with them.
-
Stubbing: Providing pre-defined responses from mock objects to simulate specific scenarios.
-
Test-Driven Development (TDD): Writing unit tests before writing the code they test. This encourages modular design and ensures testability from the outset.
Applying Unit Tests in a Job Interview Context
Presenting your unit testing skills during a job interview can significantly enhance your candidature, demonstrating your understanding of software quality and best practices. Here are some strategies:
-
Prepare Examples: Have a few well-documented unit test examples ready to discuss. Choose examples relevant to the job description and the technologies used by the company.
-
Explain Your Approach: Don't just show the code; explain your thought process. Describe how you chose the tests, what edge cases you considered, and what your strategies were for handling different scenarios.
-
Discuss Frameworks: Show familiarity with popular unit testing frameworks (JUnit, pytest, NUnit, etc.). Explain your preferred framework and why.
-
Address Challenges: Be prepared to discuss challenges you encountered while writing unit tests. How did you overcome these challenges? What did you learn from the experience?
Conclusion: The Importance of 1.06 Unit Test and Beyond
"1.06 Unit Test," while a hypothetical representation, underscores the importance of meticulous unit testing in ensuring software quality and reliability, particularly within applications related to job management. Through rigorous testing, you can identify and fix bugs early in the development process, leading to a more robust and user-friendly application. Mastering unit testing not only enhances your technical skills but also demonstrates a commitment to quality and professionalism, making you a highly desirable candidate in any software development role. Remember that the principles and techniques discussed here extend far beyond the hypothetical "1.06 Unit Test" and are applicable to a wide range of software projects. Continuous learning and practice are essential for honing your unit testing skills and staying ahead in the ever-evolving world of software development.
Latest Posts
Latest Posts
-
Name The Strong Transparent Covering That Encases The Kidney
Mar 29, 2025
-
Gina Wilson All Things Algebra Unit 2 Homework 7
Mar 29, 2025
-
Text Can Be Used With A Diamond Symbol To Delineate
Mar 29, 2025
-
A Identify 3 Driving Risks Pictured Above
Mar 29, 2025
-
Add Curved Arrows To Draw Step 2 Of The Mechanism
Mar 29, 2025
Related Post
Thank you for visiting our website which covers about 1.06 Unit Test All About Jobs . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.