6.2.9 Find Index Of A String
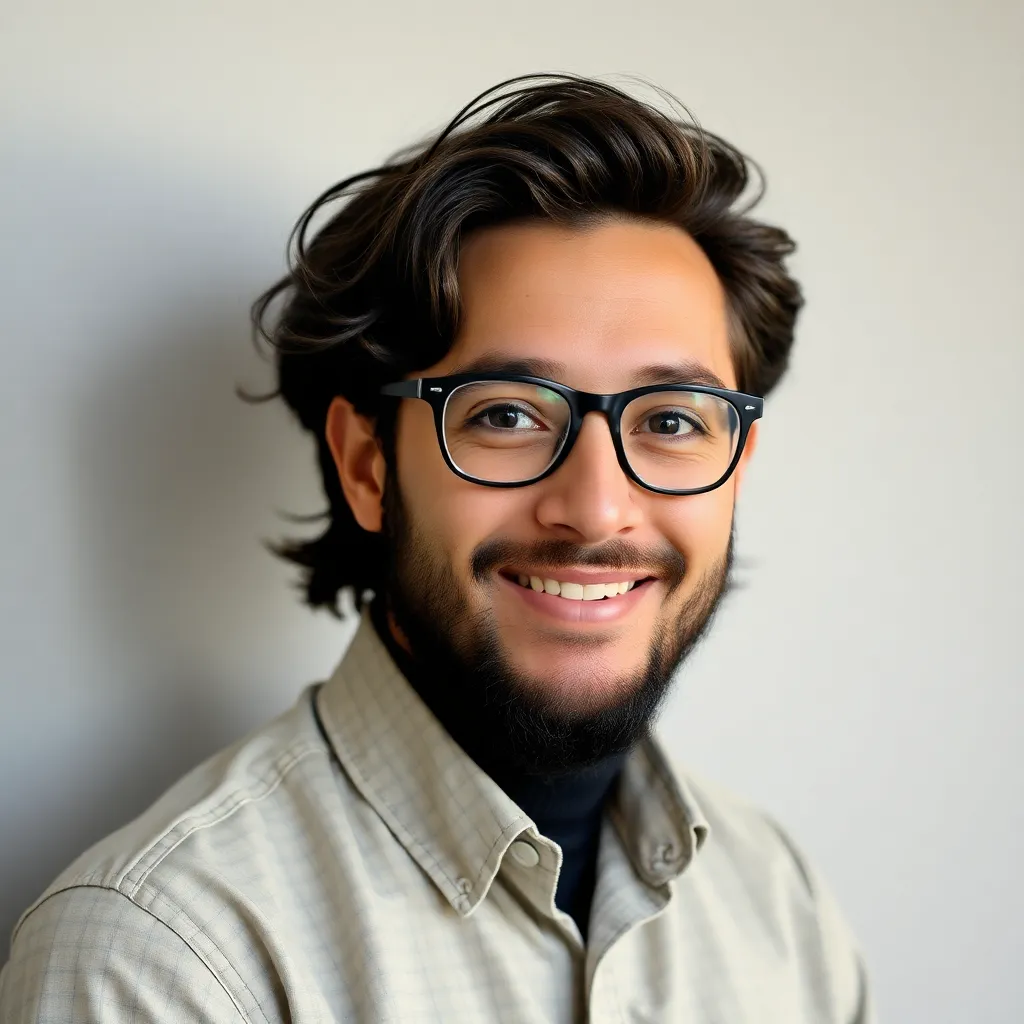
Onlines
Mar 14, 2025 · 6 min read
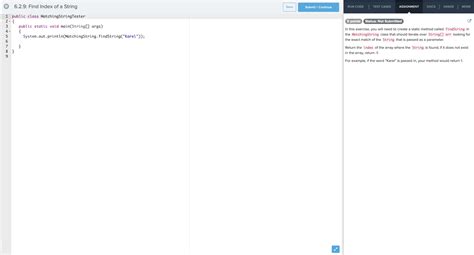
Table of Contents
6.2.9: Mastering String Index Finding Techniques
Finding the index of a substring within a larger string is a fundamental task in many programming scenarios. Whether you're parsing text files, searching for patterns in data, or manipulating strings in a web application, understanding how to efficiently locate substrings is crucial. This comprehensive guide delves into the intricacies of finding string indices, covering various techniques, their complexities, and best practices for optimal performance. We'll explore different approaches, analyze their strengths and weaknesses, and provide practical examples to solidify your understanding.
Understanding String Indices
Before diving into specific techniques, let's establish a clear understanding of string indices. A string is essentially an ordered sequence of characters. Each character occupies a specific position within the string, and this position is represented by its index. Indices typically start at 0 for the first character, 1 for the second, and so on. For example:
string = "Hello, World!"
string[0]
would return "H"string[7]
would return "W"string[12]
would return "!"
Finding the index of a substring means determining the starting index of the first occurrence of that substring within the main string.
Method 1: Using the index()
Method (Python)
Python offers a built-in index()
method that simplifies the process of finding a substring's index. This method is straightforward and efficient for many common use cases.
Syntax:
string.index(substring, start, end)
string
: The main string where you're searching.substring
: The substring you want to find the index of.start
(optional): The starting index of the search.end
(optional): The ending index of the search.
Example:
text = "This is a sample string."
index = text.index("sample")
print(index) # Output: 10
This code snippet finds the index of the substring "sample" within the string "This is a sample string." The output is 10 because "sample" starts at the 10th index (remembering that indexing starts at 0).
Handling Cases Where the Substring is Not Found:
The index()
method raises a ValueError
if the substring is not found. To handle this gracefully, you can use a try-except
block:
text = "This is a sample string."
try:
index = text.index("missing")
print(index)
except ValueError:
print("Substring not found!")
Method 2: Using the find()
Method (Python)
Similar to index()
, the find()
method locates a substring within a string. However, instead of raising a ValueError
when the substring is not found, find()
returns -1.
Syntax:
string.find(substring, start, end)
- Identical parameters to
index()
.
Example:
text = "This is a sample string."
index = text.find("sample")
print(index) # Output: 10
index = text.find("missing")
print(index) # Output: -1
The find()
method is often preferred when you need to check for the presence of a substring without explicitly handling exceptions. It's more concise and can be slightly faster due to the absence of exception handling overhead.
Method 3: Manual Iteration (For Understanding and Customization)
Understanding the underlying logic is crucial. A manual iteration approach can be helpful in learning the core concepts and allows for greater flexibility in handling specific scenarios.
Example (Python):
def find_substring_index(text, substring):
for i in range(len(text) - len(substring) + 1):
if text[i:i + len(substring)] == substring:
return i
return -1
text = "This is a sample string."
index = find_substring_index(text, "sample")
print(index) # Output: 10
This function iterates through the main string, comparing substrings of the same length as the target substring. It returns the index if a match is found; otherwise, it returns -1. This approach is less efficient than built-in methods for large strings but offers greater control and insight into the process.
Method 4: Regular Expressions (For Complex Pattern Matching)
Regular expressions provide a powerful tool for pattern matching within strings. They allow you to define complex search patterns beyond simple substring searches.
Example (Python):
import re
text = "This is a sample string with multiple 123 numbers."
match = re.search(r"\b\d+\b", text) # searches for whole numbers
if match:
print(match.start()) #prints starting index of the first match
else:
print("No match found")
This uses re.search
to find the first occurrence of one or more digits (\d+
) that are whole words (\b
). The match.start()
method provides the starting index of the matched pattern. Regular expressions offer flexibility for intricate pattern recognition but come with a steeper learning curve.
Method 5: Optimized Search Algorithms (For Extremely Large Strings)
For exceptionally large strings, the efficiency of the search algorithm becomes paramount. Simple linear search approaches (like manual iteration) can become prohibitively slow. Algorithms like the Boyer-Moore algorithm and the Knuth-Morris-Pratt (KMP) algorithm offer significant performance improvements for large-scale string searches. These algorithms are more complex to implement but provide substantially faster results, especially when dealing with repetitive patterns or very long strings. While the details of these algorithms are beyond the scope of this basic introduction, understanding their existence is important for optimizing string search operations in performance-critical applications.
Choosing the Right Method
The optimal method for finding a string's index depends on the specific context:
- Simple substring searches: The built-in
index()
orfind()
methods are efficient and easy to use.find()
is generally preferred for its exception handling. - Substring absence check:
find()
's -1 return value makes it ideal for easily checking if a substring exists. - Learning or customization: Manual iteration provides a deeper understanding of the underlying process and allows tailored handling of specific situations.
- Complex pattern matching: Regular expressions provide flexibility for intricate search patterns.
- Extremely large strings: Optimized algorithms like Boyer-Moore or KMP provide significant performance improvements.
Error Handling and Best Practices
- Always handle potential errors: Use
try-except
blocks with theindex()
method or check for a return value of -1 withfind()
to gracefully handle cases where the substring is not found. - Consider case sensitivity: If case sensitivity is not desired, convert the string and substring to lowercase or uppercase before searching.
- Optimize for performance: For very large strings or frequent searches, consider using optimized algorithms (Boyer-Moore, KMP).
- Use appropriate data structures: If you need to perform multiple searches on the same string, consider preprocessing the string into a more efficient data structure (e.g., a suffix tree or trie) for faster lookups.
Conclusion
Finding the index of a substring within a string is a core string manipulation task. Choosing the right approach – whether it's using built-in methods, manual iteration, regular expressions, or optimized algorithms – depends heavily on the specific needs of your application. Understanding the trade-offs between simplicity, efficiency, and flexibility allows you to select the most appropriate technique for optimal performance and code clarity. By mastering these methods and incorporating best practices, you can confidently navigate the world of string manipulation and build robust and efficient applications.
Latest Posts
Latest Posts
-
Filing Your Taxes Chapter 10 Lesson 4 Answer Key
Mar 14, 2025
-
You Market Many Different Types Of Insurance
Mar 14, 2025
-
Black Ties And White Lies Pdf
Mar 14, 2025
-
Scot Fitzwilliam Is A Medicare Beneficiary
Mar 14, 2025
-
Who Is Authorized To Modify Linux
Mar 14, 2025
Related Post
Thank you for visiting our website which covers about 6.2.9 Find Index Of A String . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.