3.13 Unit Test How Important Ideas Are Expressed
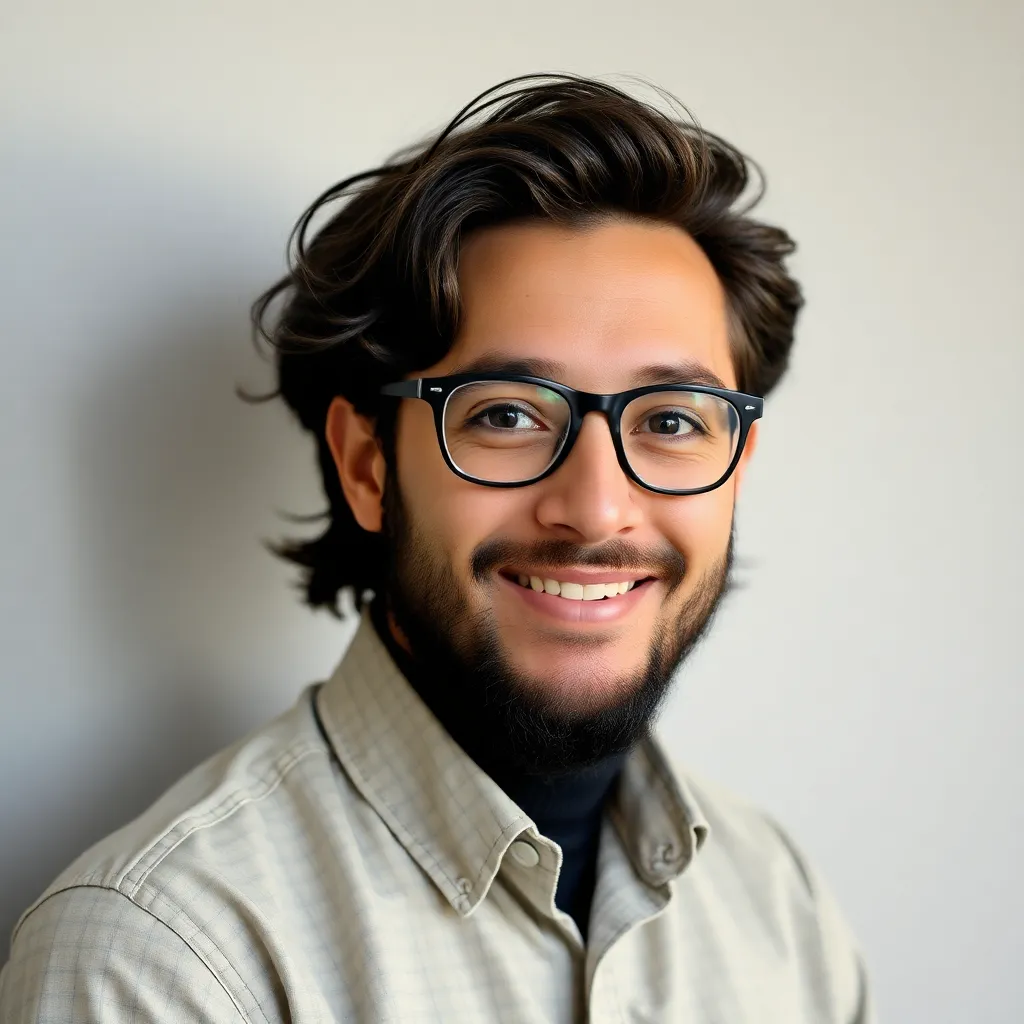
Onlines
Apr 01, 2025 · 6 min read
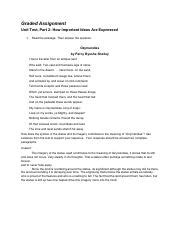
Table of Contents
3.13 Unit Test: How Important Ideas Are Expressed
Unit testing, a cornerstone of software development best practices, often gets overlooked or treated as an afterthought. However, understanding how effectively your unit tests express important ideas is crucial for building robust, maintainable, and reliable software. This goes beyond simply achieving 100% test coverage; it's about crafting tests that clearly communicate the intent and behavior of your code. This article delves deep into the nuances of expressing important ideas within your unit tests, focusing on techniques and principles that elevate them from mere code checkers to powerful communication tools.
The Importance of Expressive Unit Tests
Before diving into specific techniques, let's solidify why expressive unit tests are paramount:
-
Improved Code Understanding: Well-written unit tests serve as living documentation. They articulate how different parts of your code are intended to function, making it easier for developers (including your future self) to understand the codebase. This is especially valuable in larger projects or when team members change.
-
Reduced Debugging Time: Clear, concise tests pinpoint the exact location and nature of bugs. When a test fails, the failure message should immediately reveal the problem, saving hours of debugging.
-
Enhanced Maintainability: Expressive tests simplify the process of modifying existing code. Because the tests clearly define expected behavior, developers can confidently refactor or add new features, knowing the tests will catch any unintended consequences.
-
Improved Collaboration: When tests are easily understandable, collaboration becomes more efficient. Team members can quickly grasp the functionality of different modules and contribute effectively.
-
Increased Confidence: Comprehensive and expressive unit tests instill confidence in the quality and stability of the software. Developers can deploy changes with greater assurance, knowing that a thorough testing suite protects against regressions.
Key Principles for Expressive Unit Tests
Several guiding principles underpin the creation of highly expressive unit tests:
-
Clarity over Conciseness: While brevity is desirable, prioritize clarity. A slightly longer test that's easily understandable is far superior to a cryptic, concise test that's difficult to decipher.
-
Descriptive Naming: Test names should clearly convey the tested functionality. Avoid generic names like
test_something()
ortest_function()
. Instead, use descriptive names such astest_calculate_total_price_with_discount()
ortest_user_cannot_login_with_incorrect_password()
. -
Single Assertion per Test: Each test should ideally focus on verifying a single aspect of the code's behavior. This makes it easier to pinpoint the cause of failure. If multiple assertions are required, consider breaking down the test into smaller, more focused tests.
-
Arrange-Act-Assert (AAA) Pattern: This pattern provides a structured approach to writing unit tests.
- Arrange: Set up the necessary preconditions and inputs for the test.
- Act: Execute the code under test.
- Assert: Verify the expected outcomes.
-
Meaningful Assertions: Use assertion messages that clearly explain the expected outcome and the actual result when an assertion fails. This helps developers quickly diagnose the problem.
Techniques for Enhancing Expressiveness
Let's explore practical techniques to make your unit tests more expressive:
-
Employing Test-Driven Development (TDD): TDD promotes writing tests before writing the code. This approach forces you to think carefully about the desired functionality and ensures your tests are well-aligned with the code's intended behavior.
-
Using Mocks and Stubs: These techniques are particularly valuable when dealing with dependencies. Mocks allow you to simulate the behavior of external components, making your tests more isolated and easier to understand. Stubs provide simplified implementations of dependencies, preventing them from interfering with the test's main focus.
-
Leveraging Test Frameworks: Modern testing frameworks offer features that enhance test readability and maintainability. These frameworks often include features for organizing tests, running tests, and generating reports.
Example: Illustrating Expressive Testing
Let's consider a simple Python function that calculates the area of a rectangle:
def calculate_rectangle_area(length, width):
"""Calculates the area of a rectangle."""
if length <= 0 or width <= 0:
raise ValueError("Length and width must be positive values.")
return length * width
A poorly written unit test might look like this:
import unittest
class TestRectangleArea(unittest.TestCase):
def test_area(self):
self.assertEqual(calculate_rectangle_area(5, 10), 50)
self.assertEqual(calculate_rectangle_area(2, 3), 6)
This test lacks expressiveness. A more expressive version would be:
import unittest
class TestRectangleArea(unittest.TestCase):
def test_calculates_area_correctly_for_positive_values(self):
length = 5
width = 10
expected_area = 50
actual_area = calculate_rectangle_area(length, width)
self.assertEqual(actual_area, expected_area, f"Expected area: {expected_area}, Actual area: {actual_area}")
def test_calculates_area_correctly_for_small_positive_values(self):
length = 2
width = 3
expected_area = 6
actual_area = calculate_rectangle_area(length,width)
self.assertEqual(actual_area, expected_area, f"Expected area: {expected_area}, Actual area: {actual_area}")
def test_raises_value_error_for_zero_length(self):
with self.assertRaises(ValueError) as context:
calculate_rectangle_area(0, 5)
self.assertEqual(str(context.exception), "Length and width must be positive values.")
def test_raises_value_error_for_zero_width(self):
with self.assertRaises(ValueError) as context:
calculate_rectangle_area(5, 0)
self.assertEqual(str(context.exception), "Length and width must be positive values.")
def test_raises_value_error_for_negative_length(self):
with self.assertRaises(ValueError) as context:
calculate_rectangle_area(-5, 5)
self.assertEqual(str(context.exception), "Length and width must be positive values.")
def test_raises_value_error_for_negative_width(self):
with self.assertRaises(ValueError) as context:
calculate_rectangle_area(5, -5)
self.assertEqual(str(context.exception), "Length and width must be positive values.")
This improved version uses descriptive test names, follows the AAA pattern, and includes informative assertion messages. The addition of tests for edge cases (zero and negative values) enhances the test suite's completeness and robustness.
Advanced Techniques for Expressive Unit Tests
Beyond the basics, several advanced techniques further elevate expressiveness:
-
Property-Based Testing: This approach generates a large number of test cases automatically, based on defined properties of the function under test. This helps uncover edge cases and unexpected behavior that might be missed with manually written tests. Libraries like Hypothesis (Python) facilitate property-based testing.
-
Data-Driven Testing: This technique allows you to execute the same test with different input data sets. This is particularly useful when testing functions with various input combinations. Frameworks often provide mechanisms for parameterizing tests.
-
Using a Consistent Style Guide: Adhering to a consistent coding style guide for your tests improves readability and maintainability. Consistency in naming conventions, formatting, and commenting enhances the overall clarity of your test suite.
Conclusion: The Power of Expressive Unit Tests
Expressive unit tests are not simply a matter of achieving high test coverage; they are a crucial component of building high-quality software. By applying the principles and techniques discussed in this article, you can transform your unit tests from simple code verifiers into powerful communication tools that enhance code understanding, simplify debugging, and foster collaboration. The investment in crafting expressive unit tests pays dividends in terms of improved software quality, reduced maintenance costs, and increased developer productivity. Remember that well-written unit tests are not just for the machines; they're for people, including your future self. Prioritizing clarity, using descriptive names, and structuring your tests logically will ultimately lead to a more robust and maintainable codebase.
Latest Posts
Latest Posts
-
What Is Ricks Body Mass Index Or Bmi Range
Apr 02, 2025
-
Juan Arrives At The Clinic 40 Minutes
Apr 02, 2025
-
Activity 3 1a Linear Measurement With Metric Units
Apr 02, 2025
-
For Each Scenario Calculate The Income Elasticity Of Demand
Apr 02, 2025
-
What Do Internship Programs Typically Accomplish
Apr 02, 2025
Related Post
Thank you for visiting our website which covers about 3.13 Unit Test How Important Ideas Are Expressed . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.