5.20 Unit Test Line And Triangle Relationships Part 1
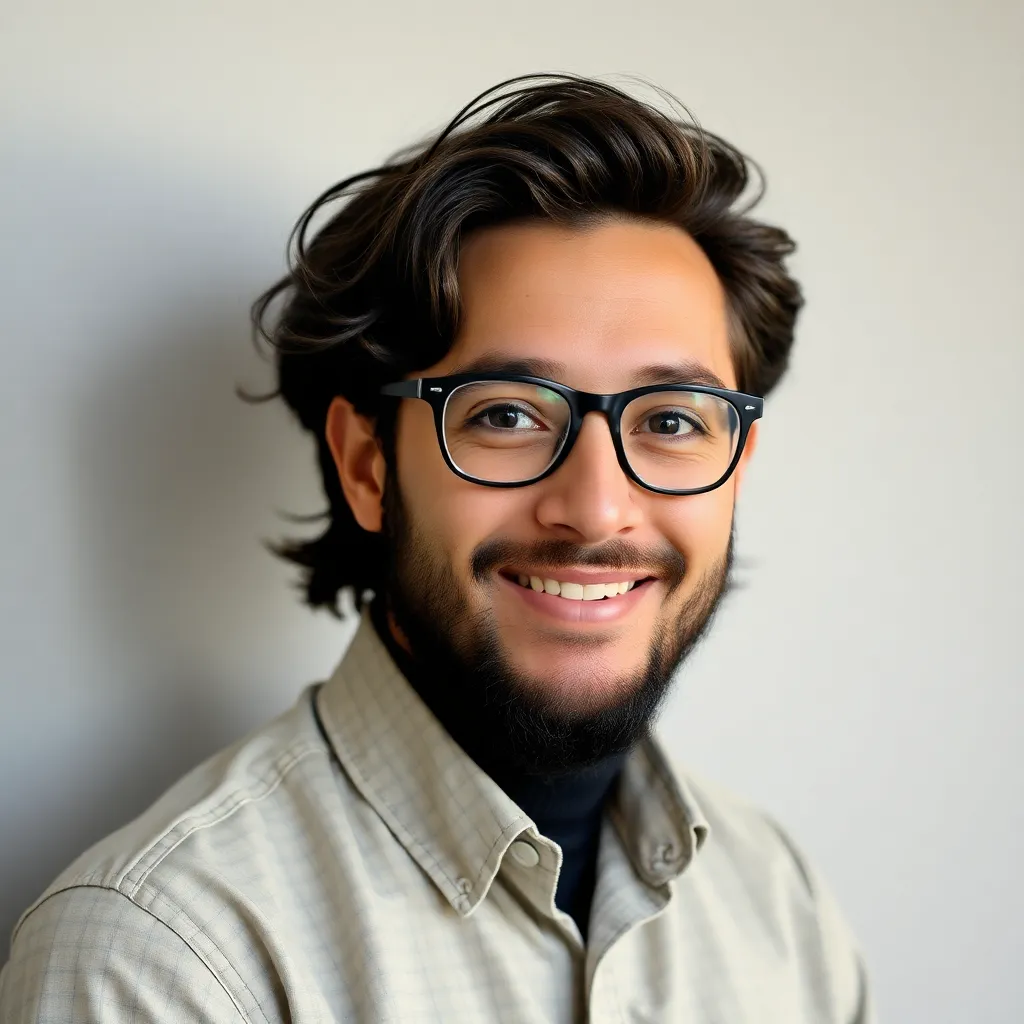
Onlines
Mar 20, 2025 · 5 min read
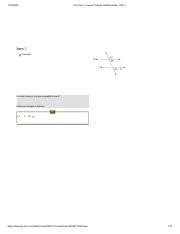
Table of Contents
5.20 Unit Test: Line and Triangle Relationships - Part 1
This article delves into the intricacies of unit testing geometric relationships, specifically focusing on lines and triangles. We'll explore various scenarios, code examples (in Python), and best practices for robust and comprehensive unit tests. This is Part 1, concentrating on foundational concepts and testing line-related properties. Part 2 will expand on triangle relationships.
Understanding the Need for Unit Testing in Geometry
Geometry, while seemingly straightforward, can be surprisingly complex when dealing with edge cases and nuanced relationships between shapes. Unit testing provides a rigorous framework to ensure the accuracy and reliability of your geometric algorithms. By isolating individual components (units) of your code, you can identify and resolve bugs early in the development process, preventing larger, more difficult-to-debug issues later.
Benefits of Unit Testing Geometric Algorithms:
- Early Bug Detection: Identifying and fixing bugs in isolated units is significantly easier than tracking down errors in complex systems.
- Improved Code Quality: The process of writing unit tests encourages more modular, well-structured, and maintainable code.
- Enhanced Confidence: Comprehensive unit tests provide assurance that your geometric algorithms function correctly across a wide range of inputs.
- Regression Prevention: As you modify your code, unit tests help prevent regressions—introducing bugs inadvertently when making changes.
- Easier Debugging: When errors occur, unit tests provide a clear and concise way to pinpoint the source of the problem.
Defining Line Properties and Relationships
Before diving into the unit tests, let's define some fundamental line properties and relationships that we'll be testing:
1. Point Representation: We'll represent points in 2D space using tuples (x, y).
2. Line Representation: We'll represent a line using two distinct points: line = (point1, point2)
.
3. Line Length: The distance between two points (x1, y1) and (x2, y2) is calculated using the distance formula: sqrt((x2 - x1)^2 + (y2 - y1)^2)
.
4. Slope: The slope (m) of a line passing through points (x1, y1) and (x2, y2) is calculated as: m = (y2 - y1) / (x2 - x1)
. Note that a vertical line has an undefined slope.
5. Parallel Lines: Two lines are parallel if their slopes are equal.
6. Perpendicular Lines: Two lines are perpendicular if the product of their slopes is -1. A horizontal and vertical line are also considered perpendicular.
7. Collinear Points: Three or more points are collinear if they lie on the same line. This can be determined by checking if the slope between any pair of points is consistent.
Python Code Implementation and Unit Tests (Part 1: Lines)
Let's implement these concepts in Python and write unit tests using the unittest
module.
import unittest
import math
def calculate_line_length(line):
"""Calculates the length of a line."""
point1, point2 = line
return math.sqrt((point2[0] - point1[0])**2 + (point2[1] - point1[1])**2)
def calculate_slope(line):
"""Calculates the slope of a line."""
point1, point2 = line
if point2[0] - point1[0] == 0:
return float('inf') #Representing undefined slope for vertical lines.
return (point2[1] - point1[1]) / (point2[0] - point1[0])
def are_lines_parallel(line1, line2):
"""Checks if two lines are parallel."""
slope1 = calculate_slope(line1)
slope2 = calculate_slope(line2)
return slope1 == slope2
def are_lines_perpendicular(line1, line2):
"""Checks if two lines are perpendicular."""
slope1 = calculate_slope(line1)
slope2 = calculate_slope(line2)
if math.isinf(slope1) or math.isinf(slope2):
return slope1 != slope2 #One is vertical, the other horizontal
return slope1 * slope2 == -1
class TestLineRelationships(unittest.TestCase):
def test_line_length(self):
self.assertEqual(calculate_line_length(((0, 0), (3, 4))), 5)
self.assertEqual(calculate_line_length(((1, 1), (1, 5))), 4)
self.assertEqual(calculate_line_length(((0,0),(0,0))), 0) #Testing Zero Length Line
def test_calculate_slope(self):
self.assertEqual(calculate_slope(((0, 0), (1, 1))), 1)
self.assertEqual(calculate_slope(((0, 0), (1, 0))), 0)
self.assertEqual(calculate_slope(((0,0),(0,1))), float('inf')) #Vertical line
self.assertAlmostEqual(calculate_slope(((1,2),(3,4))), 1.0) #Handles floats
def test_parallel_lines(self):
self.assertTrue(are_lines_parallel(((0, 0), (1, 1)), ((1, 1), (2, 2))))
self.assertFalse(are_lines_parallel(((0, 0), (1, 1)), ((0, 0), (1, 2))))
self.assertTrue(are_lines_parallel(((0,0),(0,1)),((1,0),(1,1)))) #Parallel vertical lines
def test_perpendicular_lines(self):
self.assertTrue(are_lines_perpendicular(((0, 0), (1, 1)), ((0, 0), (1, -1))))
self.assertTrue(are_lines_perpendicular(((0,0),(0,1)),((0,0),(1,0)))) #Vertical and horizontal lines
self.assertFalse(are_lines_perpendicular(((0, 0), (1, 1)), ((0, 0), (2, 2))))
if __name__ == '__main__':
unittest.main()
This code provides a basic framework. Further enhancements could include:
- Error Handling: Adding more robust error handling for invalid inputs (e.g., non-tuple points, identical points defining a line).
- More Comprehensive Tests: Adding more test cases to cover a wider range of inputs, including edge cases and boundary conditions (e.g., lines with very small or very large slopes, near-collinear points).
- Different Line Representations: Exploring other ways to represent lines (e.g., using slope-intercept form: y = mx + b) and testing the corresponding algorithms.
- Testing for Collinearity: Implementing a function to check collinearity and adding corresponding unit tests.
Advanced Concepts and Future Extensions (Part 2 Preview)
Part 2 will build upon this foundation, extending the unit tests to include triangle relationships:
- Triangle Representation: Defining how triangles will be represented (e.g., using three points).
- Triangle Area Calculation: Testing algorithms for calculating the area of a triangle (e.g., using Heron's formula or the determinant method).
- Triangle Classification: Testing algorithms for classifying triangles based on their side lengths (equilateral, isosceles, scalene) and angles (acute, obtuse, right-angled).
- Triangle Properties: Testing relationships such as the Pythagorean theorem for right-angled triangles and other geometric properties.
- Intersection of Lines and Triangles: Investigating algorithms to detect whether lines intersect triangles and testing these algorithms comprehensively.
By incrementally building upon the foundation laid in Part 1, we can create a comprehensive suite of unit tests that ensure the correctness and reliability of our geometric algorithms. Remember that thorough testing is crucial for producing high-quality, robust, and dependable geometric software. The focus on edge cases and boundary conditions will be paramount in ensuring that the software performs as expected across various scenarios. This rigorous approach minimizes bugs and enhances the overall quality and reliability of the application.
Latest Posts
Latest Posts
-
The Love Suicides At Amijima Summary
Mar 20, 2025
-
Tends To Supervise Employees Very Closely
Mar 20, 2025
-
You Arrive On The Scene With The Code Team
Mar 20, 2025
-
When Supplies Are Purchased On Credit It Means That
Mar 20, 2025
-
Choose The Letter Of The Best Answer
Mar 20, 2025
Related Post
Thank you for visiting our website which covers about 5.20 Unit Test Line And Triangle Relationships Part 1 . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.