Live Virtual Machine Lab 21-2: Basic Scripting Techniques
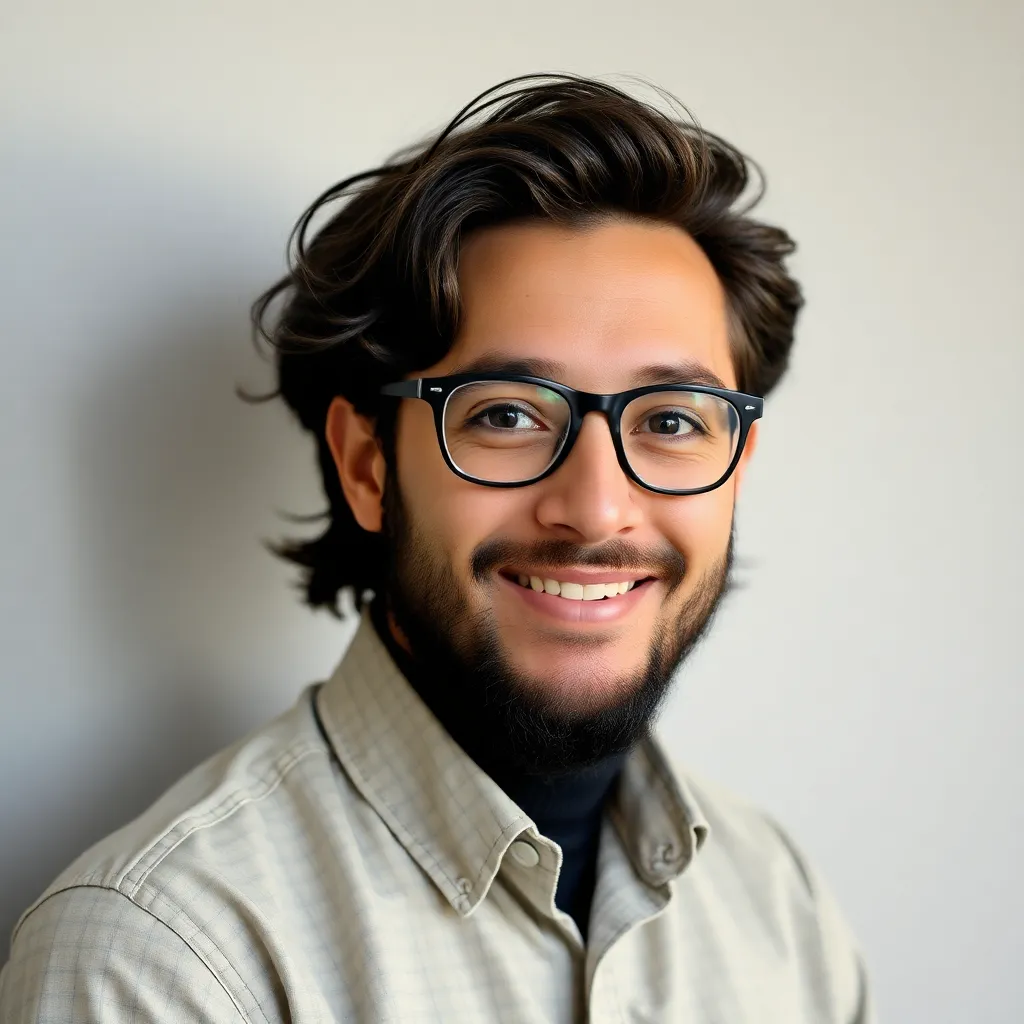
Onlines
Mar 26, 2025 · 6 min read
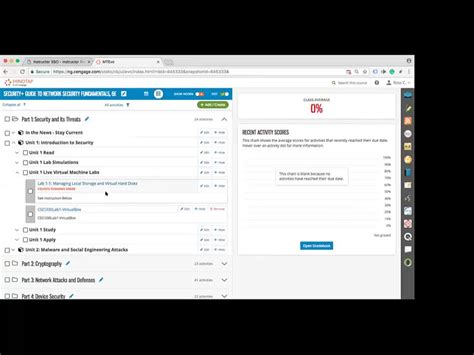
Table of Contents
Live Virtual Machine Lab 21-2: Basic Scripting Techniques
This comprehensive guide dives deep into the world of basic scripting techniques, utilizing a live virtual machine (VM) environment for hands-on learning. We'll cover fundamental concepts, practical examples, and best practices to equip you with the skills to automate tasks and enhance your system administration capabilities. This lab focuses on building a strong foundation, preparing you for more advanced scripting endeavors.
What is Scripting?
Scripting is the process of writing a sequence of commands or instructions that a computer can execute. Unlike compiled languages like C++ or Java, scripts are typically interpreted line by line by an interpreter, making them easier to write, debug, and modify. This flexibility is invaluable for automating repetitive tasks, managing system configurations, and streamlining workflows.
Key advantages of scripting include:
- Automation: Automate tedious or repetitive tasks, saving time and reducing errors.
- Efficiency: Streamline complex procedures into concise, reusable scripts.
- Flexibility: Adapt scripts to changing requirements with relative ease.
- Repeatability: Ensure consistent results by executing the same script multiple times.
- Maintainability: Easier to understand and modify compared to compiled programs.
Setting up Your Live Virtual Machine Environment
Before diving into scripting, ensure your live VM environment is ready. This typically involves:
- Choosing an appropriate operating system: Popular choices include Linux distributions (like Ubuntu or CentOS) and Windows Server. Linux is often preferred for its powerful command-line interface and extensive scripting capabilities.
- Sufficient resources: Allocate enough RAM and disk space to your VM to avoid performance bottlenecks. The exact requirements will vary depending on your chosen OS and the complexity of the scripts you'll be running.
- Network connectivity: Ensure your VM has network access to download necessary packages and resources.
- Text editor: A robust text editor is crucial. Popular choices include Vim, Nano (Linux), and Notepad++ (Windows).
Introduction to Shell Scripting (Bash)
Bash (Bourne Again Shell) is a widely-used command-line interpreter on Linux and macOS systems. It's a powerful tool for writing shell scripts, which are essentially sequences of Bash commands saved in a file. Let's explore some fundamental concepts:
Shebang
The first line of a Bash script should always be the shebang, indicating the interpreter to use:
#!/bin/bash
This tells the system to execute the script using the Bash interpreter located at /bin/bash
.
Variables
Variables store data that your script can use. They are declared without a specific data type and are assigned values using the =
operator:
name="John Doe"
age=30
To access the value of a variable, precede its name with a dollar sign ($
):
echo "My name is $name and I am $age years old."
Comments
Comments are essential for documenting your code. In Bash, comments begin with a #
symbol:
# This is a comment
name="Jane Doe" # This is an inline comment
Basic Commands
Bash scripts often involve common commands like:
echo
: Displays text to the console.date
: Displays the current date and time.pwd
: Prints the current working directory.ls
: Lists files and directories.cd
: Changes the current directory.mkdir
: Creates a new directory.rm
: Removes files or directories.cp
: Copies files or directories.mv
: Moves or renames files or directories.
Control Flow
Control flow statements dictate the order in which commands are executed.
if
statement: Executes a block of code only if a condition is true:
if [ $age -ge 18 ]; then
echo "You are an adult."
fi
if-else
statement: Executes one block of code if a condition is true, and another if it's false:
if [ $age -ge 18 ]; then
echo "You are an adult."
else
echo "You are a minor."
fi
for
loop: Iterates over a sequence of values:
for i in {1..5}; do
echo "Iteration: $i"
done
while
loop: Repeats a block of code as long as a condition is true:
count=0
while [ $count -lt 5 ]; do
echo "Count: $count"
count=$((count + 1))
done
Functions
Functions encapsulate reusable blocks of code:
greet() {
echo "Hello, $1!"
}
greet "World"
Input/Output Redirection
Redirect the output of a command to a file using >
:
ls > file_list.txt
Append output to a file using >>
:
date >> file_list.txt
Read input from a file using <
:
cat < input.txt
Advanced Scripting Techniques
Beyond the basics, let's explore some more advanced techniques:
Arrays
Arrays store collections of values:
names=("Alice" "Bob" "Charlie")
echo "${names[0]}" # Accessing the first element
Case Statements
Handle multiple conditions elegantly:
case "$option" in
"start")
echo "Starting..."
;;
"stop")
echo "Stopping..."
;;
*)
echo "Invalid option."
;;
esac
Looping through files and directories
Utilize find
and for
loops for powerful file manipulation:
find . -name "*.txt" -print0 | while IFS= read -r -d
Latest Posts
Latest Posts
-
Reinforcement For Emitting A Correct Echoic Is Usually
Mar 29, 2025
-
C Is Trying To Determine Whether To Convert
Mar 29, 2025
-
A Female Infant Is In For A Feeding Consultant
Mar 29, 2025
-
Nutrient Cycling In The Serengeti Answer Key
Mar 29, 2025
-
The Client Record Houses The Following Information Except
Mar 29, 2025
Related Post
Thank you for visiting our website which covers about Live Virtual Machine Lab 21-2: Basic Scripting Techniques . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.